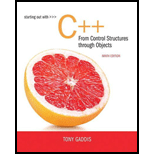
Starting Out with C++ from Control Structures to Objects (9th Edition)
9th Edition
ISBN: 9780134498379
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 16, Problem 16RQE
A template which is designed to work with a specific data type is referred as “specialized template”.
Program Plan Intro
Function template:
In C++, a function template is referred as a “generic” function, which can work with different data types.
- While writing a function template, a programmer can specify the “type parameter” instead of using the actual data type.
- The compiler generates the code, when it encounters a function call to a function template. This code will handle the particular data type which is used in the function call.
Example:
For example consider the below function template used to find the cube of given value for any types:
//template function
template <class T>
//function definition of "cube"
T cube(T x)
{
//return the cube of the value
return x*x*x ;
}
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
I want to ask someone who has experiences in writing physics based simulation software. For context I am building a game engine, and want to implement physics simulation. There are a few approaches that I managed to find, but would like to know what are other approaches to doing physics simulation entry points from scenes, would you be able to visually draw me a few approaches (like 3 approaces)?
When I say entry point to the actual physics simulation. An example of this is when the user presses the play button in the editor, it starts and initiates the physics system. Applying all of the global physics settings parameters that gets applied to that scene.
Here is the use-case, I am looking for. If you have two scenes, and select scene 1. You press the play button. The physics simulation starts. When that physics simulation starts, you are also having to update the physics through some physics dedicated delta time because physics needs to happen faster update frequency.
To elaborate,…
I want to ask someone who has experiences in writing physics based simulation software. For context I am building a game engine, and want to implement physics simulation. There are a few approaches that I managed to find, but would like to know what are other approaches to doing physics simulation entry points from scenes, would you be able to visually draw me a few approaches (like 3 approaces)?When I say entry point to the actual physics simulation. An example of this is when the user presses the play button in the editor, it starts and initiates the physics system. Applying all of the global physics settings parameters that gets applied to that scene.Here is the use-case, I am looking for. If you have two scenes, and select scene 1. You press the play button. The physics simulation starts. When that physics simulation starts, you are also having to update the physics through some physics dedicated delta time because physics needs to happen faster update frequency.To elaborate, what…
Male comedians were typically the main/dominant star of television sitcoms made during the FCC licensing freeze.
Question 19 options:
True
False
In the episode of The Honeymooners that you watched this week, why did Alice decide to get a job outside of the home?
Question 1 options:
to earn enough money to buy a mink coat
to have something to do while the kids were at school
to pay the bills after her husband got laid off
Chapter 16 Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Ch. 16.1 - Prob. 16.1CPCh. 16.1 - Prob. 16.2CPCh. 16.1 - Prob. 16.3CPCh. 16.1 - Prob. 16.4CPCh. 16.1 - Prob. 16.5CPCh. 16.3 - Prob. 16.6CPCh. 16.3 - The following function accepts an i nt argument...Ch. 16.3 - Prob. 16.8CPCh. 16.3 - Prob. 16.9CPCh. 16.4 - Prob. 16.10CP
Ch. 16.4 - Prob. 16.11CPCh. 16 - Prob. 1RQECh. 16 - Prob. 2RQECh. 16 - Prob. 3RQECh. 16 - Prob. 4RQECh. 16 - What is unwinding the stack?Ch. 16 - What happens if an exception is thrown by a classs...Ch. 16 - How do you prevent a program from halting when the...Ch. 16 - Why is it more convenient to write a function...Ch. 16 - Why must you be careful when writing a function...Ch. 16 - The line containing a throw statement is known as...Ch. 16 - Prob. 11RQECh. 16 - Prob. 12RQECh. 16 - Prob. 13RQECh. 16 - The beginning of a template is marked by a(n)...Ch. 16 - Prob. 15RQECh. 16 - Prob. 16RQECh. 16 - Write a function that searches a numeric array for...Ch. 16 - Write a function that dynamically allocates a...Ch. 16 - Make the function you wrote in Question 17 a...Ch. 16 - Write a template for a function that displays the...Ch. 16 - Prob. 21RQECh. 16 - Prob. 22RQECh. 16 - Prob. 23RQECh. 16 - Prob. 24RQECh. 16 - T F All type parameters defined in a function...Ch. 16 - Prob. 26RQECh. 16 - T F A class object passed to a function template...Ch. 16 - Prob. 28RQECh. 16 - Prob. 29RQECh. 16 - Prob. 30RQECh. 16 - Prob. 31RQECh. 16 - T F A class template may not be derived from...Ch. 16 - T F A class template may not be used as a base...Ch. 16 - Prob. 34RQECh. 16 - Prob. 35RQECh. 16 - try { quotient = divide(num1, num2); } cout The...Ch. 16 - template class T T square(T number) { return T T;...Ch. 16 - template class T int square(int number) { return...Ch. 16 - Prob. 39RQECh. 16 - Assume the following definition appears in a...Ch. 16 - Assume the following statement appears in a...Ch. 16 - Prob. 1PCCh. 16 - Prob. 2PCCh. 16 - Prob. 3PCCh. 16 - Prob. 4PCCh. 16 - Prob. 5PCCh. 16 - IntArray Class Exception Chapter 14 presented an...Ch. 16 - TestScores Class Write a class named TestScores....Ch. 16 - Prob. 8PCCh. 16 - Prob. 9PCCh. 16 - SortableVector Class Template Write a class...Ch. 16 - Inheritance Modification Assuming you have...Ch. 16 - Prob. 12PCCh. 16 - Prob. 13PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- After the FCC licensing freeze was lifted, sitcoms featuring urban settings and working class characters became far less common. Question 14 options: True Falsearrow_forwardsolve this questions for me .arrow_forwarda) first player is the minimizing player. What move should be chosen?b) What nodes would not need to be examined using the alpha-beta pruning procedure?arrow_forward
- Consider the problem of finding a path in the grid shown below from the position S to theposition G. The agent can move on the grid horizontally and vertically, one square at atime (each step has a cost of one). No step may be made into a forbidden crossed area. Inthe case of ties, break it using up, left, right, and down.(a) Draw the search tree in a greedy search. Manhattan distance should be used as theheuristic function. That is, h(n) for any node n is the Manhattan distance from nto G. The Manhattan distance between two points is the distance in the x-directionplus the distance in the y-direction. It corresponds to the distance traveled along citystreets arranged in a grid. For example, the Manhattan distance between G and S is4. What is the path that is found by the greedy search?(b) Draw the search tree in an A∗search. Manhattan distance should be used as thearrow_forwardwhats for dinner? pleasearrow_forwardConsider the follow program that prints a page number on the left or right side of a page. Define and use a new function, isEven, that returns a Boolean to make the condition in the if statement easier to understand. ef main() : page = int(input("Enter page number: ")) if page % 2 == 0 : print(page) else : print("%60d" % page) main()arrow_forward
- What is the correct python code for the function def countWords(string) that will return a count of all the words in the string string of workds that are separated by spaces.arrow_forwardConsider the following program that counts the number of spaces in a user-supplied string. Modify the program to define and use a function, countSpaces, instead. def main() : userInput = input("Enter a string: ") spaces = 0 for char in userInput : if char == " " : spaces = spaces + 1 print(spaces) main()arrow_forwardWhat is the python code for the function def readFloat(prompt) that displays the prompt string, followed by a space, reads a floating-point number in, and returns it. Here is a typical usage: salary = readFloat("Please enter your salary:") percentageRaise = readFloat("What percentage raise would you like?")arrow_forward
- assume python does not define count method that can be applied to a string to determine the number of occurances of a character within a string. Implement the function numChars that takes a string and a character as arguments and determined and returns how many occurances of the given character occur withing the given stringarrow_forwardConsider the ER diagram of online sales system above. Based on the diagram answer the questions below, a) Based on the ER Diagram, determine the Foreign Key in the Product Table. Just mention the name of the attribute that could be the Foreign Key. b) Mention the relationship between the Order and Customer Entities. You can use the following: 1:1, 1:M, M:1, 0:1, 1:0, M:0, 0:M c) Is there a direct relationship that exists between Store and Customer entities? Answer Yes/No? d) Which of the 4 Entities mention in the diagram can have a recursive relationship? e) If a new entity Order_Details is introduced, will it be a strong entity or weak entity? If it is a weak entity, then mention its type?arrow_forwardNo aiarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
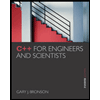
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
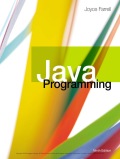
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
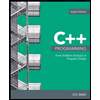
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
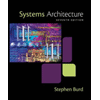
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
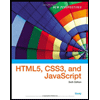
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning