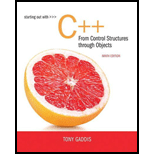
Starting Out with C++ from Control Structures to Objects (9th Edition)
9th Edition
ISBN: 9780134498379
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 16, Problem 18RQE
Write a function that dynamically allocates a block of memory and returns a char pointer to the block. The function should take an integer argument that is the amount of memory to be allocated. If the new operator cannot allocate the memory, the function should return a null pointer.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
parameter list can also contain the data type of the output of function : true/false
a function declared int addition (int a and b) is capable of returning one value back to the main loop : true/false
main () is a void function: true / false
the address returned by the reference pointer is always the same regardless of operating system: true/false
a function declares as int addition (int a, int b) has a and b as output arguments : true/ false
In C programming:
Write a function printAllCourses() which receives an array of course pointers and the array’s size, then prints all courses in the array by calling printCourseRow()
In this assignment you are to use pointers to manipulate dynamically created arrays.
An array's name is basically a pointer to the fisrt element of the array. Dynamic arrays are created using pointer syntax, but
can subsequently use array syntax, which is the best practice approach.
Instructions (main.cpp)
Inside main.cpp implement the following functions:
int makeArray (const int size);
This function dynamically allocates an int array of the specified size.
• size the size of the dynamically allocated array.
• returns - a dynamically allocated integer array of size elements.
void initializeArray (int * array, const int size, const int initValue);
This function initializes an array of size elements with the initValue.
array-The array whose elements are to be initialized.
size the size of the array.
initValue the value with which to initialize each element.
int
duplicateArray (const int const sourceArray, const int size);
This function duplicates an array of size elements. When copying…
Chapter 16 Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Ch. 16.1 - Prob. 16.1CPCh. 16.1 - Prob. 16.2CPCh. 16.1 - Prob. 16.3CPCh. 16.1 - Prob. 16.4CPCh. 16.1 - Prob. 16.5CPCh. 16.3 - Prob. 16.6CPCh. 16.3 - The following function accepts an i nt argument...Ch. 16.3 - Prob. 16.8CPCh. 16.3 - Prob. 16.9CPCh. 16.4 - Prob. 16.10CP
Ch. 16.4 - Prob. 16.11CPCh. 16 - Prob. 1RQECh. 16 - Prob. 2RQECh. 16 - Prob. 3RQECh. 16 - Prob. 4RQECh. 16 - What is unwinding the stack?Ch. 16 - What happens if an exception is thrown by a classs...Ch. 16 - How do you prevent a program from halting when the...Ch. 16 - Why is it more convenient to write a function...Ch. 16 - Why must you be careful when writing a function...Ch. 16 - The line containing a throw statement is known as...Ch. 16 - Prob. 11RQECh. 16 - Prob. 12RQECh. 16 - Prob. 13RQECh. 16 - The beginning of a template is marked by a(n)...Ch. 16 - Prob. 15RQECh. 16 - Prob. 16RQECh. 16 - Write a function that searches a numeric array for...Ch. 16 - Write a function that dynamically allocates a...Ch. 16 - Make the function you wrote in Question 17 a...Ch. 16 - Write a template for a function that displays the...Ch. 16 - Prob. 21RQECh. 16 - Prob. 22RQECh. 16 - Prob. 23RQECh. 16 - Prob. 24RQECh. 16 - T F All type parameters defined in a function...Ch. 16 - Prob. 26RQECh. 16 - T F A class object passed to a function template...Ch. 16 - Prob. 28RQECh. 16 - Prob. 29RQECh. 16 - Prob. 30RQECh. 16 - Prob. 31RQECh. 16 - T F A class template may not be derived from...Ch. 16 - T F A class template may not be used as a base...Ch. 16 - Prob. 34RQECh. 16 - Prob. 35RQECh. 16 - try { quotient = divide(num1, num2); } cout The...Ch. 16 - template class T T square(T number) { return T T;...Ch. 16 - template class T int square(int number) { return...Ch. 16 - Prob. 39RQECh. 16 - Assume the following definition appears in a...Ch. 16 - Assume the following statement appears in a...Ch. 16 - Prob. 1PCCh. 16 - Prob. 2PCCh. 16 - Prob. 3PCCh. 16 - Prob. 4PCCh. 16 - Prob. 5PCCh. 16 - IntArray Class Exception Chapter 14 presented an...Ch. 16 - TestScores Class Write a class named TestScores....Ch. 16 - Prob. 8PCCh. 16 - Prob. 9PCCh. 16 - SortableVector Class Template Write a class...Ch. 16 - Inheritance Modification Assuming you have...Ch. 16 - Prob. 12PCCh. 16 - Prob. 13PC
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
What do you call a program that translates a high-level language program into a separate machine language progr...
Starting out with Visual C# (4th Edition)
Drives License Exam The local drivers license office has asked you to design a program that grades the written ...
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
What is a router?
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
3.12 (Date Create a class called Date that includes three pieces Of information as data
members—a month (type ...
C++ How to Program (10th Edition)
Finish the program that takes a word as a command-line argument and looks up the word to see whether it is in t...
Programming in C
Explain why the rapid delivery and deployment of new systems is often more important to businesses than the det...
Software Engineering (10th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- In this assignment you are to use pointers to manipulate dynamically created arrays. An array's name is basically a pointer to the fisrt element of the array. Dynamic arrays are created using pointer syntax, but can subsequently use array syntax, which is the best practice approach. Instructions (main.cpp) Inside main.cpp implement the following functions: int makeArray (const int size); This function dynamically allocates an int array of the specified size. • size the size of the dynamically allocated array. • returns - a dynamically allocated integer array of size elements. void initializeArray (int * array, const int size, const int initValue); This function initializes an array of size elements with the initValue. array The array whose elements are to be initialized. size the size of the array. initValue the value with which to initialize each element. int duplicateArray (const int const sourceArray, const int size); This function duplicates an array of size elements. When copying…arrow_forwardIn C Programming: Write a function inputAllCourses() which receives an array of course pointers and the array’s size, then allows the user to input all courses in the array by calling inputCourse()arrow_forwardIn C++, When an array is passed to a function as a pointer, the function doesn't know the size of the array. List 3 ways to handle this problem.arrow_forward
- In c++, please and thank you! Write a function that dynamically allocates an array of integers. The function should accept an integer argument indicating the number of elements to allocate. The function should return a pointer to the array.arrow_forwardProgramming language C++ NB :Make use of pointers to solve the following programarrow_forwardArray Expander - Use Pointer Notation for the function and within the function. Use a main function and return the pointer from the ArrayExpander function to mainarrow_forward
- in carrow_forwardCreate a function that can accept a pointers and array with values of 1000, 2, 3, 17, 50. Your program will display the array values and its averagearrow_forwardProgramming language: C++ Implement a function named insert that takes a dynamically allocated array of ints, the array’s length, the index at which a new value should be inserted, and the new value that should be inserted. The function should allocate a new array populated with the contents of the original array plus the new value inserted at the given index. The originally array should be freed. The following sections provide a detailed description of this function: Make sure your source code is well-commented, consistently formatted, uses no magic numbers/values, follows programming best-practices, and is ANSI-compliant. It is expected to have the program outputarrow_forward
- C Program Functions and Pointers Create a function called terminate that asks: integer array to be referenced for modification integer value to serve as an indexing variable. This function sets the value of an element in the array to 0 based on the index value provided. This function does not return anything. In the main function, write a program that asks the user for an integer input and call the terminate function by passing the array and the inputted integer. Print the contents of the modified array where each elements are separated by a space. Input 1. One line containing an integer input Output Ten int outputs each separated by a space Enter·a·number(0-9):·1 1·0·1·1·1·1·1·1·1·1 Create a function called terminate that asks: integer array to be referenced for modification integer value to serve as an indexing variable. This function sets the value of an element in the array to 0 based on the index value provided. This function does not return anything.…arrow_forwardC++ Write a function that accepts an int array and the array’s size as arguments. The function should create a new array that is twice the size of the argument array. The function should copy the contents of the argument array to the new array and initialize the unused elements of the second array with 0. The function should return a pointer to the new array. Demonstrate the function in a complete program.arrow_forwardin c++ In statistics, the mode of a set of values is the value that occurs most often or with the greatest frequency. Write a function that accepts as arguments the following: A) An array of integers B) An integer that indicates the number of elements in the array The function should determine the mode of the array. That is, it should determine which value in the array occurs most often. The mode is the value the function should return. If the array has no mode (none of the values occur more than once), the function should return −1. (Assume the array will always contain nonnegative values.) Demonstrate your pointer prowess by using pointer notation instead of array notation in this function.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
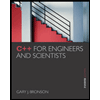
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
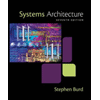
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
1.1 Arrays in Data Structure | Declaration, Initialization, Memory representation; Author: Jenny's lectures CS/IT NET&JRF;https://www.youtube.com/watch?v=AT14lCXuMKI;License: Standard YouTube License, CC-BY
Definition of Array; Author: Neso Academy;https://www.youtube.com/watch?v=55l-aZ7_F24;License: Standard Youtube License