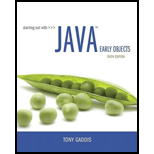
Starting Out with Java: Early Objects (6th Edition)
6th Edition
ISBN: 9780134462011
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 14, Problem 10PC
Recursive Population Class
In
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Lab Goal : This lab was designed to teach you more about recursion.
Lab Description : Take a number and recursively determine how many of its digits are even. Return the count of the even digits in each number.
% might prove useful to take the number apart digit by digitSample Data :
453211145322224532714246813579Sample Output :
23540
I want a code class and a runner class
Lab Goal : This lab was designed to teach you more about recursion.
Lab Description : luckyThrees will return a count of the 3s in the number unless the 3 is at the start. A 3 at the start of the number does not count.
/* luckyThrees will return the count of 3s in the number* unless the 3 is at the front and then it does not count* 3 would return 0* 31332 would return 2* 134523 would return 2* 3113 would return 1* 13331 would return 3* 777337777 would return 2* the solution to this problem must use recursion*/public static int luckyThrees( long number ){}
Sample Data :
331332134523311313331777337777
Sample Output :
022132
Charge Account Validation
Using Java programming
Create a class with a method that accepts a charge account number as its argument. The method should determine whether the number is valid by comparing it to the following list of valid charge account numbers:5658845 4520125 7895122 8777541 8451277 13028508080152 4562555 5552012 5050552 7825877 12502551005231 6545231 3852085 7576651 7881200 4581002These numbers should be stored in an array. Use a sequential search to locate the number passed as an argument. If the number is in the array, the method should return true, indicating the number is valid. If the number is not in the array, the method should return false, indicating the number is invalid.Write a program that tests the class by asking the user to enter a charge account number. The program should display a message indicating whether the number is valid or invalid.
Chapter 14 Solutions
Starting Out with Java: Early Objects (6th Edition)
Ch. 14.2 - It is said that a recursive algorithm has more...Ch. 14.2 - Prob. 14.2CPCh. 14.2 - What is a recursive case?Ch. 14.2 - What causes a recursive algorithm to stop calling...Ch. 14.2 - What is direct recursion? What is indirect...Ch. 14 - Prob. 1MCCh. 14 - This is the part of a problem that can be solved...Ch. 14 - This is the part of a problem that is solved with...Ch. 14 - This is when a method explicitly calls itself. a....Ch. 14 - Prob. 5MC
Ch. 14 - Prob. 6MCCh. 14 - True or False: An iterative algorithm will usually...Ch. 14 - True or False: Some problems can be solved through...Ch. 14 - True or False: It is not necessary to have a base...Ch. 14 - True or False: In the base case, a recursive...Ch. 14 - Find the error in the following program: public...Ch. 14 - Prob. 1AWCh. 14 - Prob. 2AWCh. 14 - What will the following program display? public...Ch. 14 - Prob. 4AWCh. 14 - What will the following program display? public...Ch. 14 - Convert the following iterative method to one that...Ch. 14 - Write an iterative version (using a loop instead...Ch. 14 - What is the difference between an iterative...Ch. 14 - What is a recursive algorithms base case? What is...Ch. 14 - What is the base case of each of the recursive...Ch. 14 - What type of recursive method do you think would...Ch. 14 - Which repetition approach is less efficient: a...Ch. 14 - When recursion is used to solve a problem, why...Ch. 14 - How is a problem usually reduced with a recursive...Ch. 14 - Prob. 1PCCh. 14 - isMember Method Write a recursive boolean method...Ch. 14 - String Reverser Write a recursive method that...Ch. 14 - maxElement Method Write a method named maxElement,...Ch. 14 - Palindrome Detector A palindrome is any word,...Ch. 14 - Character Counter Write a method that uses...Ch. 14 - Recursive Power Method Write a method that uses...Ch. 14 - Sum of Numbers Write a method that accepts an...Ch. 14 - Ackermarms Function Ackermanns function is a...Ch. 14 - Recursive Population Class In Programming...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
T F You must sort a range of elements before searching it with the binary_search() function.
Starting Out with C++ from Control Structures to Objects (9th Edition)
3.12 (Date Create a class called Date that includes three pieces Of information as data
members—a month (type ...
C++ How to Program (10th Edition)
Describe a method that can be used to gather a piece of data such as the users age.
Web Development and Design Foundations with HTML5 (9th Edition) (What's New in Computer Science)
Find the Error 33. The following pseudocode algorithm has an error. It is supposed to use values input for a re...
Starting Out with C++: Early Objects
Give the name of the algorithm that results from each of the following special cases: a. Local beam search with...
Artificial Intelligence: A Modern Approach
For Exercises 3.16-3.20, perform each of these steps: Read the problem statement. Formulate the algorithm using...
C How to Program (8th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- 3-The following pattern of numbers is called Pascal's triangle. 1 1 1 14 641 The numbers at the edge of the triangle are all 1, and each number inside the triangle is the sum of the two numbers above it. Write a procedure that computes elements of Pascal's triangle by means of a recursive process. 1 1 1 2 1 3 3arrow_forwardT/F: All recursive algorithms are efficient due to its recursive property.arrow_forwardRolling Dice Simulator [Java Assignment]Objective: Create a Java program that rolls two dice and displays the results. The program should have two Java classes: one for a single die and another for a pair of dice. Assignment Details: User Input: Ask the user to specify the number of sides they want on each die. Ensure that the user's input is within a reasonable range. Dice Rolling: Simulate rolling the dice using Math.random() based on the user's chosen number of sides. Display the sum of the values rolled, e.g., "5 + 3 = 8." Special Combinations: If the dice roll results in combinations of 2, 7, or 12, print special messages: "1 + 1 = 2 snake eyes!" "3 + 4 = 7 craps!" "6 + 6 = 12 box cars!" Main Method: In the main method, create a pair of dice, roll them, and display the results. Allow the user to decide whether to continue rolling the dice or exit the program. Additional Features: You are welcome to add more features or enhancements to the program if desired.…arrow_forward
- Topics Classes Methods Data Collections: Lists, Tuples, and Dictionaries String Manipulation Chess Objective: practicing with classes, instance methods, data collections, loops, if and elif statements, and string methods Description In this assignment you will write a program that shows the valid moves of chess pieces. Your program will draw a board with 64 squares using the traditional layout, next ask the user to choose a move, and then, depending on the user's choice, redraw the board with the selected chess piece and its valid moves. Please see the examples of valid moves of chess pieces and the traditional chess board layout below: At the beginning, your program should draw an empty chess board and prompt the user to enter a move: Welcome to the Chess Game! a b c d e f g h +---+---+---+---+---+---+---+---+8| | | | | | | | |8 +---+---+---+---+---+---+---+---+7| | | | | | | | |7 +---+---+---+---+---+---+---+---+6| | | | | |…arrow_forwardConsider the following pseudo code, Method func() { PRINT “This is recursive function" func() } Method main( { func() } What will happen when the above snippet is executed?arrow_forwardC++ language Write a menu driven program which has following options:1. Factorial2. Prime or not3. Odd or even4. ExitOnce a menu is selected, the appropriate action should be taken and once this action is finished,the menu should reappear. Unless the user selects the ‘Exit’ option the program should continueto work.Note: Consider array of random numbers from user to find Prime Numbers/Odd/Even and userentered value for factorial.arrow_forward
- T/F The body of a method may be emptyarrow_forwardObjectives Java refresher (including file I/O) Use recursion Description For this project, you get to write a maze solver. A maze is a two dimensional array of chars. Walls are represented as '#'s and ' ' are empty squares. The maze entrance is always in the first row, second column (and will always be an empty square). There will be zero or more exits along the outside perimeter. To be considered an exit, it must be reachable from the entrance. The entrance is not an exit.Here are some example mazes:mazeA 7 9 # # ##### # # # # # # # ### # # # # ##### # # # ######### mazeB 7 12 # ########## # # # # # # # #### # # # # # # ##### ## # # # # ############ mazeC 3 5 # # # ## ## Requirements Write a MazeSolver class in Java. This program needs to prompt the user for a maze filename and then explore the maze. Display how many exits were found and the positions (not indices) of the valid exits. Your program can display the…arrow_forwardPython question Application: Python Fragment Formulation (Q1 – Q4) In this group of questions you are asked to produce short pieces of Python code. When you are asked to "write a Python expression" to complete a task, you can either give the expression in one line or break down the task into several lines. The last expression you write must represent the required task. Question 1 (Reduce parentheses) Give an equivalent version of this expression by removing as many redundant parentheses as possible, without expanding the brackets or simplifying. (x**(2**y))+(y*((z+x)**3)) Question 2 (Translate arithmetic concept into Python) You are given a list of numbers, named numbers, containing 3 integers. Write a python expression (one line) that evaluates to True if and only if the product of any two numbers in the given list is greater than the sum of all three numbers. Note: the product of two numbers, x and y is x*y. Question 3 (List/table access) You are given a table,…arrow_forward
- Parking Charges Program in java Write a program that works out the amount a person has to pay for parking in a car park in a tourist town. If they say they are disabled, they are told it is free. Otherwise they enter the number of hours as a whole number (1-8) that they wish to park as well as whether they have an “I live locally” badge or are an old age pensioner both of which leads to a discount. The program tells them the cost to park. The calculation is done in this program as follows. If they are disabled it is free. Otherwise ... Take number of hours they wish to park and give a basic charge: • • • • 1 hour: 3.00 pounds 2-4 hours: 4.00 pounds 5-6 hours: 4.50 pounds 7-8 hours: 5.50 pounds Next modify the resulting charge based on whether they are local or not: • If local: subtract 1 pound If OAP: subtract 2 poundsYour program MUST include methods including ones that - asks for the hours and returns the basic charge. - asks whether they live locally /…arrow_forwardTreasure Hunter description You are in front of a cave that has treasures. The cave canrepresented in a grid which has rows numbered from 1to , and of the columns numbered from 1 to . For this problem, define (?, )is the tile that is in the -th row and -column.There is a character in each tile, which indicates the type of that tile.Tiles can be floors, walls, or treasures that are sequentially representedwith the characters '.' (period), '#' (hashmark), and '*' (asterisk). You can passfloor and treasure tiles, but can't get past wall tiles.Initially, you are in a tile (??, ). You want to visit all the treasure squares, andtake the treasure. If you visit the treasure chest, then treasurewill be instantly taken, then the tile turns into a floor.In a move, if you are in a tile (?, ), then you can move tosquares immediately above (? 1, ), right (?, + 1), bottom (? + 1, ), and left (?, 1) of thecurrent plot. The tile you visit must not be off the grid, and must not be awall patch.Determine…arrow_forwardvar adventurersName = ["George", " Tim", " Sarah", " Mike", " Edward"];var adventurersKilled = 3;var survivors;var leader = "Captain Thomas King";var numberOfAdventurers = adventurersName.length; survivors = numberOfAdventurers - adventurersKilled; console.log("Welcome to The God Among Us\n");console.log("A group of adventurers began their search for the mystical god said to live among us. In charge of the squad was " + leader + " who was famous for his past exploits. Along the way, the group of comrades were attacked by the god's loyal followers. The adventurers fought with bravado and strength under the tutelage of "+ leader + " the followers were defeated but they still suffered great losses. After a headcount of the remaining squad, "+ adventurersKilled +" were found to be dead which left only " + survivors + " remaining survivors.\n"); console.log("Current Statistics :\n");console.log("Total Adventurers = " + numberOfAdventurers);console.log("Total Killed = " +…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
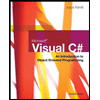
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
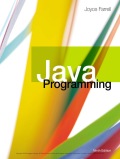
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
1.1 Arrays in Data Structure | Declaration, Initialization, Memory representation; Author: Jenny's lectures CS/IT NET&JRF;https://www.youtube.com/watch?v=AT14lCXuMKI;License: Standard YouTube License, CC-BY
Definition of Array; Author: Neso Academy;https://www.youtube.com/watch?v=55l-aZ7_F24;License: Standard Youtube License