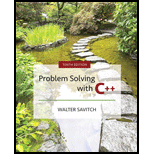
Problem Solving with C++ (10th Edition)
10th Edition
ISBN: 9780134448282
Author: Walter Savitch, Kenrick Mock
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 16, Problem 7PP
Program Plan Intro
- Include required library files.
- Define a class named “ArrOutOfRangeErr”.
- Declare the required variables, constructors and function inside the access specifier “public” and “private”.
- Define a “testExcep()” function to test the exception.
- Using exception, set and get the values.
- Define a “create2DArr()” function to create an two-dimensional array.
- Define a “check_args()” function to check the validity and return the message if something is wrong.
- Define a “set()” function to store values.
- Define a “get()” function to retrieve the values.
- Define a default constructor for no messages.
- Define a parameterized constructor for row and column data.
- Define a “getMsg()” function to return the exception message string.
- Define a “getRows()” function to return the number of rows.
- Define a “getCols()” function to return the number of columns.
- Define a “getDesiredRow()” function to return the requested row number.
- Define a “getDesiredColumn()” function to return the requested column number.
- Define a “writeErr()” function to write the error message.
- Define a “main()” function.
- Declare the required variables.
- Create a 2D array for rows and columns.
- Populate the rows and columns.
- Print the array contents using “for” loop.
- Then test the some boundary cases.
- Using exception, set and get the values.
- Declare the required variables.
- Create a 2D array for rows and columns.
- Populate the rows and columns.
- Print the array contents using “for” loop.
- Then test the some boundary cases.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
This is a question that I have and would like someone who has experiences with scene graphs and entity component systems to answer.For context, I am currently implementing a game engine and currently I am debating on our current design.Our current design is we have a singular game component class that every component inherits from. Where we have components like SpriteRendererComponent, Mehs Component, etc. They inherit from this GameComponent class. The point of this is being able to have O(1) access to the scene to being able to modify components to attach more components with the idea of accessing those components to specific scene objects in a scene.Now, my question is what kinds of caveauts can this cause in terms of cache coherence? I am well aware that yes its O(1) and that is great but cache coherence is going to be really bad, but would like to know more explicit details and real-life examples such as write in RAM examples on how this is bad. A follow-up question that is part…
Q4: Consider the following MAILORDER relational schema describing the data for a mail order
company. (Choose five only).
PARTS(Pno, Pname, Qoh, Price, Olevel)
CUSTOMERS(Cno, Cname, Street, Zip, Phone)
EMPLOYEES(Eno, Ename, Zip, Hdate)
ZIP CODES(Zip, City)
ORDERS(Ono, Cno, Eno, Received, Shipped)
ODETAILS(Ono, Pno, Qty)
(10 Marks)
I want a detailed explanation to
understand the mechanism how it is
Qoh stands for quantity on hand: the other attribute names are self-explanatory. Specify and
execute the following queries using the RA interpreter on the MAILORDER database schema.
a. Retrieve the names of parts that cost less than $20.00.
b. Retrieve the names and cities of employees who have taken orders for parts costing more than
$50.00.
c. Retrieve the pairs of customer number values of customers who live in the same ZIP Code.
d. Retrieve the names of customers who have ordered parts from employees living in Wichita.
e. Retrieve the names of customers who have ordered parts costing less…
Q4: Consider the following MAILORDER relational schema describing the data for a mail order
company. (Choose five only).
(10 Marks)
PARTS(Pno, Pname, Qoh, Price, Olevel)
CUSTOMERS(Cno, Cname, Street, Zip, Phone)
EMPLOYEES(Eno, Ename, Zip, Hdate)
ZIP CODES(Zip, City)
ORDERS(Ono, Cno, Eno, Received, Shipped)
ODETAILS(Ono, Pno, Qty)
Qoh stands for quantity on hand: the other attribute names are self-explanatory. Specify and
execute the following queries using the RA interpreter on the MAILORDER database schema.
a. Retrieve the names of parts that cost less than $20.00.
b. Retrieve the names and cities of employees who have taken orders for parts costing more than
$50.00.
c. Retrieve the pairs of customer number values of customers who live in the same ZIP Code.
d. Retrieve the names of customers who have ordered parts from employees living in Wichita.
e. Retrieve the names of customers who have ordered parts costing less than$20.00.
f. Retrieve the names of customers who have not placed…
Chapter 16 Solutions
Problem Solving with C++ (10th Edition)
Ch. 16.1 - Prob. 1STECh. 16.1 - What would be the output produced by the code in...Ch. 16.1 - Prob. 3STECh. 16.1 - What happens when a throw statement is executed?...Ch. 16.1 - In the code given in Self-Test Exercise 1, what is...Ch. 16.1 - Prob. 6STECh. 16.1 - Prob. 7STECh. 16.1 - What is the output produced by the following...Ch. 16.1 - What is the output produced by the program in...Ch. 16.2 - Prob. 10STE
Knowledge Booster
Similar questions
- ut da Q4: Consider the LIBRARY relational database schema shown in Figure below a. Edit Author_name to new variable name where previous surname was 'Al - Wazny'. Update book_Authors set Author_name = 'alsaadi' where Author_name = 'Al - Wazny' b. Change data type of Phone to string instead of numbers. عرفنه شنو الحل BOOK Book id Title Publisher_name BOOK AUTHORS Book id Author_name PUBLISHER Name Address Phone e u al b are rage c. Add two Publishers Company existed in UK. insert into publisher(name, address, phone) value('ali','uk',78547889), ('karrar', 'uk', 78547889) d. Remove all books author when author name contains second character 'D' and ending by character 'i'. es inf rmar nce 1 tic عرفته شنو الحل e. Add one book as variables data? عرفته شنو الحلarrow_forwardAdd a timer in the following code. public class GameGUI extends JPanel { private final Labyrinth labyrinth; private final Player player; private final Dragon dragon; private Timer timer; private long elapsedTime; public GameGUI(Labyrinth labyrinth, Player player, Dragon dragon) { this.labyrinth = labyrinth; this.player = player; this.dragon = dragon; String playerName = JOptionPane.showInputDialog("Enter your name:"); player.setName(playerName); elapsedTime = 0; timer = new Timer(1000, e -> { elapsedTime++; repaint(); }); timer.start(); } @Override protected void paintComponent(Graphics g) { super.paintComponent(g); int cellSize = Math.min(getWidth() / labyrinth.getSize(), getHeight() / labyrinth.getSize());}arrow_forwardChange the following code so that when player wins the game, the game continues by creating new GameGUI with the same player. However the player's starting position is same, everything else should be reseted. public static void main(String[] args) { Labyrinth labyrinth = new Labyrinth(10); Player player = new Player(9, 0); Random rand = new Random(); Dragon dragon = new Dragon(rand.nextInt(10), 9); JFrame frame = new JFrame("Labyrinth Game"); GameGUI gui = new GameGUI(labyrinth, player, dragon); frame.setLayout(new BorderLayout()); frame.setSize(600, 600); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.add(gui, BorderLayout.CENTER); frame.pack(); frame.setResizable(false); frame.setVisible(true); } public class GameGUI extends JPanel { private final Labyrinth labyrinth; private final Player player; private final Dragon dragon; private Timer timer; private long…arrow_forward
- Create a menu item which restarts the game. Also add a timer, which counts the elapsed time since the start of the game level. When the restart is pressed, the restarted game should ask the player' name (in the GameGUI constructor) and set the score of player to 0 (player.setScore(0)), and the timer should restart again. And create a logic so that if the player loses his life (checkGame if the condition is false), then save this number together with his name into two variables. And display two buttons where one quits the game altogether (System.exit(0)) and the other restarts the game. public class GameGUI extends JPanel { private final Labyrinth labyrinth; private final Player player; private final Dragon dragon; private final ImageIcon playerIcon = new ImageIcon("data/images/player.png"); private final ImageIcon dragonIcon = new ImageIcon("data/images/dragon.png"); private final ImageIcon wallIcon = new ImageIcon("data/images/wall.png"); private final ImageIcon…arrow_forwardPlease original work Analyze the complexity issues of processing big data What are five complexities and talk about the reasons they make the implementation complex. Please cite in text references and add weblinksarrow_forwardCreate a Database in JAVA Netbeans that saves name of the player and how many labyrinths did the player solve. Record the number of how many labyrinths did the player solve, and if he loses his life, then save this number together with his name into the database. Create a menu item, which displays a highscore table of the players for the 10 best scores public class GameGUI extends JPanel { private final Labyrinth labyrinth; private final Player player; private final Dragon dragon; private void checkGameState() { if (player.getX() == 0 && player.getY() == labyrinth.getSize() - 1) { JOptionPane.showMessageDialog(this, "You escaped! Congratulations!"); System.exit(0); } if (Math.abs(player.getX() - dragon.getX()) <= 1 && Math.abs(player.getY() - dragon.getY()) <= 1) { JOptionPane.showMessageDialog(this, "The dragon caught you! Game Over."); System.exit(0); } } } public…arrow_forward
- Create a Database in JAVA OOP that saves name of the player and how many labyrinths did the player solve. Record the number of how many labyrinths did the player solve, and if he loses his life, then save this number together with his name into the database. Create a menu item, which displays a highscore table of the players for the 10 best scores. Also, create a menu item which restarts the game. public class GameGUI extends JPanel { private final Labyrinth labyrinth; private final Player player; private final Dragon dragon; private void checkGameState() { if (player.getX() == 0 && player.getY() == labyrinth.getSize() - 1) { JOptionPane.showMessageDialog(this, "You escaped! Congratulations!"); System.exit(0); } if (Math.abs(player.getX() - dragon.getX()) <= 1 && Math.abs(player.getY() - dragon.getY()) <= 1) { JOptionPane.showMessageDialog(this, "The dragon caught you! Game Over.");…arrow_forwardChange the following code so that the player can see only the neighboring fields at a distance of 3 units. public class GameGUI extends JPanel { private final Labyrinth labyrinth; private final Player player; private final Dragon dragon; private final ImageIcon playerIcon = new ImageIcon("data/images/player.png"); private final ImageIcon dragonIcon = new ImageIcon("data/images/dragon.png"); private final ImageIcon wallIcon = new ImageIcon("data/images/wall.png"); private final ImageIcon emptyIcon = new ImageIcon("data/images/empty.png"); public GameGUI(Labyrinth labyrinth, Player player, Dragon dragon) { this.labyrinth = labyrinth; this.player = player; this.dragon = dragon; setFocusable(true); addKeyListener(new KeyAdapter() { @Override public void keyPressed(KeyEvent e) { char move = switch (e.getKeyCode()) { case KeyEvent.VK_W -> 'W'; case…arrow_forwardQ/ Fill in the table below with the correct answers for the network devices and components required, then draw the topology diagram for the network of this three-story building: I want a drawing Cisco Packet tracer Ground Floor: This floor will house the main server room, reception area, and a few conference rooms. The server room should contain the core network devices that will connect the entire building. The reception area and conference rooms need network access require access for mobile devices for visitors and guests with devices. First Floor: This floor is dedicated to offices with workstations, each needing reliable network connectivity for staff computers, IP phones, IP camera, and printers. Second Floor (Education Department): This floor consists of educational spaces requiring controlled internet access. Only the educational website (https://uokerbala.edu.iq) should be accessible, with all other sites restricted.. Device Media type Location floor Type of IP Static/dynamic…arrow_forward
- Problem Statement You are working as a Devops Administrator. Y ou’ve been t asked to deploy a multi - tier application on Kubernetes Cluster. The application is a NodeJS application available on Docker Hub with the following name: d evopsedu/emp loyee This Node JS application works with a mongo database. MongoDB image is available on D ockerHub with the following name: m ongo You are required to deploy this application on Kubernetes: • NodeJS is available on port 8888 in the container and will be reaching out to por t 27017 for mongo database connection • MongoDB will be accepting connections on port 27017 You must deploy this application using the CL I . Once your application is up and running, ensure you can add an employee from the NodeJS application and verify by going to Get Employee page and retrieving your input. Hint: Name the Mongo DB Service and deployment, specifically as “mongo”.arrow_forwardI need help in server client project. It is around 1200 lines of code in both . I want to meet with the expert online because it is complicated. I want the server send a menu to the client and the client enters his choice and keep on this until the client chooses to exit . the problem is not in the connection itself as far as I know.I tried while loops but did not work. please help its emergentarrow_forwardI need help in my server client in C languagearrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
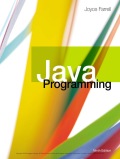
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT