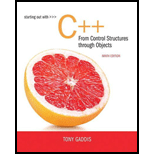
Starting Out with C++ from Control Structures to Objects (9th Edition)
9th Edition
ISBN: 9780134498379
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 16, Problem 24RQE
Program Plan Intro
Exception:
In C++, the exception is an object used to signal the unexpected error occurred during a program execution.
- An exception has been thrown when an error has been occurred in a program.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
PARTICIPATION
ACTIVITY
Arrange the following lines to make a program that determines when the number of people in a restaurant equals or exceeds 10
occupants. The program continually gets the number of people entering or leaving the restaurant. Ex: 2 means two people
entered, and -3 means three people left. After each input, the program outputs the number of people in the restaurant. Once the
number of people in the restaurant equals or exceeds 10, the program exits.
If an Input MismatchException exception occurs, the program should get and discard a single string from input. Ex: The input "2
abc 8" should result in 10 occupants. Not all lines are used in the solution.
How to use this tool ✓
Unused
totalNumPeople += scnr.nextInt ();
while (totalNumPeople < maxNumPeople) {
}
}
11.1.5: Handling input exceptions: restaurant max occupancy tracker.
catch {
try {
catch (InputMismatchException e) {
scnr .nextLine();
scnr.next();
System.out.println("Error");
}
System.out.println("Occupancy:…
def test_func(a,b,c):
return (a+b)/c
This function is normally designed to be used with three numbers: a, b, and c. However, a careless coder may call this function with an ill combination of arguments to cause certain exceptions. Specifically, if any of a, b or c is not a valid number, then this code will produce a TypeError; and if a and b are valid numbers, and c is 0, then the code will produce a ZeroDivisionError.
Your job is to enhance this function by adding proper try...except... blocks, surrounding and capturing the exceptions. When a TypeError occurs, instead of crashing, your code must print on the screen: "Code produced TypeError". And, when a ZeroDivisionError occurs, instead of crashing, your code must print on the screen: "Code produced ZeroDivisionError". In both cases, your function will not crash, will not throw an exception, and silently return None.
POINTERS-DYNAMIC ARRAY- EXCEPTION HANDLING
POINTERS:
GRADE ELIMINATION. A program that will input 10 score for quizzes (0-100) .Get the lowest quiz and eliminate the lowest quiz and compute and output the average of the 9 remaining quizzes. Finally, output only the SUM, LOWEST GRADE and the AVERAGE.
DYNAMIC ARRAY & EXCEPTION HANDLING:
COMPUTE FOR MILES PER GALLON Make a program that will calculate and compute for the quotient of miles and gallons (mpg: miles per gallons). Your program should must ask the user to specify the size of the array (using dynamic array) for the following variable: miles ,gallons and mpg. Prompt the user to Initialize the value of miles (value for miles should be 100-250) and gallons (values should be from 5-25). Use pointer galPtr for gallons, milPtr for miles and mpgPtr for mpg. Use function MilesPerrGallon (double,double) to compute for the values of mpg and use exception handling try-throw-catch to validate the values of miles and gallons.…
Chapter 16 Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Ch. 16.1 - Prob. 16.1CPCh. 16.1 - Prob. 16.2CPCh. 16.1 - Prob. 16.3CPCh. 16.1 - Prob. 16.4CPCh. 16.1 - Prob. 16.5CPCh. 16.3 - Prob. 16.6CPCh. 16.3 - The following function accepts an i nt argument...Ch. 16.3 - Prob. 16.8CPCh. 16.3 - Prob. 16.9CPCh. 16.4 - Prob. 16.10CP
Ch. 16.4 - Prob. 16.11CPCh. 16 - Prob. 1RQECh. 16 - Prob. 2RQECh. 16 - Prob. 3RQECh. 16 - Prob. 4RQECh. 16 - What is unwinding the stack?Ch. 16 - What happens if an exception is thrown by a classs...Ch. 16 - How do you prevent a program from halting when the...Ch. 16 - Why is it more convenient to write a function...Ch. 16 - Why must you be careful when writing a function...Ch. 16 - The line containing a throw statement is known as...Ch. 16 - Prob. 11RQECh. 16 - Prob. 12RQECh. 16 - Prob. 13RQECh. 16 - The beginning of a template is marked by a(n)...Ch. 16 - Prob. 15RQECh. 16 - Prob. 16RQECh. 16 - Write a function that searches a numeric array for...Ch. 16 - Write a function that dynamically allocates a...Ch. 16 - Make the function you wrote in Question 17 a...Ch. 16 - Write a template for a function that displays the...Ch. 16 - Prob. 21RQECh. 16 - Prob. 22RQECh. 16 - Prob. 23RQECh. 16 - Prob. 24RQECh. 16 - T F All type parameters defined in a function...Ch. 16 - Prob. 26RQECh. 16 - T F A class object passed to a function template...Ch. 16 - Prob. 28RQECh. 16 - Prob. 29RQECh. 16 - Prob. 30RQECh. 16 - Prob. 31RQECh. 16 - T F A class template may not be derived from...Ch. 16 - T F A class template may not be used as a base...Ch. 16 - Prob. 34RQECh. 16 - Prob. 35RQECh. 16 - try { quotient = divide(num1, num2); } cout The...Ch. 16 - template class T T square(T number) { return T T;...Ch. 16 - template class T int square(int number) { return...Ch. 16 - Prob. 39RQECh. 16 - Assume the following definition appears in a...Ch. 16 - Assume the following statement appears in a...Ch. 16 - Prob. 1PCCh. 16 - Prob. 2PCCh. 16 - Prob. 3PCCh. 16 - Prob. 4PCCh. 16 - Prob. 5PCCh. 16 - IntArray Class Exception Chapter 14 presented an...Ch. 16 - TestScores Class Write a class named TestScores....Ch. 16 - Prob. 8PCCh. 16 - Prob. 9PCCh. 16 - SortableVector Class Template Write a class...Ch. 16 - Inheritance Modification Assuming you have...Ch. 16 - Prob. 12PCCh. 16 - Prob. 13PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Code on phyton: Write a program that accepts an amino acid sequence. The outshould should be able to display the peptide fragments wherein this was obtained using the enzyme called chymotrypsin. Note that: This enzyme should cleave the carboxyl side of Phenylalanine, Tyrosine, and Tryptophan. Also remember that there is an exception to the rule when it comes to Proline. Output should be like this: Enter Amino Acid Sequence: MQKESLWARYLEIFVGNFPYL The peptide fragment/s are: MQKESLW ARY LEIF VGNFPY Larrow_forwardDIRECTION: Using Java as program language Create a simple program for a quiz bee with a class named QuizBee. The program shall:o Contain an array of 15 multiple choice questions with three (3) choiceseach and;o Require the user to choose among A, B or C.o Note: Cases are ignored. Lowercase letters are acceptable (a,b, or c) Create a try-catch structure to handle three (3) exceptions. These are when theuser inputs the following:o An invalid letter (not A, B or C)o A number or any special character.o Blank (no answer). Prompt the user to answer again if any of the three (3) exceptions is thrown. Display the score, number of incorrect answers and corrects answers.arrow_forwardPurpose To become familiar with throwing and catching exceptions. Directions: The Fibonacci series is a famous sequence of numbers that occurs often in nature, architecture, painting, etc. (you can more read about it on Wikipedia if you are interested). The sequence is defined as follows: • The first Fibonacci number is 1 • The second Fibonacci number is also 1 • The nth Fibonacci number is the sum of the previous two numbers. The first few numbers in the sequence are therefore: 1, 1, 2, 3, 5, 8, 13, 21, 34, … Your task is to write a method called fibonacci that accepts an integer argument called n and returns the nth Fibonacci number. Because this method only makes sense for positive values of n, your method should throw an exception (which you will need to create) of type InvalidArgumentException. Your method should have the following signature: public static int fibonacci(int n) throws InvalidArgumentException Hint: One way to implement the fibonacci method is to have two variables…arrow_forward
- answer in java languagearrow_forwardC++ PROGRAM EXCEPTION HANDLING Write a program with a function that accepts a string from user. The function prints “Valid String” only when the string contains at least one capital letter, one vowel, one integer and one ‘@’ character. An example of a valid string is @Developer123. If the user enters any operators like +,*,= then your program must throw a character exception. If the string contains any brackets like {[(}]), then your program must throw an integer exception. Write appropriate catch blocks of these exceptions.arrow_forwardPlease debug this code. // Handles a Format Exception if user does not enter a number using System; using static System.Console; using System.Globalization; class DebugEleven01 { static void Main() { double salary; string salVal; bool isValidSalary; while(!isValidSalary) { try Write("Enter an employee's salary "); salVal = ReadLine(); salary = Convert.ToDouble(salVal); isValidSalary = true; catch(Formatexception) { WriteLine("You must enter a number for the salary."); } } WriteLine("The salary {0} is valid", salary.ToString("C2", CultureInfo.GetCultureInfo("en-US"))); } }arrow_forward
- Homework - identical arrays for one-dimensional: Write a test program which prompts a user to enter the size of two arrays as well as to fill their elements. After that, the program checks the same contents of the elements of the two arrays. If these arrays are equal, the program displays message which is "The two arrays are identical". If not equal, displaying "The two arrays are not identical". Write an equals method that reads two arrays and displays these messages above.arrow_forwardC PROGRAMMING HELP I need help fixing an old assignment of mine. The point of the script is to read the pasted/attached Number_new.txt file's contents, meant to represent a classes' final grades out of 100 percent, and print an .exe screen as well as another .txt file that repeats the grade number with a letter grade and a PASS or FAIL next to it. At the end it needs to say how many students passed and how many failed. Nothing too complicated with the grading, just 90.0 and up is an A, 80.0 to 89.99 is a B, etc. And of course, anything below 60.0 is an F/FAIL. Please NO IOSTREAM.H OR CACIO.H! I cant use those header files. Below is the script: #include<stdio.h>#include<math.h>#define THEFILE "Number_new.txt" int main(){ float r0[10]; float rr[10]; FILE *fp; char line[81]; fp = fopen(THEFILE, "Number_new.txt"); if (fp != NULL) { /* The file is open. */ printf("%7s %7s %7s %7s %7s %7s %7s %7s %7s %7s\n", "0", "0.01",…arrow_forwardin c++ Write a function named createTwoNames that will do the following: - Read in a word and its definition - Create the Word object for that word and definition - Read in an item’s name and its price - Create the Item object for that item - It will return an array of two pointers pointing to these newly created Word and Item objects. It will use try-catch statement to handle the exception. If the user enters an empty or all-blank word or definition or item’s name, it will not create the object(s) and return null for that object only. In another words, it may create 0, 1 or 2 objects. Whenever the error occurs, it must print out the correct error reason: empty or blank (but not both). Note that this function can and will use cin and cout to read in values from the user.arrow_forward
- You can dereference a smart pointer with the * operator. True Falsearrow_forwardC++ PROGRAM EXCEPTION HANDLING Write a program with a function that takes a string as an argument from user which displays the string in descending lexicographical order (dictionary order). Suppose user enters ahmad then output should be mhdaa. The program should contain at least 5 characters. If there are less than 5 characters, then your program must throw an integer exception. If the user enters only numeric characters, then your program must also throw an integer exception. Write appropriate catch blocks for exceptions.arrow_forwardIn C++Using the code provided belowDo the Following: Modify the Insert Tool Function to ask the user if they want to expand the tool holder to accommodate additional tools Add the code to the insert tool function to increase the capacity of the toolbox (Dynamic Array) USE THE FOLLOWING CODE and MODIFY IT: #define _SECURE_SCL_DEPRECATE 0 #include <iostream> #include <string> #include <cstdlib> using namespace std; class GChar { public: static const int DEFAULT_CAPACITY = 5; //constructor GChar(string name = "john", int capacity = DEFAULT_CAPACITY); //copy constructor GChar(const GChar& source); //Overload Assignment GChar& operator=(const GChar& source); //Destructor ~GChar(); //Insert a New Tool void insert(const std::string& toolName); private: //data members string name; int capacity; int used; string* toolHolder; }; //constructor GChar::GChar(string n, int cap) { name = n; capacity = cap; used = 0; toolHolder = new…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
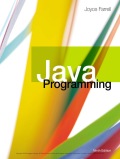
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
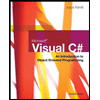
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,