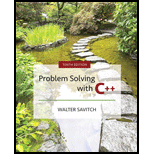
Problem Solving with C++ (10th Edition)
10th Edition
ISBN: 9780134448282
Author: Walter Savitch, Kenrick Mock
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 13.1, Problem 5STE
Program Plan Intro
Linked list:
- Linked list denotes a linear data structure.
- The elements are not stored at contiguous locations; the elements are linked using pointers.
- It stores linear data of similar types not like arrays.
- The size of linked list can be changed based on requirement.
- It is represented by a pointer to first linked list node.
- The first node denotes a head.
- If linked list is empty, value of head is NULL.
- The node in a list has two parts, data and pointer to next node.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
I need help to solve the following case, thank you
hi I would like to get help to resolve the following case
Could you help me to know features of the following concepts:
- defragmenting.
- dynamic disk.
- hardware RAID
Chapter 13 Solutions
Problem Solving with C++ (10th Edition)
Ch. 13.1 - Suppose your program contains the following type...Ch. 13.1 - Suppose that your program contains the type...Ch. 13.1 - Prob. 3STECh. 13.1 - Prob. 4STECh. 13.1 - Prob. 5STECh. 13.1 - Prob. 6STECh. 13.1 - Prob. 7STECh. 13.1 - Suppose your program contains type definitions and...Ch. 13.1 - Prob. 9STECh. 13.2 - Prob. 10STE
Ch. 13.2 - Prob. 11STECh. 13.2 - Prob. 12STECh. 13.2 - Prob. 13STECh. 13 - The following program creates a linked list with...Ch. 13 - Re-do Practice Program 1, but instead of a struct,...Ch. 13 - Write a void function that takes a linked list of...Ch. 13 - Write a function called mergeLists that takes two...Ch. 13 - In this project you will redo Programming Project...Ch. 13 - A harder version of Programming Project 4 would be...Ch. 13 - Prob. 6PPCh. 13 - Prob. 8PPCh. 13 - Prob. 9PPCh. 13 - Prob. 10PP
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- what is a feature in the Windows Server Security Compliance Toolkit, thank you.arrow_forwardYou will write a program that allows the user to keep track of college locations and details about each location. To begin you will create a College python class that keeps track of the csollege's unique id number, name, address, phone number, maximum students, and average tuition cost. Once you have built the College class, you will write a program that stores College objects in a dictionary while using the College's unique id number as the key. The program should display a menu in this order that lets the user: 1) Add a new College 2) Look up a College 4) Delete an existing College 5) Change an existing College's name, address, phone number, maximum guests, and average tuition cost. 6) Exit the programarrow_forwardShow all the workarrow_forward
- Show all the workarrow_forward[5 marks] Give a recursive definition for the language anb2n where n = 1, 2, 3, ... over the alphabet Ó={a, b}. 2) [12 marks] Consider the following languages over the alphabet ={a ,b}, (i) The language of all words that begin and end an a (ii) The language where every a in a word is immediately followed by at least one b. (a) Express each as a Regular Expression (b) Draw an FA for each language (c) For Language (i), draw a TG using at most 3 states (d) For Language (ii), construct a CFG.arrow_forwardQuestion 1 Generate a random sample of standard lognormal data (rlnorm()) for sample size n = 100. Construct histogram estimates of density for this sample using Sturges’ Rule, Scott’s Normal Reference Rule, and the FD Rule. Question 2 Construct a frequency polygon density estimate for the sample in Question 1, using bin width determined by Sturges’ Rule.arrow_forward
- Generate a random sample of standard lognormal data (rlnorm()) for sample size n = 100. Construct histogram estimates of density for this sample using Sturges’ Rule, Scott’s Normal Reference Rule, and the FD Rule.arrow_forwardCan I get help with this case please, thank youarrow_forwardI need help to solve the following, thank youarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage LearningSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
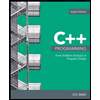
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
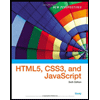
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
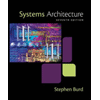
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
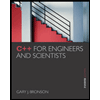
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
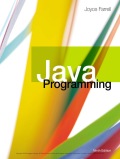
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage