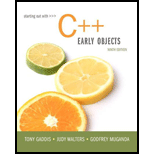
Starting Out with C++: Early Objects (9th Edition)
9th Edition
ISBN: 9780134400242
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 14, Problem 7PC
Program Plan Intro
String Reverser
- Include the required header files to the program.
- Declare function prototype.
- Define the “main()” function. Inside this function,
- Declare an array of character named “word[]”.
- Get a word from the user and store it in a variable “word”.
- Print the reversed string by calling the function “print_Reverse()”.
- Return the statement.
- Give function definition for “print_Reverse()”.
- Check if the string “s” is not null.
- Call the function “print_Reverse()” recursively.
- Print the first character in the last position.
- Check if the string “s” is not null.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Which of the following opens when you click the launcher in the Size group on the Ribbon?
Question 19Select one:
a.
Size dialog box
b.
Layout dialog box
c.
Width and Height dialog box
d.
Format dialog box
How do you resize a graphic object horizontally while keeping the center position fixed?
Question 20Select one:
a.
Drag a side sizing handle.
b.
Press [Ctrl] and drag a side sizing handle.
c.
Press [Alt] and drag a side sizing handle.
d.
Press [Shift] and drag a side sizing handle.
Which of the following indicates that a graphic is anchored to the nearest paragraph?
Question 18Select one:
a.
X and Y coordinates
b.
An anchor symbol
c.
A paragraph symbol
d.
ruler marks
Chapter 14 Solutions
Starting Out with C++: Early Objects (9th Edition)
Ch. 14.1 - What is a recursive functions base case?Ch. 14.1 - What happens if a recursive function does not...Ch. 14.1 - Prob. 14.3CPCh. 14.1 - What is the difference between direct and indirect...Ch. 14 - What type of recursive function do you think would...Ch. 14 - Which repetition approach is less efficient; a...Ch. 14 - When should you choose a recursive algorithm over...Ch. 14 - Prob. 4RQECh. 14 - Prob. 5RQECh. 14 - Prob. 6RQE
Ch. 14 - Predict the Output 7. What is the output of the...Ch. 14 - Soft Skills 8. Programming is communication; the...Ch. 14 - Prob. 1PCCh. 14 - Recursive Conversion Convert the following...Ch. 14 - Prob. 3PCCh. 14 - Recursive Array Sum Write a function that accepts...Ch. 14 - Prob. 5PCCh. 14 - Recursive Member Test Write a recursive Boolean...Ch. 14 - Prob. 7PCCh. 14 - Prob. 8PCCh. 14 - Ackermanns Function Ackermanns function is a...Ch. 14 - Prefix to Postfix Write a program that reads...Ch. 14 - Prob. 11PCCh. 14 - Prob. 12PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Which command in the Adjust group allows you to change one picture for another but retain the original picture's size and formatting? Question 17Select one: a. Change Picture b. Replace c. Swap d. Relinkarrow_forwardHow do you insert multiple rows at the same time? Question 10Select one: a. Select the number of rows you want to insert, then use an Insert Control or use the buttons on the Ribbon. b. Click Insert Multiple Rows in the Rows & Columns group. c. Select one row and click the Insert Above or Insert Below button. You will be prompted to choose how many rows to insert. d. You cannot insert multiple rows at the same time.arrow_forwardHow do you center the text vertically in each table cell? Question 9Select one: a. Select the table and click the Distribute Columns button. b. Select the table and click the Center button in the Paragraph group on the Home tab. c. Select the table and click the AutoFit button. d. Click the Select button in the Table group, click Select Table, then click the Align Center Left button in the Alignment group.arrow_forward
- A(n) ____ is a box formed by the intersection of a column and a row. Question 8Select one: a. divider b. table c. border d. cellarrow_forwardA ____ row is the first row of a table that contains the column headings. Question 7Select one: a. header b. primary c. title d. headingarrow_forwardThe Horse table has the following columns: ID - integer, auto increment, primary key RegisteredName - variable-length string Breed - variable-length string Height - decimal number BirthDate - date Delete the following rows: Horse with ID 5 All horses with breed Holsteiner or Paint All horses born before March 13, 2013 To confirm that the deletes are correct, add the SELECT * FROM HORSE; statement.arrow_forward
- Why is Linux popular? What would make someone choose a Linux OS over others? What makes a server? How is a server different from a workstation? What considerations do you have to keep in mind when choosing between physical, hybrid, or virtual server and what are the reasons to choose a virtual installation over the other options?arrow_forwardObjective you will: 1. Implement a Binary Search Tree (BST) from scratch, including the Big Five (Rule of Five) 2. Implement the TreeSort algorithm using a in-order traversal to store sorted elements in a vector. 3. Compare the performance of TreeSort with C++'s std::sort on large datasets. Part 1: Understanding TreeSort How TreeSort Works TreeSort is a comparison-based sorting algorithm that leverages a Binary Search Tree (BST): 1. Insert all elements into a BST (logically sorting them). 2. Traverse the BST in-order to extract elements in sorted order. 3. Store the sorted elements in a vector. Time Complexity Operation Average Case Worst Case (Unbalanced Tree)Insertion 0(1log n) 0 (n)Traversal (Pre-order) 0(n) 0 (n)Overall Complexity 0(n log n) 0(n^2) (degenerated tree) Note: To improve performance, you could use a…arrow_forwardI need help fixing the minor issue where the text isn't in the proper place, and to ensure that the frequency cutoff is at the right place. My code: % Define frequency range for the plot f = logspace(1, 5, 500); % Frequency range from 10 Hz to 100 kHz w = 2 * pi * f; % Angular frequency % Parameters for the filters - let's adjust these to get more reasonable cutoffs R = 1e3; % Resistance in ohms (1 kΩ) C = 1e-6; % Capacitance in farads (1 μF) % For bandpass, we need appropriate L value for desired cutoffs L = 0.1; % Inductance in henries - adjusted for better bandpass response % Calculate cutoff frequencies first to verify they're in desired range f_cutoff_RC = 1 / (2 * pi * R * C); f_resonance = 1 / (2 * pi * sqrt(L * C)); Q_factor = (1/R) * sqrt(L/C); f_lower_cutoff = f_resonance / (sqrt(1 + 1/(4*Q_factor^2)) + 1/(2*Q_factor)); f_upper_cutoff = f_resonance / (sqrt(1 + 1/(4*Q_factor^2)) - 1/(2*Q_factor)); % Transfer functions % Low-pass filter (RC) H_low = 1 ./ (1 + 1i * w *…arrow_forward
- My code is experincing minor issue where the text isn't in the proper place, and to ensure that the frequency cutoff is at the right place. My code: % Define frequency range for the plot f = logspace(1, 5, 500); % Frequency range from 10 Hz to 100 kHz w = 2 * pi * f; % Angular frequency % Parameters for the filters - let's adjust these to get more reasonable cutoffs R = 1e3; % Resistance in ohms (1 kΩ) C = 1e-6; % Capacitance in farads (1 μF) % For bandpass, we need appropriate L value for desired cutoffs L = 0.1; % Inductance in henries - adjusted for better bandpass response % Calculate cutoff frequencies first to verify they're in desired range f_cutoff_RC = 1 / (2 * pi * R * C); f_resonance = 1 / (2 * pi * sqrt(L * C)); Q_factor = (1/R) * sqrt(L/C); f_lower_cutoff = f_resonance / (sqrt(1 + 1/(4*Q_factor^2)) + 1/(2*Q_factor)); f_upper_cutoff = f_resonance / (sqrt(1 + 1/(4*Q_factor^2)) - 1/(2*Q_factor)); % Transfer functions % Low-pass filter (RC) H_low = 1 ./ (1 + 1i * w *…arrow_forwardI would like to know the main features about the following three concepts: 1. Default forwarded 2. WINS Server 3. IP Security (IPSec).arrow_forwardmap the following ER diagram into a relational database schema diagram. you should take into account all the constraints in the ER diagram. Underline the primary key of each relation, and show each foreign key as a directed arrow from the referencing attributes (s) to the referenced relation. NOTE: Need relational database schema diagramarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
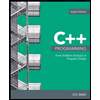
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
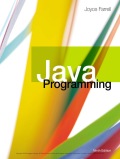
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
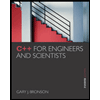
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
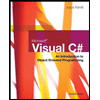
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
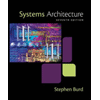
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning