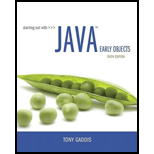
Starting Out with Java: Early Objects (6th Edition)
6th Edition
ISBN: 9780134462011
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 14, Problem 1PC
Program Plan Intro
- Import javax.swing.JOptionPane library.
- Define the public class definition.
- Define the main method using public static void main(String[] args).
- Declare double variables to pass the required arguments.
- Get the required value of the first number using input() method and store in num1 variable.
- Get the required value of the second number using input() method and store in num2 variable.
- Calculate their product and display it.
- Close the main method.
- Define the recursive method to multiply the variables.
- Define if condition and equate the variable x with 1.
- Return the value stored in the variable y.
- Define else condition.
- Return the value stored in the variable y.
- Close the recursive method.
- Close the public class.
- Define the main method using public static void main(String[] args).
- Declare double variables to pass the required arguments.
- Get the required value of the first number using input() method and store in num1 variable.
- Get the required value of the second number using input() method and store in num2 variable.
- Calculate their product and display it.
- Close the main method.
- Define the recursive method to multiply the variables.
- Define if condition and equate the variable x with 1.
- Return the value stored in the variable y.
- Define else condition.
- Return the value stored in the variable y.
- Close the recursive method.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Resolver por superposicion
Describe three (3) Multiplexing techniques common for fiber optic links
Could you help me to know features of the following concepts:
- commercial CA
- memory integrity
- WMI filter
Chapter 14 Solutions
Starting Out with Java: Early Objects (6th Edition)
Ch. 14.2 - It is said that a recursive algorithm has more...Ch. 14.2 - Prob. 14.2CPCh. 14.2 - What is a recursive case?Ch. 14.2 - What causes a recursive algorithm to stop calling...Ch. 14.2 - What is direct recursion? What is indirect...Ch. 14 - Prob. 1MCCh. 14 - This is the part of a problem that can be solved...Ch. 14 - This is the part of a problem that is solved with...Ch. 14 - This is when a method explicitly calls itself. a....Ch. 14 - Prob. 5MC
Ch. 14 - Prob. 6MCCh. 14 - True or False: An iterative algorithm will usually...Ch. 14 - True or False: Some problems can be solved through...Ch. 14 - True or False: It is not necessary to have a base...Ch. 14 - True or False: In the base case, a recursive...Ch. 14 - Find the error in the following program: public...Ch. 14 - Prob. 1AWCh. 14 - Prob. 2AWCh. 14 - What will the following program display? public...Ch. 14 - Prob. 4AWCh. 14 - What will the following program display? public...Ch. 14 - Convert the following iterative method to one that...Ch. 14 - Write an iterative version (using a loop instead...Ch. 14 - What is the difference between an iterative...Ch. 14 - What is a recursive algorithms base case? What is...Ch. 14 - What is the base case of each of the recursive...Ch. 14 - What type of recursive method do you think would...Ch. 14 - Which repetition approach is less efficient: a...Ch. 14 - When recursion is used to solve a problem, why...Ch. 14 - How is a problem usually reduced with a recursive...Ch. 14 - Prob. 1PCCh. 14 - isMember Method Write a recursive boolean method...Ch. 14 - String Reverser Write a recursive method that...Ch. 14 - maxElement Method Write a method named maxElement,...Ch. 14 - Palindrome Detector A palindrome is any word,...Ch. 14 - Character Counter Write a method that uses...Ch. 14 - Recursive Power Method Write a method that uses...Ch. 14 - Sum of Numbers Write a method that accepts an...Ch. 14 - Ackermarms Function Ackermanns function is a...Ch. 14 - Recursive Population Class In Programming...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Briefly describe the issues involved in using ATM technology in Local Area Networksarrow_forwardFor this question you will perform two levels of quicksort on an array containing these numbers: 59 41 61 73 43 57 50 13 96 88 42 77 27 95 32 89 In the first blank, enter the array contents after the top level partition. In the second blank, enter the array contents after one more partition of the left-hand subarray resulting from the first partition. In the third blank, enter the array contents after one more partition of the right-hand subarray resulting from the first partition. Print the numbers with a single space between them. Use the algorithm we covered in class, in which the first element of the subarray is the partition value. Question 1 options: Blank # 1 Blank # 2 Blank # 3arrow_forward1. Transform the E-R diagram into a set of relations. Country_of Agent ID Agent H Holds Is_Reponsible_for Consignment Number $ Value May Contain Consignment Transports Container Destination Ф R Goes Off Container Number Size Vessel Voyage Registry Vessel ID Voyage_ID Tonnagearrow_forward
- I want to solve 13.2 using matlab please helparrow_forwarda) Show a possible trace of the OSPF algorithm for computing the routing table in Router 2 forthis network.b) Show the messages used by RIP to compute routing tables.arrow_forwardusing r language to answer question 4 Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.arrow_forward
- using r language Obtain a bootstrap t confidence interval estimate for the correlation statistic in Example 8.2 (law data in bootstrap).arrow_forwardusing r language Compute a jackknife estimate of the bias and the standard error of the correlation statistic in Example 8.2.arrow_forwardusing r languagearrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
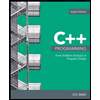
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
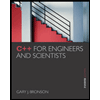
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
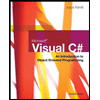
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
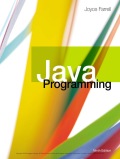
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
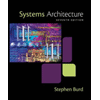
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning