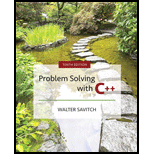
Problem Solving with C++ (10th Edition)
10th Edition
ISBN: 9780134448282
Author: Walter Savitch, Kenrick Mock
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 13.1, Problem 4STE
Program Plan Intro
Linked list:
- Linked list denotes a linear data structure.
- The elements are not stored at contiguous locations; the elements are linked using pointers.
- It stores linear data of similar types not like arrays.
- The size of linked list can be changed based on requirement.
- It is represented by a pointer to first linked list node.
- The first node denotes a head.
- If linked list is empty, value of head is NULL.
- The node in a list has two parts, data and pointer to next node.
Given code:
//Define a structure
struct ListNode
{
//Declare variable
string item;
//Declare variable
int count;
//Define next node
ListNode *link;
};
//Create instance
ListNode *head = new ListNode;
Explanation:
- The “ListNode” defines a structure with “item” and “count” as members.
- It has a pointer “link” to next element of list.
- The “ListNode *head = new ListNode” creates an instance of structure and a new element is created.
Complete program:
//Include libraries
#include<iostream>
#include<string>
//Use namespace
using namespace std;
//Define a structure
struct ListNode
{
//Declare variable
string item;
//Declare variable
int count;
//Define next node
ListNode *link;
};
//Create instance
ListNode *head = new ListNode;
//Define main method
void main()
{
//Assign value
head->item = "Wilbur's brother Orville";
//Display value
cout << head->item << endl;
//Pause console window
system("pause");
}
Explanation:
- The “ListNode” defines a structure with “item” and “count” as members.
- It contains a pointer “link” to next element of list.
- The “ListNode *head = new ListNode” creates an instance of structure and a new element is created.
- The main method assigns value to “item” to head of linked list.
- The value of head of linked list is been displayed.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
#ifndef LLCP_INT_H#define LLCP_INT_H
#include <iostream>
struct Node{ int data; Node *link;};
bool DelOddCopEven(Node* headPtr);int FindListLength(Node* headPtr);bool IsSortedUp(Node* headPtr);void InsertAsHead(Node*& headPtr, int value);void InsertAsTail(Node*& headPtr, int value);void InsertSortedUp(Node*& headPtr, int value);bool DelFirstTargetNode(Node*& headPtr, int target);bool DelNodeBefore1stMatch(Node*& headPtr, int target);void ShowAll(std::ostream& outs, Node* headPtr);void FindMinMax(Node* headPtr, int& minValue, int& maxValue);double FindAverage(Node* headPtr);void ListClear(Node*& headPtr, int noMsg = 0);
// prototype of DelOddCopEven of Assignment 5 Part 1
#endif
// definition of DelOddCopEven of Assignment 5 Part 1//Algorithm should: /*NOT destroy any of the originally even-valued node. This means that the originally even-valued nodes should be retained as part of the resulting list. Destroy…
#ifndef LLCP_INT_H#define LLCP_INT_H
#include <iostream>
struct Node{ int data; Node *link;};void DelOddCopEven(Node*& headPtr);int FindListLength(Node* headPtr);bool IsSortedUp(Node* headPtr);void InsertAsHead(Node*& headPtr, int value);void InsertAsTail(Node*& headPtr, int value);void InsertSortedUp(Node*& headPtr, int value);bool DelFirstTargetNode(Node*& headPtr, int target);bool DelNodeBefore1stMatch(Node*& headPtr, int target);void ShowAll(std::ostream& outs, Node* headPtr);void FindMinMax(Node* headPtr, int& minValue, int& maxValue);double FindAverage(Node* headPtr);void ListClear(Node*& headPtr, int noMsg = 0);
// prototype of DelOddCopEven of Assignment 5 Part 1
#endif
this is code
#include <iostream> using namespace std; struct Node { int data; struct Node *next; }; struct Node* head = NULL; void insert(int new_data) { struct Node* new_node = (struct Node*) malloc(sizeof(struct Node)); new_node->data = new_data; new_node->next = head; head = new_node; } void display() { struct Node* ptr; ptr = head; while (ptr != NULL) { cout<< ptr->data <<" "; ptr = ptr->next; } } int main() { insert(3); insert(1); insert(7); insert(2); insert(9); cout<<"The linked list is: "; display(); return 0; }
i want
Re-implement it on doubly linked list take in account the set-position method and the operator overload and coy constructor and destructor ? in c++
Chapter 13 Solutions
Problem Solving with C++ (10th Edition)
Ch. 13.1 - Suppose your program contains the following type...Ch. 13.1 - Suppose that your program contains the type...Ch. 13.1 - Prob. 3STECh. 13.1 - Prob. 4STECh. 13.1 - Prob. 5STECh. 13.1 - Prob. 6STECh. 13.1 - Prob. 7STECh. 13.1 - Suppose your program contains type definitions and...Ch. 13.1 - Prob. 9STECh. 13.2 - Prob. 10STE
Ch. 13.2 - Prob. 11STECh. 13.2 - Prob. 12STECh. 13.2 - Prob. 13STECh. 13 - The following program creates a linked list with...Ch. 13 - Re-do Practice Program 1, but instead of a struct,...Ch. 13 - Write a void function that takes a linked list of...Ch. 13 - Write a function called mergeLists that takes two...Ch. 13 - In this project you will redo Programming Project...Ch. 13 - A harder version of Programming Project 4 would be...Ch. 13 - Prob. 6PPCh. 13 - Prob. 8PPCh. 13 - Prob. 9PPCh. 13 - Prob. 10PP
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- prg-1 #include <iostream>using namespace std; struct Node { int data; Node* left; Node* right;}; Node* newNode(int data) { Node* node = new Node; node->data = data; node->left = NULL; node->right = NULL; return node;} void printInorder(Node* node) { if (node == NULL) return; printInorder(node->left); cout << node->data << " "; printInorder(node->right);} void printPreorder(Node* node) { if (node == NULL) return; cout << node->data << " "; printPreorder(node->left); printPreorder(node->right);} void printPostorder(Node* node) { if (node == NULL) return; printPostorder(node->left); printPostorder(node->right); cout << node->data << " ";} int main() { Node* root = newNode(70); root->left = newNode(60); root->left->left = newNode(58); root->left->right = newNode(62); root->left->left->left =…arrow_forwardQuestion 9 #include using namespace std; struct ListNode { string data; ListNode *next; }; int main() { ListNode *ptr, *list; list = new ListNode; list->data = "New York"; ptr = new ListNode; ptr->data = "Boston"; list->next = ptr; ptr->next = new ListNode; ptr->next->data = "Houston"; ptr->next->next = nullptr; // new code goes here Which of the following code correctly deletes the last node of the list when added at point of insertion indicated above? O delete ptr->next; ptr->next = nullptr;; O ptr = list; while (ptr != nullptr) ptr = ptr->next; delete ptr; O ptr = last; delete ptr; list->next->next = nullptr; delete ptr; O None of thesearrow_forwardC++: Consider the following code: struct node { int data; node *next; }; node myNode; node *p = &myNode; Which of these will set member variable data of myNode to the value 7? A) node->data = 7; B) p->node = 7; C) p.node = 7; D) myNode.data = 7;arrow_forward
- In C, using malloc to allocate memory for a linked list uses which memory allocation scheme? Heap allocation Static allocationarrow_forward#include <conio.h>#include <iostream>#include <string> using namespace std;bool check = true;struct node {char name[20];char jobtitle[20];int empno;char branch;node *next;}*head,*lastptr; // pointes void add() // calling {node *p; //*p pomiterp=new node;cout<<"Enter name of employ:"<<endl;gets(p->name);cout<<"Enter job title of employ:"<<endl;gets(p->jobtitle);cout<<"Enter Number of employ:"<<endl;cin>>p->empno;cout<<"Enter branch of employ:"<<endl;cin>>p->branch;p->next=NULL; if(check) //if check is true its means heads is empty and there is no node exist.{head = p;lastptr = p;check = false;}else{lastptr->next=p;lastptr=p;}cout<<endl<<"Recored Entered";} void modify() {node *ptr; node *prev=NULL;node *current=NULL;int e_no;cout<<"Enter Number to…arrow_forward#include<stdio.h> #include<stdlib.h> #include<conio.h> struct nodetype { struct nodetype *left ; int info ; struct nodetype *right; }; typedef struct nodetype *NODEPTR; NODEPTR maketree(int); NODEPTR getnode(void); void inordtrav(NODEPTR); int main(void) { NODEPTR root , p , q; printf("%s\n","Enter First number"); scanf("%d",&number); root=maketree(number); /* insert first root item */ while(scanf("%d",&number) !=EOF) { p=q=root; /* find insertion point */ while((number !=p->info) && q!=NULL) {p=q; if (number <p->info) q = p->left; else q = p->right; } q=maketree(number); /* insertion */ if (number==p->info) printf("%d is a duplicate \n",number); else if (number<p->info)…arrow_forward
- struct BookInfo string title; string author; string publisher; double price; Booklnfo booklist[20]; Fill in the table below: ZARLE List What is structure name? Answer: How nany members? How many element? Write a code (one line only) to access the title member of booklist[5]: BOURCE CODE 1.arrow_forward//impl.h#include <string>#include <stack>#include <iostream>using namespace std;class VideoGame{public:string title, genre, publisher;int year;VideoGame(string t, string p, string g, int y){set_title(t);set_genre(g);set_publisher(p);set_year(y);}void set_title(string t){title = t;}void set_genre(string g){genre = g;}void set_publisher(string p){publisher = p;}void set_year(int y){year = y;}string get_publisher(){return publisher;}string get_title(){return title;}string get_genre(){return genre;}int get_year(){return year;}};//impl.cpp#include "impl.h"void createstack(stack <VideoGame> s){while (!s.empty()){VideoGame v = s.top();cout << "TITLE : " << v.get_title() << endl;cout << "YEAR : " << v.get_year() << endl;cout << "GENRE : " << v.get_genre() << endl;cout << "PUBLISHER : " << v.get_publisher() << endl;cout << '\n';cout << "------------------------" <<…arrow_forward#include <bits/stdc++.h>#include<unordered_map>#include <string> using namespace std; struct Student{string firstName;string lastName;string studentId;double gpa;}; class MyClass{public:unordered_map<string, int>classHoursInfo;vector<Student> students;unordered_map<string, int> creditValue = {{"A", 4}, {"B", 3}, {"C", 2}, {"D", 1}, {"F", 0}}; void readStudentData(string filepath){cout << "reading student data \n";ifstream inpStream(filepath);string text;string tmp[4];while (getline (inpStream, text)) {string str = "", prevKey = ""; int j= 0;unordered_map<string, int> curStudentClassInfo;for(int i=0; i<text.length(); i++){if(isspace(text[i])){if(j>3){if(prevKey.length()==0){curStudentClassInfo[str] = 0;prevKey = str;str.clear();}else{curStudentClassInfo[prevKey] = creditValue[str];prevKey = "";str.clear();}}else{tmp[j++] = str;str.clear();}}else{str = str+text[i];}}if(str.length() != 0){curStudentClassInfo[prevKey] =…arrow_forward
- #include <stdio.h>#include <stdlib.h>#include <time.h> struct treeNode { struct treeNode* leftPtr; int data; struct treeNode* rightPtr;}; typedef struct treeNode TreeNode;typedef TreeNode* TreeNodePtr; void insertNode(TreeNodePtr* treePtr, int value);void inOrder(TreeNodePtr treePtr);void preOrder(TreeNodePtr treePtr);void postOrder(TreeNodePtr treePtr); int main(void) { TreeNodePtr rootPtr = NULL; srand(time(NULL)); puts("The numbers being placed in the tree are:"); for (unsigned int i = 1; i <= 10; ++i) { int item = rand() % 15; printf("%3d", item); insertNode(&rootPtr, item); } puts("\n\nThe preOrder traversal is: "); preOrder(rootPtr); puts("\n\nThe inOrder traversal is: "); inOrder(rootPtr); puts("\n\nThe postOrder traversal is: "); postOrder(rootPtr);} void insertNode(TreeNodePtr* treePtr, int value) { if (*treePtr == NULL) { *treePtr = malloc(sizeof(TreeNode)); if…arrow_forward#include <stdio.h>#include <stdlib.h>#include <time.h> struct treeNode { struct treeNode *leftPtr; int data; struct treeNode *rightPtr;}; typedef struct treeNode TreeNode;typedef TreeNode *TreeNodePtr; void insertNode(TreeNodePtr *treePtr, int value);void inOrder(TreeNodePtr treePtr);void preOrder(TreeNodePtr treePtr);void postOrder(TreeNodePtr treePtr); int main(void) { TreeNodePtr rootPtr = NULL; srand(time(NULL)); puts("The numbers being placed in the tree are:"); for (unsigned int i = 1; i <= 10; ++i) { int item = rand() % 15; printf("%3d", item); insertNode(&rootPtr, item); } puts("\n\nThe preOrder traversal is: "); preOrder(rootPtr); puts("\n\nThe inOrder traversal is: "); inOrder(rootPtr); puts("\n\nThe postOrder traversal is: "); postOrder(rootPtr);} void insertNode(TreeNodePtr *treePtr, int value) { if (*treePtr == NULL) { *treePtr = malloc(sizeof(TreeNode)); if (*treePtr != NULL) { (*treePtr)->data = value;…arrow_forwardC++ Given code #include <iostream>using namespace std; class Node {public:int data;Node *pNext;}; void displayNumberValues( Node *pHead){while( pHead != NULL) {cout << pHead->data << " ";pHead = pHead->pNext;}cout << endl;} //Option 1: Search the list// TODO: complete the function below to search for a given value in linked lsit// return true if value exists in the list, return false otherwise. ?? linkedlistSearch( ???){ } //Option 2: get sum of all values// TODO: complete the function below to return the sum of all elements in the linked list. ??? getSumOfAllNumbers( ???){ } int main(){int userInput;Node *pHead = NULL;Node *pTemp;cout<<"Enter list numbers separated by space, followed by -1: "; cin >> userInput;// Keep looping until end of input flag of -1 is givenwhile( userInput != -1) {// Store this number on the listpTemp = new Node;pTemp->data = userInput;pTemp->pNext = pHead;pHead = pTemp;cin >> userInput;}cout <<"…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
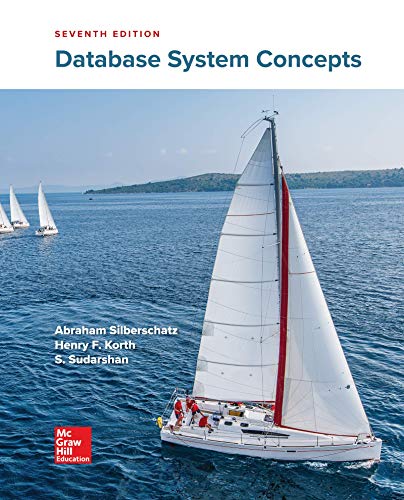
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
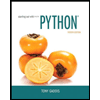
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
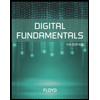
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
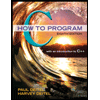
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
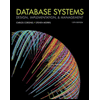
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
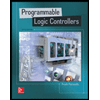
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education