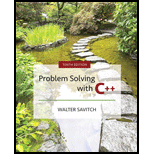
The following
#include <iostream>
#include <string>
using namespace std;
struct Node
{
string name;
Node *link;
};
typedef Node* NodePtr;
int main()
{
NodePtr listPtr, tempPtr;
listPtr = new Node;
listPtr–>name = “Emily”;
tempPtr = new Node;
tempPtr–>name = “James”;
listPtr–>link = tempPtr;
tempPtr–>link = new Node;
tempPtr = tempPtr–>link;
tempPtr–>name = “Joules”;
tempPtr–>link = NULL;
return 0;
}
Add code to the main function that:
- a. Outputs in order all names in the list.
- b. Inserts the name “Joshua” in the list after “James” then outputs the modified list.
- c. Deletes the node with “Joules” then outputs the modified list,
- d. Deletes all nodes in the list.

Creation of program to produce a linked list and perform operations
Program Plan:
- Define a structure “Node” with member variable and link to another node.
- Define a method “displayList()” to display nodes present in the list.
- Perform a loop operation until list reaches null.
- Display name of node and move to next node.
- Continue this process until list reaches null.
- Define a method “insertNameAfter()” to insert a name after a particular name.
- Perform a loop operation until list reaches null.
- Compare each word with given name.
- If required name is reached, insert new name after the given name.
- Update the next node pointer.
- Define a method “deleteNameList()” to delete a particular name in list.
- Perform a loop operation until list reaches null.
- Compare each word with given name.
- If required name is reached, delete name.
- Update the next node pointer.
- Define a method “deleteAllNodes()” to delete all nodes in list.
- Declare variables that are required for program.
- Perform a loop operation until list reaches null.
- Assign “head” to a temporary variable.
- Move to next node.
- Delete the “head” of linked list.
- Define a main method to perform operations on list.
- Declare variables that are required for program.
- Define nodes and assign values.
- Call method “displayList()” to display nodes in list.
- Call method “insertNameAfter()” to insert a name after a particular name.
- Call method “deleteNameList()” to delete a particular name in list.
- Call method “deleteAllNodes()” to delete all nodes in list.
Program Description:
The following C++ program describes about creation of program to create a linked list and perform operations on list.
Explanation of Solution
Program:
//Include libraries
#include <iostream>
#include <string>
//Use namespace
using namespace std;
//Define a structure
struct Node
{
//Declare member variable
string name;
//Declare link
Node *link;
};
//Define instance
typedef Node* NodePtr;
//Define a method displayList()
void displayList(NodePtr head)
{
//Loop until empty
while(head!=NULL)
{
//Display value
cout<<head->name<<" ";
//Move to next node
head=head->link;
}
//New line
cout<<endl;
}
//Define method insertNameAfter()
void insertNameAfter(NodePtr head,string sName, string newName)
{
//Declare variable
NodePtr temp;
//Create instance of node
temp=new Node;
//Assign value
temp->name=newName;
//Assign null value
temp->link=NULL;
//Loop until it reaches null
while(head!=NULL)
{
//If condition satisfies
if(head->name.compare(sName)==0)
{
//Assign value
temp->link=head->link;
//Assign value
head->link=temp;
//Break
break;
}
//Assign value
head=head->link;
}
}
//Define method deleteNameList()
void deleteNameList(NodePtr head,string sName)
{
//Declare variable
NodePtr prev=NULL;
//Loop until it reaches null
while(head!=NULL)
{
//If condition satisfies
if(head->name.compare(sName)==0)
{
//Assign value
prev->link=head->link;
//Delete
delete head;
//Break
break;
}
//Assign value
prev=head;
//Assign value
head=head->link;
}
}
//Define method deleteAllNodes()
void deleteAllNodes(NodePtr head)
{
//Declare variable
NodePtr temp;
//Loop
while(head)
{
//Assign value
temp=head;
//Move to next value
head=head->link;
//Delete node
delete temp;
}
}
//Define main method
int main()
{
//Declare variables
NodePtr listPtr,tempPtr;
//Create new instance
listPtr =new Node;
//Assign value
listPtr->name="Emily";
//Create new node
tempPtr=new Node;
//Assign value
tempPtr->name="James";
//Assign value
listPtr->link=tempPtr;
//Create new node
tempPtr->link=new Node;
//Move to next value
tempPtr=tempPtr->link;
//Assign value
tempPtr->name="Joules";
//Assign null value
tempPtr->link=NULL;
//Display message
cout<<"All Names in the list are: "<<endl;
//Call method displayList()
displayList(listPtr);
//Call method insertNameAfter()
insertNameAfter(listPtr,"James","Joshua");
//Display message
cout<<"Output modified list after Insert Joshua are:"<<endl;
//Call method displayList()
displayList(listPtr);
//Call method deleteNameList()
deleteNameList(listPtr,"Joules");
//Display message
cout<<"Output modified list after deleting Joshua are:"<<endl;
//Call method displayList()
displayList(listPtr);
//Call method deleteAllNodes()
deleteAllNodes(listPtr);
//Pause console window
system("pause");
//Return
return 0;
}
All Names in the list are:
Emily James Joules
Output modified list after Insert Joshua are:
Emily James Joshua Joules
Output modified list after deleting Joshua are:
Emily James Joshua
Press any key to continue . . .
Want to see more full solutions like this?
Chapter 13 Solutions
Problem Solving with C++ (10th Edition)
Additional Engineering Textbook Solutions
Starting Out with Java: Early Objects (6th Edition)
Starting Out With Visual Basic (8th Edition)
Starting Out With Visual Basic (7th Edition)
Introduction To Programming Using Visual Basic (11th Edition)
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Starting Out with C++: Early Objects (9th Edition)
- 73% void showLinked() Linked_List "p; p=List; while(pl=NULL) { coutname agephoneN0ID_NOnext; cout>k; for(int i=0;i>nam; couts>ag: cout>ph: couts>id; InserFront(nam,ag.ph,id); coutsd; for (int i=0;iarrow_forwardIn C, using malloc to allocate memory for a linked list uses which memory allocation scheme? Heap allocation Static allocationarrow_forwardQuestion 9 #include using namespace std; struct ListNode { string data; ListNode *next; }; int main() { ListNode *ptr, *list; list = new ListNode; list->data = "New York"; ptr = new ListNode; ptr->data = "Boston"; list->next = ptr; ptr->next = new ListNode; ptr->next->data = "Houston"; ptr->next->next = nullptr; // new code goes here Which of the following code correctly deletes the last node of the list when added at point of insertion indicated above? O delete ptr->next; ptr->next = nullptr;; O ptr = list; while (ptr != nullptr) ptr = ptr->next; delete ptr; O ptr = last; delete ptr; list->next->next = nullptr; delete ptr; O None of thesearrow_forwardC++ Given code #include <iostream>using namespace std; class Node {public:int data;Node *pNext;}; void displayNumberValues( Node *pHead){while( pHead != NULL) {cout << pHead->data << " ";pHead = pHead->pNext;}cout << endl;} //Option 1: Search the list// TODO: complete the function below to search for a given value in linked lsit// return true if value exists in the list, return false otherwise. ?? linkedlistSearch( ???){ } //Option 2: get sum of all values// TODO: complete the function below to return the sum of all elements in the linked list. ??? getSumOfAllNumbers( ???){ } int main(){int userInput;Node *pHead = NULL;Node *pTemp;cout<<"Enter list numbers separated by space, followed by -1: "; cin >> userInput;// Keep looping until end of input flag of -1 is givenwhile( userInput != -1) {// Store this number on the listpTemp = new Node;pTemp->data = userInput;pTemp->pNext = pHead;pHead = pTemp;cin >> userInput;}cout <<"…arrow_forwardmain.cc file #include <iostream>#include <memory> #include "customer.h" int main() { // Creates a line of customers with Adele at the front. // LinkedList diagram: // Adele -> Kehlani -> Giveon -> Drake -> Ruel std::shared_ptr<Customer> ruel = std::make_shared<Customer>("Ruel", 5, nullptr); std::shared_ptr<Customer> drake = std::make_shared<Customer>("Drake", 8, ruel); std::shared_ptr<Customer> giveon = std::make_shared<Customer>("Giveon", 2, drake); std::shared_ptr<Customer> kehlani = std::make_shared<Customer>("Kehlani", 15, giveon); std::shared_ptr<Customer> adele = std::make_shared<Customer>("Adele", 4, kehlani); std::cout << "Total customers waiting: "; // =================== YOUR CODE HERE =================== // 1. Print out the total number of customers waiting // in line by invoking TotalCustomersInLine. //…arrow_forwardmain.cc file #include <iostream>#include <memory> #include "customer.h" int main() { // Creates a line of customers with Adele at the front. // LinkedList diagram: // Adele -> Kehlani -> Giveon -> Drake -> Ruel std::shared_ptr<Customer> ruel = std::make_shared<Customer>("Ruel", 5, nullptr); std::shared_ptr<Customer> drake = std::make_shared<Customer>("Drake", 8, ruel); std::shared_ptr<Customer> giveon = std::make_shared<Customer>("Giveon", 2, drake); std::shared_ptr<Customer> kehlani = std::make_shared<Customer>("Kehlani", 15, giveon); std::shared_ptr<Customer> adele = std::make_shared<Customer>("Adele", 4, kehlani); std::cout << "Total customers waiting: "; // =================== YOUR CODE HERE =================== // 1. Print out the total number of customers waiting // in line by invoking TotalCustomersInLine. //…arrow_forwardprg-1 #include <iostream>using namespace std; struct Node { int data; Node* left; Node* right;}; Node* newNode(int data) { Node* node = new Node; node->data = data; node->left = NULL; node->right = NULL; return node;} void printInorder(Node* node) { if (node == NULL) return; printInorder(node->left); cout << node->data << " "; printInorder(node->right);} void printPreorder(Node* node) { if (node == NULL) return; cout << node->data << " "; printPreorder(node->left); printPreorder(node->right);} void printPostorder(Node* node) { if (node == NULL) return; printPostorder(node->left); printPostorder(node->right); cout << node->data << " ";} int main() { Node* root = newNode(70); root->left = newNode(60); root->left->left = newNode(58); root->left->right = newNode(62); root->left->left->left =…arrow_forwardC++ Program #include <iostream>#include <cstdlib>#include <ctime>using namespace std; int getData() { return (rand() % 100);} class Node {public: int data; Node* next;}; class LinkedList{public: LinkedList() { // constructor head = NULL; } ~LinkedList() {}; // destructor void addNode(int val); void addNodeSorted(int val); void displayWithCount(); int size(); void deleteAllNodes(); bool exists(int val);private: Node* head;}; // function to check data exist in a listbool LinkedList::exists(int val){ if (head == NULL) { return false; } else { Node* temp = head; while (temp != NULL) { if(temp->data == val){ return true; } temp = temp->next; } } return false;} // function to delete all data in a listvoid LinkedList::deleteAllNodes(){ if (head == NULL) { cout << "List is empty, No need to delete…arrow_forwardC++ problem #include <iostream> #include <string> using namespace std; class node { public: string data; node* next; }; //Print all items from first to end of list void printList(node* first) { } int main() { //Create a new node with data "Tiger" node* T; T = new node; (*T).data = "Tiger"; //Create a node with "Monkey" node* M; M = new node; (*M).data = "Monkeykey"; //Now, hook T's next field to point to M. (*T).next = M; //Let's create a "Whale" node node* W; W = new node; (*W).data = "Whale"; //Hook M's next to W (*M).next = W; //Let's create a "Dpg" node node* D; D = new node; (*D).data = "Dog"; //Let's hook W's next to D (*W).next = D; //Make next point of last item //point to nothing (null) (*D).next = nullptr; // Step 1: printList(T); //Print: Tiger Monkey Whale Dog // Step 2: create function that removes last item from…arrow_forward//give the output of the following program and draw the link list. struct node{ int info; struct node *link;};typedef struct node *nodePTR;void insort(nodePTR*,struct Info);nodePTR getnode();int main(void){nodePTR p=NULL,head=NULL,save;inti; for(i=0;i<3;i++){ insort (&head,i+5);}save=head;do{ printf("%d",save->info); save=save->link;}while(save!=NULL); return0;}nodePTR getnode(){nodePTR q;q=(nodePTR)malloc(sizeof(struct node));return q;}void insort(NODEPTR *head , int x){ NODEPTR p,q,r; q=NULL; for(p=*head; p!=NULL &&x>p->info; p=p->link) q=p; if(q=NULL) { p=getnode(); p->info=x; p->link=head; head=p; }else{ r=getnode(); q->link=r; r->info=x; r->link=p; }}arrow_forward//impl.h#include <string>#include <stack>#include <iostream>using namespace std;class VideoGame{public:string title, genre, publisher;int year;VideoGame(string t, string p, string g, int y){set_title(t);set_genre(g);set_publisher(p);set_year(y);}void set_title(string t){title = t;}void set_genre(string g){genre = g;}void set_publisher(string p){publisher = p;}void set_year(int y){year = y;}string get_publisher(){return publisher;}string get_title(){return title;}string get_genre(){return genre;}int get_year(){return year;}};//impl.cpp#include "impl.h"void createstack(stack <VideoGame> s){while (!s.empty()){VideoGame v = s.top();cout << "TITLE : " << v.get_title() << endl;cout << "YEAR : " << v.get_year() << endl;cout << "GENRE : " << v.get_genre() << endl;cout << "PUBLISHER : " << v.get_publisher() << endl;cout << '\n';cout << "------------------------" <<…arrow_forwardOutput in C please String is: abcdef String is: 1234 String is: cm I can’t get my code to output the stringsarrow_forwardarrow_back_iosSEE MORE QUESTIONSarrow_forward_ios
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
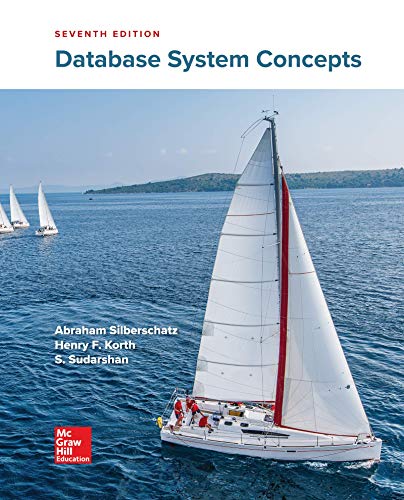
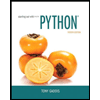
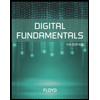
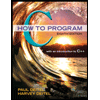
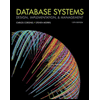
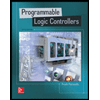