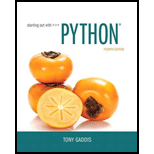
Starting Out with Python (4th Edition)
4th Edition
ISBN: 9780134444321
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 12, Problem 4MC
Program Plan Intro
Problem Solving:
In Python, a problem can be divided into smaller parts only if it has an identical structure to the whole problem. Then, it can be solved using recursion.
Working method of recursive function:
A recursive function has two approaches. They are as follows:
- If a problem can be solved currently without using recursion, then use function to solve it and returns.
- If a problem cannot be solved currently, then the function reduces the problem into smaller parts only if it has a similar structure to the whole program. And the function calls itself to solve the sub-parts.
Problem cases:
In order to apply the above approaches into the program for solving it, then it is important to identify the problem case. There are two cases that are available in problem solving. They are base case and recursive case.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
In a recursive solution, the _____ case is easily calculated, provides a stopping criterion, and prevents infinite loops. In the _____ case, the solution calls itself.
Recursive Power MethodWrite a method called powCal that uses recursion to raise a number to a power. The method should accept two arguments: The first argument is the exponent and the second argument is the number to be raised (example” powCal(10,2) means 210). Assume that the exponent is a nonnegative integer. Demonstrate the method in a program called Recursive
(This means that you need to write a program that has at least two methods: main and powCal. The powCal method is where you implement the requirements above and the main method is where you make a method call to demonstrate how your powCal method work).
3-The following pattern of numbers is called Pascal's triangle.
1
1
1
14
641
The numbers at the edge of the triangle are all 1, and each number inside the
triangle is the sum of the two numbers above it. Write a procedure that computes
elements of Pascal's triangle by means of a recursive process.
1
1
1
2 1
3 3
Chapter 12 Solutions
Starting Out with Python (4th Edition)
Ch. 12.2 - It is said that a recursive algorithm has more...Ch. 12.2 - Prob. 2CPCh. 12.2 - What is a recursive case?Ch. 12.2 - What causes a recursive algorithm to stop calling...Ch. 12.2 - What is direct recursion? What is indirect...Ch. 12 - Prob. 1MCCh. 12 - A function is called once from a program's main...Ch. 12 - Prob. 3MCCh. 12 - Prob. 4MCCh. 12 - Prob. 5MC
Ch. 12 - Prob. 6MCCh. 12 - Any problem that can be solved recursively can...Ch. 12 - Actions taken by the computer when a function is...Ch. 12 - A recursive algorithm must _______ in the...Ch. 12 - A recursive algorithm must ______ in the base...Ch. 12 - An algorithm that uses a loop will usually run...Ch. 12 - Some problems can be solved through recursion...Ch. 12 - It is not necessary to have a base case in all...Ch. 12 - In the base case, a recursive method calls itself...Ch. 12 - In Program 12-2 , presented earlier in this...Ch. 12 - In this chapter, the rules given for calculating...Ch. 12 - Is recursion ever required to solve a problem?...Ch. 12 - When recursion is used to solve a problem, why...Ch. 12 - How is a problem usually reduced with a recursive...Ch. 12 - What will the following program display? def...Ch. 12 - Prob. 2AWCh. 12 - The following function uses a loop. Rewrite it as...Ch. 12 - Prob. 1PECh. 12 - Prob. 2PECh. 12 - Prob. 3PECh. 12 - Largest List Item Design a function that accepts a...Ch. 12 - Recursive List Sum Design a function that accepts...Ch. 12 - Prob. 6PECh. 12 - Prob. 7PECh. 12 - Ackermann's Function Ackermann's Function is a...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- q6arrow_forwardLab Goal : This lab was designed to teach you more about recursion. Lab Description : Take a number and recursively determine how many of its digits are even. Return the count of the even digits in each number. % might prove useful to take the number apart digit by digit Sample Data : 453211145322224532714246813579 Sample Output : 23540arrow_forwardpytthon plzzzzzarrow_forward
- Magic Number of coding-:A number is said to be a magic number,if summing the digits of the number and then recursively repeating this process for the given sumuntill the number becomes a single digit number equal to 1. Example: Number = 50113 => 5+0+1+1+3=10 => 1+0=1 [This is a Magic Number] Number = 1234 => 1+2+3+4=10 => 1+0=1 [This is a Magic Number] Number = 199 => 1+9+9=19 => 1+9=10 => 1+0=1 [This is a Magic Number] Number = 111 => 1+1+1=3 [This is NOT a Magic Number].arrow_forwardLab Goal : This lab was designed to teach you more about recursion. Lab Description : luckyThrees will return a count of the 3s in the number unless the 3 is at the start. A 3 at the start of the number does not count. /* luckyThrees will return the count of 3s in the number* unless the 3 is at the front and then it does not count* 3 would return 0* 31332 would return 2* 134523 would return 2* 3113 would return 1* 13331 would return 3* 777337777 would return 2* the solution to this problem must use recursion*/public static int luckyThrees( long number ){} Sample Data : 331332134523311313331777337777 Sample Output : 022132arrow_forwardJava 2arrow_forward
- Magic Number coding question---1. A number is said to be a magic number,if summing the digits of the number and then recursively repeating this process for the given sumuntill the number becomes a single digit number equal to 1. Example: Number = 50113 => 5+0+1+1+3=10 => 1+0=1 [This is a Magic Number] Number = 1234 => 1+2+3+4=10 => 1+0=1 [This is a Magic Number] Number = 199 => 1+9+9=19 => 1+9=10 => 1+0=1 [This is a Magic Number] Number = 111 => 1+1+1=3 [This is NOT a Magic Number].arrow_forwardLab Goal : This lab was designed to teach you more about recursion. Lab Description : Take a number and recursively determine how many of its digits are even. Return the count of the even digits in each number. % might prove useful to take the number apart digit by digitSample Data : 453211145322224532714246813579Sample Output : 23540 I want a code class and a runner classarrow_forwardPYTHON RECURSIVE FUNCTION Write a python program that lists all ways people can line up for a photo (all permutations of a list of strings). The program will read a list of one word names, then use a recursive method to create and output all possible orderings of those names, one ordering per line. When the input is: Julia Lucas Mia then the output is (must match the below ordering): Julia Lucas Mia Julia Mia Lucas Lucas Julia Mia Lucas Mia Julia Mia Julia Lucas Mia Lucas Juliaarrow_forward
- Magic Number Code question::-1.A number is said to be a magic number,if summing the digits of the number and then recursively repeating this process for the given sumuntill the number becomes a single digit number equal to 1. Example: Number = 50113 => 5+0+1+1+3=10 => 1+0=1 [This is a Magic Number] Number = 1234 => 1+2+3+4=10 => 1+0=1 [This is a Magic Number] Number = 199 => 1+9+9=19 => 1+9=10 => 1+0=1 [This is a Magic Number] Number = 111 => 1+1+1=3 [This is NOT a Magic Number].arrow_forwardBacktracking normally uses ______ to find out all solutions to a computational problem. (recursion, iteration).arrow_forward2. Sum: a recursive function that computes the sum of integers 1, 2, 3, …., n for a given number n. So Sum(6) should return 1 + 2 + 3 + 4 + 5 + 6 , i.e. 21.sum(n) = n + sum(n-1)arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
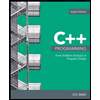
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning