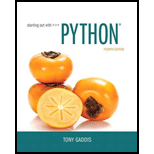
Concept explainers
Ackermann's Function
Ackermann's Function is a recursive mathematical
If m = 0 then return n + 1
If n = 0 then return ackermann (m - 1, 1)
Otherwise, return ackermann (m - 1, ackermann (m, n - 1))
Once you've designed your function, test it by calling it with small values for m and n.

Want to see the full answer?
Check out a sample textbook solution
Chapter 12 Solutions
Starting Out with Python (4th Edition)
Additional Engineering Textbook Solutions
Starting Out with Programming Logic and Design (4th Edition)
Web Development and Design Foundations with HTML5 (9th Edition) (What's New in Computer Science)
Experiencing MIS
Web Development and Design Foundations with HTML5 (8th Edition)
Java: An Introduction to Problem Solving and Programming (8th Edition)
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
- By using a recursive function to find s value: S= 2/x – 4/x + 6/x – 8/x .. 2n/xarrow_forwardWrite a recursive function that takes an array and a callback function and returns True if any value of that array returns True from that callback function otherwise returns False.As a callback funtion for this problem, assume that the callback function is used to check if a given input is an even number.- Analyse the running time of the algorithmarrow_forwardFor function addOdd(n) write the missing recursive call. This function should return the sum of all postive odd numbers less than or equal to n. Examples: addOdd(1) -> 1addOdd(2) -> 1addOdd(3) -> 4addOdd(7) -> 16 public int addOdd(int n) { if (n <= 0) { return 0; } if (n % 2 != 0) { // Odd value return <<Missing a Recursive call>> } else { // Even value return addOdd(n - 1); }}arrow_forward
- In the absence of a exit condition in a recursive function, the following error is givenarrow_forwardWrite a recursive function that returns the nth Fibonacci number from the Fibonacciseries.int fib(int n);arrow_forwardWrite a recursive Python function that calculates the values of the following series, defined by : -U0=1-U1=1-Un=Un-1+Un-2 write it in python code.arrow_forward
- Write a recursive function that finds n-th power of number m. Ex:m=3 n=4 Ans=81 WRITE IN PYTHON PLEASEarrow_forwardcomplete the identified statement such that the recursive function funx(n+1) = n*funx(n-1), any positive integer value of n, and funx(1)=1. 1 float funx (int n) 3 if (n <= 1) 4 return l; else 6 return // complete this statement 7arrow_forwardlanguage: Python Problem: Write a recursive function power(x, n), where n is 0 or a postive integer. For example, power(2, 10) will return 1024. Write a suitable base case, and for the general case use the idea that x" = x* x"-1.arrow_forward
- Write a recursive function to generate nth fibonacci term in C programming. How to generate nth fibonacci term in C programming using recursion. Logic to find nth Fibonacci term using recursion in C programming. Fibonacci series is a series of numbers where the current number is the sum of previous two terms. For Example: 0, 1, 1, 2, 3, 5, 8, 13, 21, ... , (n-1th + n-2th) Example: Input: Input any number: 10 Output 10th Fibonacci term: 55 please use C languagearrow_forwardWrite the definition of a recursive function int simpleSqrt(int n) The function returns the integer square root of n, meaning the biggest integer whose square is less than or equal to n. You may assume that the function is always called with a nonnegative value for n. Use the following algorithm: If n is 0 then return 0. Otherwise, call the function recursively with n-1 as the argument to get a number t. Check whether or not t+1 squared is strictly greater than n. Based on that test, return the correct result. For example, a call to simpleSqrt(8) would recursively call simpleSqrt(7) and get back 2 as the answer. Then we would square (2+1) = 3 to get 9. Since 9 is bigger than 8, we know that 3 is too big, so return 2 in this case. On the other hand a call to simpleSqrt(9) would recursively call simpleSqrt(8) and get back 2 as the answer. Again we would square (2+1) = 3 to get back 9. So 3 is the correct return value in this case.arrow_forwardjsarrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
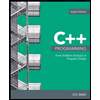