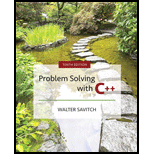
Concept explainers
Linked list:
- Linked list denotes a linear data structure.
- The elements are not stored at contiguous locations; the elements are linked using pointers.
- It stores linear data of similar types not like arrays.
- The size of linked list can be changed based on requirement.
- It is represented by a pointer to first linked list node.
- The first node denotes a head.
- If linked list is empty, value of head is NULL.
- The node in a list has two parts, data and pointer to next node.
Given code:
//Define a structure
struct Box
{
//Declare variable
string name;
//Declare variable
int number;
//Define pointer to next
Box *next;
};
//Create an instance
BoxPtr head;
//Assign value
head = new Box;
//Assign value
head->name = "Sally";
//Assign value
head->number = 18;
//Assign value
head->next = NULL;
//Display value
cout << (*head).name << endl;
//Display value
cout << head->name << endl;
//Display value
cout << (*head).number << endl;
//Display value
cout << head->number << endl;
Explanation:
- The “Box” defines a structure with “name” and “number” as members.
- It contains a pointer to next element of list.
- The “BoxPtr head” creates an instance of structure.
- A new “Box” element is created.
- The values of “name” and “number” are been set.
- The values of “name” and “number” are been displayed.
- The member variable next to the node is been set to NULL.
Complete program:
//Include libraries
#include<iostream>
#include<string>
//Use namespace
using namespace std;
//Define a structure
struct Box
{
//Declare variable
string name;
//Declare variable
int number;
//Define pointer to next
Box *next;
};
//Define instance
typedef Box* BoxPtr;
//Define main method
void main()
{
//Create an instance
BoxPtr head;
//Assign value
head = new Box;
//Assign value
head->name = "Sally";
//Assign value
head->number = 18;
//Display value
cout << (*head).name << endl;
//Display value
cout << head->name << endl;
//Display value
cout << (*head).number << endl;
//Display value
cout << head->number << endl;
//Delete
delete head;
//Display message
cout<<"List head is been destroyed"<<endl;
//Pause console window
system("pause");
}
Explanation:
- The “Box” defines a structure with “name” and “number” as members.
- It contains a pointer to next element of list.
- Define a main method
- The “BoxPtr head” creates an instance of structure.
- A new “Box” element is created.
- The values of “name” and “number” are been set.
- The values of “name” and “number” are been displayed.
- The member variable next to the node is been set to NULL.
- The linked list is been destroyed using “delete” statement.

Want to see the full answer?
Check out a sample textbook solution
Chapter 13 Solutions
Problem Solving with C++ (10th Edition)
- Python - need help creating a python program that will sum the digits of a number entered by the user. For example if the user inputs the value 243 the program will output 9 because 2 + 4 + 3 = 9. The program should ask for a single integer from the user, it should then calculate the sum of all the digits of that number and output the result.arrow_forwardI need help with this in Python (with flowchart): Im creating a reverse guessing game. Then to choose a random number from 1 to 100 and the computer program will attempt to guess it, displaying the directions calculated or not. The guess will be displayed and the user will answer if it was correct or not. If correct, the game ends, if not then the computer asks if the guess was too high or too low. Finally inputting an answer and the computer generates a new guess within the proper range. Oh and to make sure the program doesnt guess outside of the ranges produced by the inputs of “too high” and “too low”. The program ending when the user guesses correctly or after the program takes 6 guesses. HELP ASAP!arrow_forwardI need help with this in Python (with flowchart): Im creating a reverse guessing game. Then to choose a random number from 1 to 100 and the computer program will attempt to guess it, displaying the directions calculated or not. The guess will be displayed and the user will answer if it was correct or not. If correct, the game ends, if not then the computer asks if the guess was too high or too low. Finally inputting an answer and the computer generates a new guess within the proper range. Oh and to make sure the program doesnt guess outside of the ranges produced by the inputs of “too high” and “too low”. The program ending when the user guesses correctly or after the program takes 6 guesses. HELP ASAP!arrow_forward
- Need help finding errors in my pseudocode (Two)! Declare Boolean finished = False Declare Integer value, cube While NOT finished Display "Enter a value to be cubed." Input value; Set cube = value^3 Display value, " cubed is ", cube End While Next, I intended the following pseudocode to display the numbers 1 through 60, and then display the message "Time’s up!". Doesnt work and has error. Declare Integer counter = 1 Const Integer TIME_LIMIT = 60 While counter < TIME_LIMIT Display counter Set counter = counter + 1 End While Display "Time's up!"arrow_forwardHaving error in pseudcode; wanting to get five sets of two numbers each, calculate the sum of each set, and calculate the sum of all the numbers entered. Not functioning as intended and can't find the error.Code: // This program calculates the sum of five sets of two numbers. Declare Integer number, sum, total Declare Integer sets, numbers Constant Integer MAX_SETS = 5 Constant Integer MAX_NUMBERS = 2 Set sum = 0; Set total = 0; For sets = 1 To MAX_NUMBERS For numbers = 1 To MAX_SETS Display "Enter number ", numbers, " of set ", sets, "." Input number; Set sum = sum + number End For Display "The sum of set ", sets, " is ", sum "." Set total = total + sum Set sum = 0 End For Display "The total of all the sets is ", total, "."arrow_forwardNeed help converting loops!1. Convert the following While loop to a For loop: Declare Integer count = 0 While count < 50 Display "The count is ", count Set count = count + 1 End While _________________________________________________ 2. Convert the following For loop to a While loop: Declare Integer count For count = 1 To 50 Display count End Forarrow_forward
- Need help making this!1.Design a nested loop that displays 10 rows of # characters. There should be 15 # characters in each row. 2. Design a nested set of for loops that displays the following arrangements of ‘X’ characters X XX XXX XXXX XXXXX XXXXXXarrow_forwardI need help to resolve the case, thank youarrow_forwardIn 32-bit MSAM, You were given the following negative array. write a program that converts each array element to its positive representation. Then add all these array elements and assign them to the dl register. .data myarr sbyte -5, -6, -7, -4.code ; Write the rest of the program and paste the fully working code in the space below. the dl register should have the value 22 after summing up all elements in the array.arrow_forward
- Microprocessor 8085 Lab Experiment Experiment No. 3 Logical Instructions Write programs with effects 1. B=(2Dh XOR D/2) - (E AND 2Eh+1) when E=53, D=1Dh 2. HL= (BC+HL) XOR DE (use register pair when necessary), when BC=247, HL 516, DE 12Ach 3. Reset bits 1,4,6 of A and set bits 3,5 when A=03BH Write all as table (address line.hexacode,opcede,operant.comment with flags)arrow_forwardIn 32-bit MASM, Assume your grocery store sells three types of fruits. Apples, Oranges, and Mangos. Following are the sale numbers for the week (7 days).dataapples dword 42, 47, 52, 63, 74, 34, 73oranges dword 78, 53, 86, 26, 46, 51, 60mangos dword 30, 39, 41, 70, 75, 84, 29Using a single LOOP instruction, write a program to add elements in all these three arrays. Then assign the total result into the eax register. The eax register should have the value 1153 after a successful execution.arrow_forwardYou were given the following negative array. write a program that converts each array element to its positive representation. Then add all these array elements and assign them to the dl register. .data myarr sbyte -5, -6, -7, -4.code ; Write the rest of the program and paste the fully working code in the space below. The dl register should have the value 22 after summing up all elements in the array. Your answer must be in 32-bit MSAM.arrow_forward
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
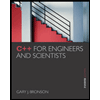
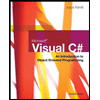
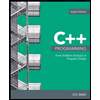
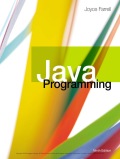
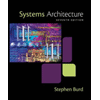