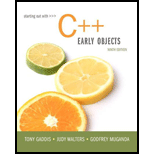
Starting Out with C++: Early Objects (9th Edition)
9th Edition
ISBN: 9780134400242
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 14, Problem 3PC
Program Plan Intro
QuickSort template
Program Plan:
“main.cpp”
- Include the required header files.
- Define the main () function.
- Declare and define the necessary variables using “for” loop.
- Prompt and get “10” array elements from the user.
- Call the method “quicksort ()” to perform the sort operation for the given elements.
- The sorted values are being displayed to the user using “for” loop.
“Quicksort.h”
- Define the necessary template function.
- Define the method definition that compare and performs sort operation for the contents of the array using quick sort.
- Define the method “swapVars ()” with two arguments,
- Assign the temporary variable and perform the necessary swap assignment statements as required.
- Define the method “partition()” with three arguments,
- Declare the necessary variables.
- Calculate the “mid” value from the list of elements.
- Call the function “swapVars ()” to perform necessary swap operations.
- Use “for” loop to iterate and validate the values using “if” condition then perform the necessary swaps by calling the function “swapVars ()”.
- Return the index of the pivot element.
- Define the method “quicksort ()”,
- Declare the variable named “pivotpt”.
- Condition statement that compares the values present at the beginning and at the end.
- Call the method “partition ()” to partition the list.
- Call the method “quicksort ()” to sort the first and second sub lists.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
C language. Function write the arraylist_sort function
This generic function sorts an array list using the given compare function.
l An array list
compare Pointer to the function which compares two elements
If you cannot write down a generic function which works for all types, write down a function which sorts resturants. If you cannot use function pointers, you can write down multiple functions which sort using different criteria.
javascript
Define a function, myIncludes, that accepts two parameters: an array and a searchValue.
myIncludes should return true if the searchValue is an element in the array. Otherwise, myIncludes should return false.
example
myIncludes([10, 20, 30], 20); // => true myIncludes(['apples', 'bananas', 'citrus'], 'pears'); // => false
Concatenate Map
This function will be given a single parameter known as the Map List. The Map List is a list of maps. Your job is to combine all the maps found in the map list into a single map and return it. There are two rules for addingvalues to the map.
You must add key-value pairs to the map in the same order they are found in the Map List. If the key already exists, it cannot be overwritten. In other words, if two or more maps have the same key, the key to be added cannot be overwritten by the subsequent maps.
Signature:
public static HashMap<String, Integer> concatenateMap(ArrayList<HashMap<String, Integer>> mapList)
Example:
INPUT: [{b=55, t=20, f=26, n=87, o=93}, {s=95, f=9, n=11, o=71}, {f=89, n=82, o=29}]OUTPUT: {b=55, s=95, t=20, f=26, n=87, o=93}
INPUT: [{v=2, f=80, z=43, k=90, n=43}, {d=41, f=98, y=39, n=83}, {d=12, v=61, y=44, n=30}]OUTPUT: {d=41, v=2, f=80, y=39, z=43, k=90, n=43}
INPUT: [{p=79, b=10, g=28, h=21, z=62}, {p=5, g=87, h=38}, {p=29,…
Chapter 14 Solutions
Starting Out with C++: Early Objects (9th Edition)
Ch. 14.1 - What is a recursive functions base case?Ch. 14.1 - What happens if a recursive function does not...Ch. 14.1 - Prob. 14.3CPCh. 14.1 - What is the difference between direct and indirect...Ch. 14 - What type of recursive function do you think would...Ch. 14 - Which repetition approach is less efficient; a...Ch. 14 - When should you choose a recursive algorithm over...Ch. 14 - Prob. 4RQECh. 14 - Prob. 5RQECh. 14 - Prob. 6RQE
Ch. 14 - Predict the Output 7. What is the output of the...Ch. 14 - Soft Skills 8. Programming is communication; the...Ch. 14 - Prob. 1PCCh. 14 - Recursive Conversion Convert the following...Ch. 14 - Prob. 3PCCh. 14 - Recursive Array Sum Write a function that accepts...Ch. 14 - Prob. 5PCCh. 14 - Recursive Member Test Write a recursive Boolean...Ch. 14 - Prob. 7PCCh. 14 - Prob. 8PCCh. 14 - Ackermanns Function Ackermanns function is a...Ch. 14 - Prefix to Postfix Write a program that reads...Ch. 14 - Prob. 11PCCh. 14 - Prob. 12PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Defintion of the code templatearrow_forwardProgramming Language: C++ I need the codes for arrayListType.h, main.cpp, myString.cpp, myString.harrow_forwardC++ Format Please Write a function (named "Lookup") that takes two const references to vectors. The first vector is a vector of strings. This vector is a list of words. The second parameter is a list of ints. Where each int denotes an index in the first vector. The function should return a string formed by concatenated the words (separated by a space) of the first vector in the order denoted by the second vector. Input of Test Case provided in PDF:arrow_forward
- Problem DNA: Subsequence markingA common task in dealing with DNA sequences is searching for particular substrings within longer DNA sequences. Write a function mark_dna that takes as parameters a DNA sequence to search, and a shorter target sequence to find within the longer sequence. Have this function return a new altered sequence that is the original sequence with all non-overlapping occurrences of the target surrounded with the characters >> and <<. Hints: ● String slicing is useful for looking at multiple characters at once. ● Remember that you cannot modify the original string directly, you’ll need to build a copy. Start with an empty string and concatenate onto it as you loop. Constraints: ● Don't use the built-in replace string method. All other string methods are permitted. >>> mark_dna('atgcgctagcatg', 'gcg') 'at>>gcg<<ctagcatg' >>> mark_dna('atgcgctagcatg', 'gc')…arrow_forwardC++ Language Please add an execution chart for this code like the example below. I have provided the code and the example execution chart. : JUST NEED EXECUTION CHARTTT. Thanks Sample Execution Chart Template//your header files// constant size of the array// declare an array of BankAccount objects called accountsArray of size =SIZE// comments for other declarations with variable names etc and their functionality// function prototypes// method to fill array from file, details of input and output values and purpose of functionvoid fillArray (ifstream &input,BankAccount accountsArray[]);// method to find the largest account using balance as keyint largest(BankAccount accountsArray[]);// method to find the smallest account using balance as keyint smallest(BankAccount accountsArray[]);// method to display all elements of the accounts arrayvoid printArray(BankAccount accountsArray[]);int main() {// function calls come here:// give the function call and a comment about the purpose,//…arrow_forwardC++ Language Please add an execution chart for this code like the example below. I have provided the code and the example execution chart. : JUST NEED EXECUTION CHARTTT. Thanks Sample Execution Chart Template//your header files// constant size of the array// declare an array of BankAccount objects called accountsArray of size =SIZE// comments for other declarations with variable names etc and their functionality// function prototypes// method to fill array from file, details of input and output values and purpose of functionvoid fillArray (ifstream &input,BankAccount accountsArray[]);// method to find the largest account using balance as keyint largest(BankAccount accountsArray[]);// method to find the smallest account using balance as keyint smallest(BankAccount accountsArray[]);// method to display all elements of the accounts arrayvoid printArray(BankAccount accountsArray[]);int main() {// function calls come here:// give the function call and a comment about the purpose,//…arrow_forward
- C++ Language Please add an execution chart for this code like the example below. I have provided the code and the example execution chart. : JUST NEED EXECUTION CHARTTT. Thanks Sample Execution Chart Template//your header files// constant size of the array// declare an array of BankAccount objects called accountsArray of size =SIZE// comments for other declarations with variable names etc and their functionality// function prototypes// method to fill array from file, details of input and output values and purpose of functionvoid fillArray (ifstream &input,BankAccount accountsArray[]);// method to find the largest account using balance as keyint largest(BankAccount accountsArray[]);// method to find the smallest account using balance as keyint smallest(BankAccount accountsArray[]);// method to display all elements of the accounts arrayvoid printArray(BankAccount accountsArray[]);int main() {// function calls come here:// give the function call and a comment about the purpose,//…arrow_forwardErlang File -module(exam). -compile(export_all). -include_lib("eunit/include/eunit.hrl"). Part A Implement a function called min which takes a tuple of two numeric values and returns the lower value of the two.Implementation notes:• If needed, use Erlang function guards• Do NOT use the if or case structuresSample calls:> exam:min({3, 4}).3> exam:min({4, 3}).3arrow_forwardCLASSES, DYNAMIC ARRAYS AND POINTERS Define a class called textLines that will be used to store a list of lines of text (each line can be specified as a string). Use a dynamic array to store the list. In addition, you should have a private data member that specifies the length of the list. Create a constructor that takes a file name as parameter, and fills up the list with lines from the file. Make sure that you set the dynamic array to expand large enough to hold all the lines from the file. Also, create a constructor that takes an integer parameter that sets the size of an empty list. in C++ Write member functions to: remove and return the last line from the list add a new line onto the end of the list, if there is room for it, otherwise print a message and expand the array empty the entire list return the number of lines still on the list take two lists and return one combined list (with no duplicates) copy constructor to support deep copying remember the destructor!arrow_forward
- Remove is a function that has been defined.arrow_forwardThe mapped list pattern Our second pattern is the mapped list pattern, described in video 4 3 mapped list pattern. Often we need to write a function that takes a list as a parameter and returns a new list in which each item in the original list is "mapped" to a new item in the result list. For example, the following function takes a list of numbers as a parameter and returns a list of all the numbers squared, e.g. squares ( [1, 3, 7]) returns [1, 9, 49]. def squares (nums): "Returns the squares of the given numbers""" result = [] for num in nums: result.append (num * num) return result Although this is just a special case of the accumulator pattern, it is so common that we give it its own name: the mapped list pattern. Consider the following function: def squares(nums): ""Returns the squares of the given numbers""" result = [] for num in nums: result.append (num * num) return result If the main program calls print(squares ( [5, -3, 2, 7]) what is the state table for the function…arrow_forwardC++arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
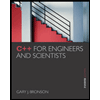
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr