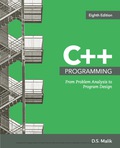
Explanation of Solution
The class dateType has a friend function declared in its definition. The friend function is then defined with two parameters of dateType and a bool return type. The logic of the function is explained with the in-lined comments in the program given below.
//class definition
class dateType
{
//declare the friend function in the class definition
friend bool before(dateType d1, dateType d2);
public:
void setDate(int month, int day, int year);
int getDay() const;
int getMonth() const;
int getYear() const;
void printDate() const;
bool isLeapYear(int) const;
dateType(int month = 1, int day = 1, int year = 1900);
private:
int dMonth; //variable to store the month
int dDay; //variable to store the day
int dYear; //variable to store the year
};
//define the friend function
bool before(dateType d1, dateType d2){
//if the year of first object is strictly less
//than the year of second object return true
//else if the year of the first object is
//strictly more than the year of the second
//object return false else if there is a tie
//then compare the months
if (d1...

Want to see the full answer?
Check out a sample textbook solution
Chapter 13 Solutions
EBK C++ PROGRAMMING: FROM PROBLEM ANALY
- using c++ Develop a class called Point with two data members: x and y. Here you are dealing with a point in planar (2D) Cartesian coordinates with two integer values (x and y). Here are what you need to do: • Define a print function that returns the coordinates of a Point object. • Define a function to find the distance between two points. • Define a function to move a point to the specified location (x’, y’). • Define a function to translate a point by (dx, dy): the new location will be (x+dx, y+dy). • Define functions to tell the user if a point is on the left side, right side, above, or below another point (it can be the combination of some of these or exactly on the same spot). You also need to implement proper constructors (default and parameter constructors), a copy constructor, a destructor, getters, and setters for the data members. Implement the program with separate files: an interface file, an implementation file, and an application file (driver program). Your driver program…arrow_forwardWrite a function template named maximum. The function takes two values of the same type as its arguments and returns the larger of the two arguments (or either value if they are equal). Give both the function declara-tion and the function definition for the template. You will use the operator< in your definition. Therefore, this function template will apply only to types for which < is defined. Write a comment for the function declaration that explains this restriction.arrow_forwardWrite the definition of class StorageFile and a test main() function. Class StorageFile does not contain inline function definitions. I. Write the class declaration for StorageFile and declare (no definitions) the following members: 1. A private member named location of type string. 2. A public default constructor. 3. A public non-default constructor with one parameter of type string. 4. A destructor 5. An accessor (getter) for location. II. Define the following members ONLY. Note that these definitions are placed outside the class' declaration. 1. Default constructor to initialize location to "none" 2. Non-default constructor to initialize location using the constructor's parameter. 3. The accessor (getter) for location II. Define main() to perform the following steps: 1. Create an instance of type StorageFile using the default constructor 2. Create an instance of type StorageFile using the non-default constructor, use "new" for the parameter. 3. Extract and print the value of…arrow_forward
- I have the basis of a program that works as an inventory, it reads the contents of a file and displays them and their quantity in three places, listed below are the things that I need to add to the program below. Provided is the current code. 1) Convert your structure into a class. For this exercise, you can leave the data as public (otherwise you would have to change the input and output functions). 2) Write a member function show_all that prints all the information for one record - name, cost, markup, and the three inventory numbers. 3) Add a user option S that lets the user see all the information for all the items in the inventory, using the show_all member function. Print a header so the user knows what each column means, and format the output to appear in columns. Hint: do a setwidth() before *each* cout. Pick widths that make sense for name, cost, markup, and the three inventory numbers. Contents of the file: Contents of Inventory.txt Red delicious apples1.00 25 6 8…arrow_forward1. Short questions: a. What is the difference between having a function return type being "const dataType &" and it being "dataType&"? b. When is it necessary to make a function or a class a friend of another class? c. List all who can access: the private member of a class protected member of a class public member of a class d. What is difference between static data and a regular data of a class?arrow_forwardQuestion P .Write the implementation (.cpp file) of the Acc2 class of the previous exercise. The full specification of the class is: An data member named sum of type integer. A constructor that accepts no parameters. THe constructor initializes the data member sum to 0. A function named getSum that accepts no parameters and returns an integer. getSum returns the value of sum . Full explain this question and text typing work only We should answer our question within 2 hours takes more time then we will reduce Rating Dont ignore this linearrow_forward
- This problem is to get you familiar with virtual functions. Create three classes Person, Professor and Student. The class Person should have data members name and age. The classes Professor and Student should inherit from the class Person. The class Professor should have two integer members: publications and cur_id. There will be two member functions: getdata and putdata. The function getdata should get the input from the user: the name, age and publications of the professor. The function putdata should print the name, age, publications and the cur_id of the professor. The class Student should have two data members: marks, which is an array of size and cur_id. It has two member functions: getdata and putdata. The function getdata should get the input from the user: the name, age, and the marks of the student in subjects. The function putdata should print the name, age, sum of the marks and the cur_id of the student. For each object being created of the Professor or the Student class,…arrow_forwardMore than one options can be correct. Please give the right answer. Thanks in advancearrow_forwardAll of the functions in the class A(n)_ are virtual functions. Let us know what you think by filling in the gaps.arrow_forward
- Suppose you are writing a class called Student that has two private members, std::string name and int perm. Write the function prototype (function declaration) only for a default constructor for Student. For full credit, include the semicolon that terminates the prototype, but do not write the body of the constructorarrow_forwardExplain the question in a very simple way Example 3: Student Grades (Returning a List of Objects from Function) create a class Student, with attributes name, and grade.We will return students who have a grade above a specified threshold using a function. class Student: def __init__(self, name, grade): self.name = name self.grade = grade def high achievers(students, min_grade): return (student for student in students if student.grade > min_grade] students = [ Student("Alice", 85), Student("Bob", 76), Student("Charlie", 92), Student("Diana", 88) • Output: top_students = high achievers(students, 80) for student in top_students: print(f"Student: (student.name}, Grade: (student.grade}") 27/04/1446 • Student: Alice, Grade: 89 Student: Charlie, Grade • Student: Diana, Gradearrow_forwardc++arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
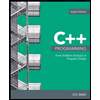