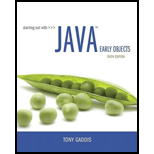
Concept explainers
Ackermarm’s Function
Ackermann’s function is a recursive mathematical
If m = 0 then return n + 1
If n = 0 then return ackermann(m - 1, 1)
Otherwise, return ackermann(m - 1, ackermann(m, n - 1))
Test your method in a program that displays the return values of the following method calls:
ackermann(0, 0) ackermann(0, 1) ackermann(1, 1) ackermann(1, 2)
ackermann(1, 3) ackermann(2, 2) ackermann(3, 2)

Want to see the full answer?
Check out a sample textbook solution
Chapter 14 Solutions
Starting Out with Java: Early Objects (6th Edition)
Additional Engineering Textbook Solutions
Introduction To Programming Using Visual Basic (11th Edition)
Modern Database Management (12th Edition)
Introduction to Programming Using Visual Basic (10th Edition)
Computer Science: An Overview (12th Edition)
Starting Out With Visual Basic (7th Edition)
Modern Database Management
- For function addOdd(n) write the missing recursive call. This function should return the sum of all postive odd numbers less than or equal to n. Examples: addOdd(1) -> 1addOdd(2) -> 1addOdd(3) -> 4addOdd(7) -> 16 public int addOdd(int n) { if (n <= 0) { return 0; } if (n % 2 != 0) { // Odd value return <<Missing a Recursive call>> } else { // Even value return addOdd(n - 1); }}arrow_forwardAckermann’s Functionarrow_forwardWrite a recursive function that returns the nth Fibonacci number from the Fibonacciseries.int fib(int n);arrow_forward
- write a code in java (using recursion)arrow_forward1. Write a recursive method expFive(n) to compute y=5^n. For instance, if n is 0, y is 1. If n is 3, then y is 125. If n is 4, then y is 625. The recursive method cannot have loops. Then write a testing program to call the recursive method. If you run your program, the results should look like this: > run RecExpTest Enter a number: 3 125 >run RecExpTest Enter a number: 3125 2. For two integers m and n, their GCD(Greatest Common Divisor) can be computed by a recursive function. Write a recursive method gcd(m,n) to find their Greatest Common Divisor. Once m is 0, the function returns n. Once n is 0, the function returns m. If neither is 0, the function can recursively calculate the Greatest Common Divisor with two smaller parameters: One is n, the second one is m mod n. Although there are other approaches to calculate Greatest Common Divisor, please follow the instructions in this question, otherwise you will not get the credit. Meaning your code needs to follow the given algorithm. Then…arrow_forwardWrite recursive and iterative methods to compute the summation of following series: ao aj az az an 5 20 80 320 4an-1 For example: a, = 5, n = 4 public static double m(.. , ..) public static double iterative_m( .. , ..) ao + az + a, +az = 425arrow_forward
- Java language Write a recursive method to add all of the odd numbers between two numbers (start and end) and return the result. The method receives these numbers as parameters.arrow_forwardConsider the following recursive method: Public static int Fib(int a1, int a2, int n){ if(n == 1) return a1; else if (n == 2) return a2;else return Fib(a1, a2, n-1) + Fib(a1, a2, n-2);} Please draw the recursion trace for Fib(2,3,5)arrow_forward8. Ackerman's Function Ackermann's Function is a recursive mathematical algorithm that can be used to test how well a system optimizes its performance of recursion. Design a function ackermann(m, n), which solves Ackermann's function. Use the following logic in your function: If m = 0 then return n + 1 If n = 0 then return ackermann(m-1,1) Otherwise, return ackermann(m-1,ackermann(m,n-1)) Once you've designed yyour function, test it by calling it with small values for m and n. Use Python.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
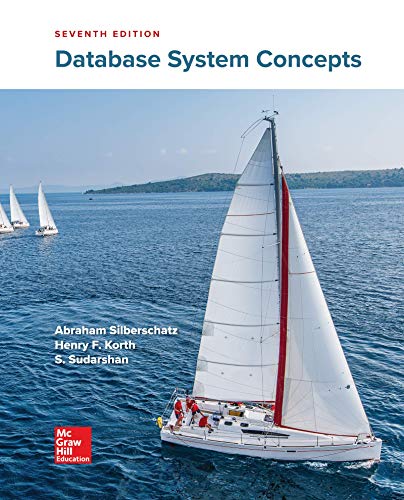
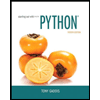
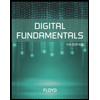
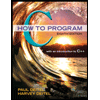
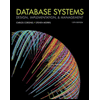
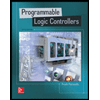