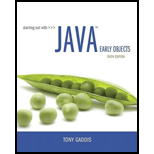
Starting Out with Java: Early Objects (6th Edition)
6th Edition
ISBN: 9780134462011
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 14, Problem 5PC
Palindrome Detector
A palindrome is any word, phrase, or sentence that reads the same forward and backward. Here are some well-known palindromes:
Able was I, ere I saw Elba
A man, a plan, a canal, Panama
Desserts, I stressed
Kayak
Write a boolean method that uses recursion to determine whether a String argument is a palindrome. The method should return true if the argument reads the same forward and backward. Demonstrate the method in a
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
In Java code do the following:Write a method that accepts a String as an argument. The method should use recursion to display each individual character in the String:
If your first name starts with a letter from A-J inclusively:
Write a recursive method that takes a string as argument and determines if the string has more vowels than consonants. Test the method by asking the user to enter a string. Hint: Write your recursive method to first count vowels and consonants.
Repeating String
Write a method that takes a String and an int and return a String that is the original String repeated n times.
For example, repeatString("Famous", 3) should return "FamousFamousFamous"
The method signature should be
public String repeatString(String str, int n)
public String repeatString(String str, int n)
{
//declare a string variable
//set up statements for repeating n number of times
//create the appropriate return statement
}
Chapter 14 Solutions
Starting Out with Java: Early Objects (6th Edition)
Ch. 14.2 - It is said that a recursive algorithm has more...Ch. 14.2 - Prob. 14.2CPCh. 14.2 - What is a recursive case?Ch. 14.2 - What causes a recursive algorithm to stop calling...Ch. 14.2 - What is direct recursion? What is indirect...Ch. 14 - Prob. 1MCCh. 14 - This is the part of a problem that can be solved...Ch. 14 - This is the part of a problem that is solved with...Ch. 14 - This is when a method explicitly calls itself. a....Ch. 14 - Prob. 5MC
Ch. 14 - Prob. 6MCCh. 14 - True or False: An iterative algorithm will usually...Ch. 14 - True or False: Some problems can be solved through...Ch. 14 - True or False: It is not necessary to have a base...Ch. 14 - True or False: In the base case, a recursive...Ch. 14 - Find the error in the following program: public...Ch. 14 - Prob. 1AWCh. 14 - Prob. 2AWCh. 14 - What will the following program display? public...Ch. 14 - Prob. 4AWCh. 14 - What will the following program display? public...Ch. 14 - Convert the following iterative method to one that...Ch. 14 - Write an iterative version (using a loop instead...Ch. 14 - What is the difference between an iterative...Ch. 14 - What is a recursive algorithms base case? What is...Ch. 14 - What is the base case of each of the recursive...Ch. 14 - What type of recursive method do you think would...Ch. 14 - Which repetition approach is less efficient: a...Ch. 14 - When recursion is used to solve a problem, why...Ch. 14 - How is a problem usually reduced with a recursive...Ch. 14 - Prob. 1PCCh. 14 - isMember Method Write a recursive boolean method...Ch. 14 - String Reverser Write a recursive method that...Ch. 14 - maxElement Method Write a method named maxElement,...Ch. 14 - Palindrome Detector A palindrome is any word,...Ch. 14 - Character Counter Write a method that uses...Ch. 14 - Recursive Power Method Write a method that uses...Ch. 14 - Sum of Numbers Write a method that accepts an...Ch. 14 - Ackermarms Function Ackermanns function is a...Ch. 14 - Recursive Population Class In Programming...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
A ______ structure can execute a set of statements only under certain circumstances. a. sequence b. circumstant...
Starting Out with Python (3rd Edition)
Explain why incremental development is the most effective approach for developing business software systems. Wh...
Software Engineering (10th Edition)
is a graphical language that allows people who design software systems to use an industry-standard notation to ...
Java How To Program (Early Objects)
Referring back to Questions 3 of Section 2.3, if the machine used the pipeline technique discussed in the text,...
Computer Science: An Overview (12th Edition)
What is the output of the following (when embedded in a complete program)? for (int n = 10; n 0; n = n 2) { c...
Problem Solving with C++ (9th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- JAVA programming language eclipse softwarearrow_forwardJAVA PPROGRAM ASAP Please create this program ASAP BECAUSE IT IS MY LAB ASSIGNMENT so it passes all the test cases. The program must pass the test case when uploaded to Hypergrade. Chapter 9. PC #2. Word Counter (page 608) Write a method that accepts a String object as an argument and returns the number of words it contains. For instance, if the argument is “Four score and seven years ago” the method should return the number 6. Demonstrate the method in a program that asks the user to input a string and then passes it to the method. The number of words in the string should be displayed on the screen. Test Case 1 Please enter a string or type QUIT to exit:\nHello World!ENTERThere are 2 words in that string.\nPlease enter a string or type QUIT to exit:\nI have a dream.ENTERThere are 4 words in that string.\nPlease enter a string or type QUIT to exit:\nJava is great.ENTERThere are 3 words in that string.\nPlease enter a string or type QUIT to exit:\nquitENTERarrow_forwardPrime Numbers A prime number is a number that can be evenly divided by only itself and 1. For example, the number 5 is prime because it can be evenly divided by only 1 and 5. The number 6, however, is not prime because it can be evenly divided by 1, 2, 3, and 6. Write a Boolean method named IsPrime that takes an integer as an argument and returns true if the argument is a prime number or false otherwise. Use the method in an application that lets the user enter a number and then displays a message indicating whether the number is prime.arrow_forward
- Free Response Question: 28. Write a method that will return true if a randomly generated number between 1 and 10 is less than or equal to 4. public static boolean FortyPercentOrLess() { }arrow_forwardJava Code public static method named oddsarrow_forwardAll the Same Letter Write a method to determine if all the letters in a String are the same letter. public boolean sameLetter(String str) { //declare first character variable //create statements to compare each character and include return statement //create other return statement }arrow_forward
- Topics Classes Methods Data Collections: Lists, Tuples, and Dictionaries String Manipulation Chess Objective: practicing with classes, instance methods, data collections, loops, if and elif statements, and string methods Description In this assignment you will write a program that shows the valid moves of chess pieces. Your program will draw a board with 64 squares using the traditional layout, next ask the user to choose a move, and then, depending on the user's choice, redraw the board with the selected chess piece and its valid moves. Please see the examples of valid moves of chess pieces and the traditional chess board layout below: At the beginning, your program should draw an empty chess board and prompt the user to enter a move: Welcome to the Chess Game! a b c d e f g h +---+---+---+---+---+---+---+---+8| | | | | | | | |8 +---+---+---+---+---+---+---+---+7| | | | | | | | |7 +---+---+---+---+---+---+---+---+6| | | | | |…arrow_forwardJAVA Problem description:You are to write a program called Seasons that prompts the user for a month and day and displays the season in which that date occurs. For reference, we will use the following date cutoffs for the various seasons: Winter December 21 – March 19 Spring March 20th – June 20th Summer June 21st – September 20th Fall September 21st – December 20th Data Validation For this program, you'll write a specific method to perform data validation based on the month and day entered. More specifically: Month – the month entered must be a valid month of the year (January – December). Day – the day entered must be a valid day for that month: January, March, May, July, August, October, December have 1-31 days. April, June, September, November have 1-30 days. February has 1-28 days (do not worry about leap years) Required program decomposition String getMonth(Scanner console) This method is called from the main method and should prompt for the month as a string…arrow_forwardSpecial methods ( variable ) - each student takes methods. - how does methods work (function). - write a program to use method and show output. P.S \ i chose max method java languagearrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
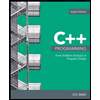
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
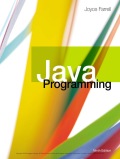
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Java random numbers; Author: Bro code;https://www.youtube.com/watch?v=VMZLPl16P5c;License: Standard YouTube License, CC-BY