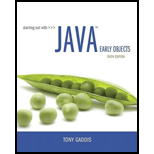
Starting Out with Java: Early Objects (6th Edition)
6th Edition
ISBN: 9780134462011
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 14, Problem 1AW
Program Plan Intro
- Define the class definition.
- Define the main method using public static void main(String[] args).
- Declare the string data fields and pass the required arguments.
- Close the main method.
- Define the recursive method.
- Define if condition to compare the string length.
- Print the required data fields using System.out.println() method.
- Close the recursive method.
- Close the public class.
- Define the main method using public static void main(String[] args).
- Declare the string data fields and pass the required arguments.
- Close the main method.
- Define the recursive method.
- Define if condition to compare the string length.
- Print the required data fields using System.out.println() method.
- Close the recursive method.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Alternate Character DisplayWrite a method that accepts a String object as an argument and then returns the alternate characters as substring starting from index 0. For instance, if the string argument passed is “GREAT”, the method should return “GET”. Demonstrate the method in a program that asks the user to input a string and then passes it to the method.
In the class String, the substring method inserts a String into another String.
-True
or
-False
Java coding
Strings as arguments
Chapter 14 Solutions
Starting Out with Java: Early Objects (6th Edition)
Ch. 14.2 - It is said that a recursive algorithm has more...Ch. 14.2 - Prob. 14.2CPCh. 14.2 - What is a recursive case?Ch. 14.2 - What causes a recursive algorithm to stop calling...Ch. 14.2 - What is direct recursion? What is indirect...Ch. 14 - Prob. 1MCCh. 14 - This is the part of a problem that can be solved...Ch. 14 - This is the part of a problem that is solved with...Ch. 14 - This is when a method explicitly calls itself. a....Ch. 14 - Prob. 5MC
Ch. 14 - Prob. 6MCCh. 14 - True or False: An iterative algorithm will usually...Ch. 14 - True or False: Some problems can be solved through...Ch. 14 - True or False: It is not necessary to have a base...Ch. 14 - True or False: In the base case, a recursive...Ch. 14 - Find the error in the following program: public...Ch. 14 - Prob. 1AWCh. 14 - Prob. 2AWCh. 14 - What will the following program display? public...Ch. 14 - Prob. 4AWCh. 14 - What will the following program display? public...Ch. 14 - Convert the following iterative method to one that...Ch. 14 - Write an iterative version (using a loop instead...Ch. 14 - What is the difference between an iterative...Ch. 14 - What is a recursive algorithms base case? What is...Ch. 14 - What is the base case of each of the recursive...Ch. 14 - What type of recursive method do you think would...Ch. 14 - Which repetition approach is less efficient: a...Ch. 14 - When recursion is used to solve a problem, why...Ch. 14 - How is a problem usually reduced with a recursive...Ch. 14 - Prob. 1PCCh. 14 - isMember Method Write a recursive boolean method...Ch. 14 - String Reverser Write a recursive method that...Ch. 14 - maxElement Method Write a method named maxElement,...Ch. 14 - Palindrome Detector A palindrome is any word,...Ch. 14 - Character Counter Write a method that uses...Ch. 14 - Recursive Power Method Write a method that uses...Ch. 14 - Sum of Numbers Write a method that accepts an...Ch. 14 - Ackermarms Function Ackermanns function is a...Ch. 14 - Recursive Population Class In Programming...
Knowledge Booster
Similar questions
- Along with driver codearrow_forwardAll the Same Letter Write a method to determine if all the letters in a String are the same letter. public boolean sameLetter(String str) { //declare first character variable //create statements to compare each character and include return statement //create other return statement }arrow_forwardCodeWorkout Gym Course Search exercises... Q Search kola shreya@columbus X275: Recursion Programming Exercise: Check Palindrome X275: Recursion Programming Exercise: Check Palindrome Write a recursive function named checkPalindrome that takes a string as input, and returns true if the string is a palindrome and false if it is not a palindrome. A string is a palindrome if it reads the same forwards or backwards. Recall that str.charAt(a) will return the character at position a in str. str.substring(a) will return the substring of str from position a to the end of str,while str.substring(a, b) will return the substring of str starting at position a and continuing to (but not including) the character at position b. Examples: checkPalindrome ("madam") -> true Your Answer: 1 public boolean checkPalindrome (String s) { 4 CodeWorkout © Virginia Tech About License Privacy Contactarrow_forward
- JAVA PPROGRAM ASAP Please create this program ASAP BECAUSE IT IS MY LAB ASSIGNMENT so it passes all the test cases. The program must pass the test case when uploaded to Hypergrade. Chapter 9. PC #2. Word Counter (page 608) Write a method that accepts a String object as an argument and returns the number of words it contains. For instance, if the argument is “Four score and seven years ago” the method should return the number 6. Demonstrate the method in a program that asks the user to input a string and then passes it to the method. The number of words in the string should be displayed on the screen. Test Case 1 Please enter a string or type QUIT to exit:\nHello World!ENTERThere are 2 words in that string.\nPlease enter a string or type QUIT to exit:\nI have a dream.ENTERThere are 4 words in that string.\nPlease enter a string or type QUIT to exit:\nJava is great.ENTERThere are 3 words in that string.\nPlease enter a string or type QUIT to exit:\nquitENTERarrow_forwardProblem Description. Arguments for the main method are passed as strings. Strings enclosed in quotation marks are considered as one argument. Write a program to parse arguments from a string. Arguments are separated by spaces. Enclosed strings are considered as one argument. Your program should prompt the user to enter a string and display the arguments, each per line, as shown in the following sample run. <input> Enter the arguments: a friend "good morning" "good afternoon" b <output> a friend good morning good afternoon b please provide the answer in JAVAarrow_forward17arrow_forward
- the function returns the result as a string if the expression is legal. the expression returns an empty string and then put the function public class Ez1MathEval {public String heavywtEval (String exprn) {return ""}}arrow_forwardRecursive Power MethodWrite a method called powCal that uses recursion to raise a number to a power. The method should accept two arguments: The first argument is the exponent and the second argument is the number to be raised (example” powCal(10,2) means 210). Assume that the exponent is a nonnegative integer. Demonstrate the method in a program called Recursive (This means that you need to write a program that has at least two methods: main and powCal. The powCal method is where you implement the requirements above and the main method is where you make a method call to demonstrate how your powCal method work).arrow_forwardRepeating String Write a method that takes a String and an int and return a String that is the original String repeated n times. For example, repeatString("Famous", 3) should return "FamousFamousFamous" The method signature should be public String repeatString(String str, int n) public String repeatString(String str, int n) { //declare a string variable //set up statements for repeating n number of times //create the appropriate return statement }arrow_forward
- C# Write a method that accepts a string as an argument and displaysits contents backward. For instance, if the string argument is"gravity" , the method should display "ytivarg"arrow_forwardMagic Number Code question::-1.A number is said to be a magic number,if summing the digits of the number and then recursively repeating this process for the given sumuntill the number becomes a single digit number equal to 1. Example: Number = 50113 => 5+0+1+1+3=10 => 1+0=1 [This is a Magic Number] Number = 1234 => 1+2+3+4=10 => 1+0=1 [This is a Magic Number] Number = 199 => 1+9+9=19 => 1+9=10 => 1+0=1 [This is a Magic Number] Number = 111 => 1+1+1=3 [This is NOT a Magic Number].arrow_forwardlanguage using - Javaarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
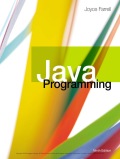
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
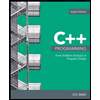
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning