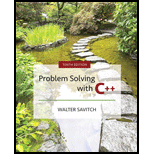
Problem Solving with C++ (10th Edition)
10th Edition
ISBN: 9780134448282
Author: Walter Savitch, Kenrick Mock
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 13, Problem 2P
Re-do Practice
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Provide me complete and correct solution thanks 3
For this assignment, you need to implement link-based List and derivative ADTs in Java. To complete this, you will need the following:
A LinkNode structure or class which will have two attributes - a data attribute and a pointer attribute to the next node. The data attribute of the LinkNode should be the Money class of Lab 1.
A Singly Linked List class which will be composed of three attributes - a count attribute, a LinkedNode pointer/reference attribute pointing to the start of the list and a LinkedNode pointer/reference attribute pointing to the end of the list. Since this is a class, make sure all these attributes are private.
The attribute names for the Node and Linked List are the words in bold in #1 and #2.
For the Linked List, implement the most common linked-list behaviors as explained in class -
getters/setters/constructors/destructors for the attributes of the class,
(a) create new list, (b) add data, (c) delete data, (d) find data, (e) count of data items in the…
Write java code for a member function insertSorted(int d) for a linked list. The
function traverses the list until it finds the correct location, then it inserts a node in that location.
You may use the function given the class.
Chapter 13 Solutions
Problem Solving with C++ (10th Edition)
Ch. 13.1 - Suppose your program contains the following type...Ch. 13.1 - Suppose that your program contains the type...Ch. 13.1 - Prob. 3STECh. 13.1 - Prob. 4STECh. 13.1 - Prob. 5STECh. 13.1 - Prob. 6STECh. 13.1 - Prob. 7STECh. 13.1 - Suppose your program contains type definitions and...Ch. 13.1 - Prob. 9STECh. 13.2 - Prob. 10STE
Ch. 13.2 - Prob. 11STECh. 13.2 - Prob. 12STECh. 13.2 - Prob. 13STECh. 13 - The following program creates a linked list with...Ch. 13 - Re-do Practice Program 1, but instead of a struct,...Ch. 13 - Write a void function that takes a linked list of...Ch. 13 - Write a function called mergeLists that takes two...Ch. 13 - In this project you will redo Programming Project...Ch. 13 - A harder version of Programming Project 4 would be...Ch. 13 - Prob. 6PPCh. 13 - Prob. 8PPCh. 13 - Prob. 9PPCh. 13 - Prob. 10PP
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
This is the process of converting an object to a series of bytes that represent the objects data. a. serializat...
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
This optional Google account security feature sends you a message with a code that you must enter, in addition ...
SURVEY OF OPERATING SYSTEMS
The current source in the circuit shown generates the current pulse
Find (a) v (0); (b) the instant of time gr...
Electric Circuits. (11th Edition)
When you open a file for the purpose of saving a pickled object to it, what file access mode do you use?
Starting Out with Python (4th Edition)
The following data have been obtained for machining a Si-AI alloy: Compute the C and n values for the Taylor to...
Degarmo's Materials And Processes In Manufacturing
Determine the maximum force P that can be applied without causing movement of the 250-lb crate that has a cente...
INTERNATIONAL EDITION---Engineering Mechanics: Statics, 14th edition (SI unit)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Java - Write a non-member method for a "enqueue" that utilizes a doubly linked list. There are three variables available (can be sent) to the method; a “front” reference, a “rear” reference, and a data element (string) (you decide what you need). Empty case is when front and rear are NULL. The node is defined as follows: class node{ node after; node before string data }arrow_forwardConcatenate Map This function will be given a single parameter known as the Map List. The Map List is a list of maps. Your job is to combine all the maps found in the map list into a single map and return it. There are two rules for addingvalues to the map. You must add key-value pairs to the map in the same order they are found in the Map List. If the key already exists, it cannot be overwritten. In other words, if two or more maps have the same key, the key to be added cannot be overwritten by the subsequent maps. Signature: public static HashMap<String, Integer> concatenateMap(ArrayList<HashMap<String, Integer>> mapList) Example: INPUT: [{b=55, t=20, f=26, n=87, o=93}, {s=95, f=9, n=11, o=71}, {f=89, n=82, o=29}]OUTPUT: {b=55, s=95, t=20, f=26, n=87, o=93} INPUT: [{v=2, f=80, z=43, k=90, n=43}, {d=41, f=98, y=39, n=83}, {d=12, v=61, y=44, n=30}]OUTPUT: {d=41, v=2, f=80, y=39, z=43, k=90, n=43} INPUT: [{p=79, b=10, g=28, h=21, z=62}, {p=5, g=87, h=38}, {p=29,…arrow_forwardAdd the following functions and write a program to test these functions in the class linkedListType: a. Write the definition of a function that returns the data of the kth element of the linked list. If such element is not exist in the list, exit the program. b. Write the definition of a function that deletes the kth element of the linked list. If such element does not exist in the list, exit the program and display message as output.arrow_forward
- Create a node class named LinkedNodes that uses up to 4 dynamic pointers to connect it to the other 8 nodes in the structure (a total of 9). The data in each node will be a unique integer number from 1 through 9. Include in your class definition these functions at a minimum: getVal(), setVal(), getNext(), setNext(), getPrev(), setPrev(), constructor(s) and destructor. Write a simple program to load these nodes into a linked structure. Do not use an array, the pointers will do that for you. You should be able to reach any node from any other node in the structure by traversing a maximum of 2 nodes from the current one. The output from your program should report the traversals between all nodes in the structure, starting with the node whose value is one. (See the example below) Node 1 2 3 4 5 6 7 8 9 -> Traverses to Node 1 Node 2 1->2 2->6->1 3->6->1 3->7->2 4->6->1 4->7->2 4->8->3 5->6->1 5->7->2 5->8->3 6->1->2 6->1->3 7->1->3 8->3 6->1 7->1 8->1 9->1 Node 3 1->3 2->3 7->2 8->2 9->2…arrow_forwardTo finish up the definition of the Node class, we need at least two constructor methods. We definitely want a default constructor that creates an emptyNode, with both the Element and Link members set to null. We also need aparameterized constructor that assigns data to the Element member and setsthe Link member to null.Write the code for the Node class:arrow_forwardPlease help me to finish this code in pythonarrow_forward
- typedef struct node_t node_t; struct node_t { }; int32_t value; node_t* next; node_t* insert (node_t* list, int32_t v, int32_t* flag); 3) As you may have noticed from MT1, Howie is lazy. He wants you to write the interface for the following implementation of function copy_to_linked_list. He really appreciates your help! /* copy_to_linked_list int32_t copy_to_linked_list (int32_t a[], int32_t size, node_t** list_p) { int32_t flag, i; for (i = 0; i < size; ++i) { *list_p = insert (*list_p, a[i], &flag); if (-1== flag) return -1; } return 0;arrow_forwardTour.java Create a Tour data type that represents the sequence of points visited in a TSP tour. Represent the tour as a circular linked list of nodes, one for each point in the tour. Each Node contains two references: one to the associated Point and the other to the next Node in the tour. Each constructor must take constant time. All instance methods must take time linear (or better) in the number of points currently in the tour. To represent a node, within Tour.java, define a nested class Node: private class Node { private Point p; private Node next; } Your Tour data type must implement the following API. You must not add public methods to the API; however, you may add private instance variables or methods (which are only accessible in the class in which they are declared). public class Tour // Creates an empty tour. public Tour() // Creates the 4-point tour a→b→c→d→a (for debugging). public Tour(Point a, Point b, Point c, Point d) // Returns the number of points in this tour. public…arrow_forwardComputer science JAVA programming languagearrow_forward
- Every time you write a non-const member function for a linked list, you should always think about if that function is preserving your class invariants. Group of answer choices A. True B. Falsearrow_forwardThis is hard help please its from my study guide in C++ LEVEL 2arrow_forwardPlease just use main.cpp,sequence.cpp and sequence.h. It has provided the sample insert()function. No documentation (commenting) is required for this assignment. This assignment is based on an assignment from "Data Structures and Other Objects Using C++" by Michael Main and Walter Savitch. Use linked lists to implement a Sequence class that stores int values. Specification The specification of the class is below. There are 9 member functions (not counting the big 3) and 2 types to define. The idea behind this class is that there will be an internal iterator, i.e., an iterator that the client cannot access, but that the class itself manages. For example, if we have a Sequence object named s, we would use s.start() to set the iterator to the beginning of the list, and s.advance() to move the iterator to the next node in the list. (I'm making the analogy to iterators as a way to help you understand the point of what we are doing. If trying to think in terms of iterators is confusing,…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
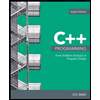
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Introduction to Linked List; Author: Neso Academy;https://www.youtube.com/watch?v=R9PTBwOzceo;License: Standard YouTube License, CC-BY
Linked list | Single, Double & Circular | Data Structures | Lec-23 | Bhanu Priya; Author: Education 4u;https://www.youtube.com/watch?v=IiL_wwFIuaA;License: Standard Youtube License