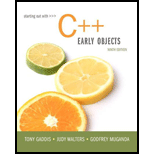
Starting Out with C++: Early Objects (9th Edition)
9th Edition
ISBN: 9780134400242
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 14, Problem 12PC
Program Plan Intro
Ancestral Trees
Program Plan:
- Include required header files.
- Declare and initialize string arrays “people []”, “mother []”, and “father []”.
- Declare and initialize positions for the integer arrays “mom []”, and “pop []”.
- Give function prototype “ancestors ()”.
- Given function definition for “ancestors ()”.
- Print the name of the person.
- Check if mom’s position is not equal to -1.
- Call the function ““ancestors ()”.
- Check if pop’s position is not equal to -1.
- Call the function ““ancestors ()”.
- Define the function “main ()”. Inside this function,
- Print the ancestors of the person containing index “0” from “people []” array by calling the function “ancestors ()” by passing “0” as the argument.
- Print the ancestors of the person containing index “6” from “people []” array by calling the function “ancestors ()” by passing “6” as the argument.
- Return the statement.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Course: Data Structure and Algorithims
Language: Java
Kindly make the program in 2 hours.
Task is well explained.
You have to make the proogram properly in Java:
Restriction: Prototype cannot be change you have to make program by using given prototype.
TAsk:
Create a class Node having two data members
int data;
Node next;
Write the parametrized constructor of the class Node which contain one parameter int value assign this value to data and assign next to null
Create class LinkList having one data members of type Node.
Node head
Write the following function in the LinkList class
publicvoidinsertAtLast(int data);//this function add node at the end of the list
publicvoid insertAthead(int data);//this function add node at the head of the list
publicvoid deleteNode(int key);//this function find a node containing "key" and delete it
publicvoid printLinkList();//this function print all the values in the Linklist
public LinkListmergeList(LinkList l1,LinkList l2);// this function…
C++ Queue LinkedList, Refer to the codes I've made as a guide and add functions where it can add/insert/remove function to get the output of the program, refer to the image as an example of input & output. Put a comment or explain how the program works/flow.
Course: Data Structure and Algorithms
Language: C++
Question is well explained
Question #2Implement a class for Circular Doubly Linked List (with a dummy header node) which stores integers in unsorted order. Your class definitions should look like as shown below:
class CDLinkedList;class DNode {friend class CDLinkedList;private int data;private DNode next;private DNode prev;};class CDLinkedList
{private:DNode head; // Dummy header nodepublic CDLinkedList(); // Default constructorpublic bool insert (int val); public bool removeSecondLastValue (); public void findMiddleValue(); public void display(); };
Chapter 14 Solutions
Starting Out with C++: Early Objects (9th Edition)
Ch. 14.1 - What is a recursive functions base case?Ch. 14.1 - What happens if a recursive function does not...Ch. 14.1 - Prob. 14.3CPCh. 14.1 - What is the difference between direct and indirect...Ch. 14 - What type of recursive function do you think would...Ch. 14 - Which repetition approach is less efficient; a...Ch. 14 - When should you choose a recursive algorithm over...Ch. 14 - Prob. 4RQECh. 14 - Prob. 5RQECh. 14 - Prob. 6RQE
Ch. 14 - Predict the Output 7. What is the output of the...Ch. 14 - Soft Skills 8. Programming is communication; the...Ch. 14 - Prob. 1PCCh. 14 - Recursive Conversion Convert the following...Ch. 14 - Prob. 3PCCh. 14 - Recursive Array Sum Write a function that accepts...Ch. 14 - Prob. 5PCCh. 14 - Recursive Member Test Write a recursive Boolean...Ch. 14 - Prob. 7PCCh. 14 - Prob. 8PCCh. 14 - Ackermanns Function Ackermanns function is a...Ch. 14 - Prefix to Postfix Write a program that reads...Ch. 14 - Prob. 11PCCh. 14 - Prob. 12PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- data structersarrow_forwardSubject-Object oriented programing Write a program which:• creates a new Array List• adds 5 decimal numbers to it• prints the list to the screen In the same program, remove the element at the last index of the Array List. Print the resulting list tothe screen.arrow_forwardint main() //default function for call { int a[100],n,i,j; for (int i = 0; i < n; i++) //Loop for ascending ordering { for (int j = 0; j < n; j++) //Loop for comparing other values { if (a[j] > a[i]) //Comparing other array elements { int tmp = a[i]; //Using temporary variable for storing last value a[i] = a[j]; //replacing value a[j] = tmp; //storing last value } } } printf("\n\nAscending : "); //Printing message for (int i = 0; i < n; i++) //Loop for printing array data after sorting { printf(" %d ", a[i]); } } Need to transform this C code to MIPS Assembly Language Code simple stepsarrow_forward
- C++ DATA STRUCTURES Implement the TNode and Tree classes. The TNode class will include a data item name of type string,which will represent a person’s name. Yes, you got it right, we are going to implement a family tree!Please note that this is not a Binary Tree. Write the methods for inserting nodes into the tree,searching for a node in the tree, and performing pre-order and post-order traversals.The insert method should take two strings as input. The second string will be added as a child node tothe parent node represented by the first string. Hint: The TNode class will need to have two TNode pointers in addition to the name data member:TNode *sibling will point to the next sibling of this node, and TNode *child will represent the first child ofthis node. You see two linked lists here??? Yes! You’ll need to use the linked listsarrow_forwardC++arrow_forwardYour colleague wrote some code that works on their local environment Debug.cpp ) but is now trying to integrate it into the main source code DataLogger.cpp ). They are getting an error and have asked you to help them fix it. COMPILE AND RUN Submit your code by pressing the button below. Write a note to your colleague about why their code does not work. Include at least two ways fix bug. Which way would/did you fix the bug? Why?arrow_forward
- a "filter" function that takes an array (int[]) and returns a new array containing only the non-negative elements of the array.arrow_forwardLanguage/Type: C++ binary trees pointers recursion Write a function named hasPath that interacts with a tree of BinaryTreeNode structures representing an unordered binary tree. The function accepts three parameters: a pointer to the root of the tree, and two integers start and end, and returns true if a path can be found in the tree from start down to end. In other words, both start and end must be element data values that are found in the tree, and end must be below start, in one of start's subtrees; otherwise the function returns false. If start and end are the same, you are simply checking whether a single node exists in the tree with that data value. If the tree is empty, your function should return false. For example, suppose a BinaryTreeNode pointer named tree points to the root of a tree storing the following elements. The table below shows the results of several various calls to your function: 67 88 52 1 21 16 99 45 Call Result Reason hasPath(tree, 67, 99) true path exists…arrow_forward*Please using JAVA only* Objective Program 3: Binary Search Tree Program The primary objective of this program is to learn to implement binary search trees and to combine their functionalities with linked lists. Program Description In a multiplayer game, players' avatars are placed in a large game scene, and each avatar has its information in the game. Write a program to manage players' information in a multiplayer game using a Binary Search (BS) tree for a multiplayer game. A node in the BS tree represents each player. Each player should have an ID number, avatar name, and stamina level. The players will be arranged in the BS tree based on their ID numbers. If there is only one player in the game scene, it is represented by one node (root) in the tree. Once another player enters the game scene, a new node will be created and inserted in the BS tree based on the player ID number. Players during the gameplay will receive hits that reduce their stamina. If the players lose…arrow_forward
- CO LL * Question Completion Status: QUESTION 3 Write a recursive function, OnlyChild(..), that returns the number of nodes in a binary tree that has only one child. Consider binaryTreeNode structure is defined as the following. struct binaryTreeNode int info; binaryTreeNode *llink: binaryTreeNode *rlink; The function is declared as the following. You must write the function as a recursive function. You will not get any credits if a non-recursive solution is used. int OnlyChild(binaryTreeNode *p); For the toolbar, press ALT+F10 (PC) or ALT+FN+F10 (Mac). Paragraph Arial 10pt B. ^三へ三 三山 三Ex? X2 = E E E 9 Click Save and Submit to save and submit. Click Save All Answers to save all ansuwers. Is E English (United States) Focus || 15 stv MacBook Air D00 O00 F4 F5 F8 64arrow_forwardC++ PROGRAMMINGTopic: Binary Search Trees Explain the c++ code below.: SEE ATTACHED PHOTO FOR THE PROBLEM INSTRUCTIONS It doesn't have to be long, as long as you explain what the important parts of the code do. (The code is already implemented and correct, only the explanation needed) node* left(node* p) { return p->left; } node* right(node* p) { return p->right; } node* sibling(node* p){ if(p != root){ node* P = p->parent; if(left(P) != NULL && right(P) != NULL){ if(left(P) == p){ return right(P); } return left(P); } } return NULL; } node* addRoot(int e) { if(size != 0){ cout<<"Error"<<endl; return NULL; } root = create_node(e,NULL); size++; return root; } node* addLeft(node* p, int e) {…arrow_forwardLanguage: JAVA Script write a function 'keyValueDuplicates (obj) that takes an object as an argument and returns an array containing all keys that are also values in that object. Examples: obj1 = { orange: "juice", apple: "sauce", sauce: "pan" }; console.log(keyValueDuplicates (obj1)); // ["sauce"] obj2 = { big: "foot", foot: "ball", ball: "boy", boy: "scout"};console.log(keyValueDuplicates(obj2)); // ["foot", "ball", "boy"] obj3 = { pizza: "pie", apple: "pie" pumpkin: "pie"); console.log(keyValueDuplicates (obj3)); // [] *******************************************************************/function keyValueDuplicates (obj) { | // Your code here } /*****DO NOT MODIFY ANYTHING UNDER THIS LINE*********/ try { | module.exports = keyValueDuplicates; } catch (e) { | module.exports = null;}arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
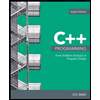
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage