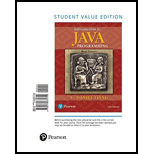
Introduction to Java Programming and Data Structures: Brief Version (11th Global Edition)
11th Edition
ISBN: 9780134671710
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Textbook Question
Chapter 11.4, Problem 11.4.2CP
True or false? You can override a static method defined in a superclass.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Complete the JavaScript function addPixels () to calculate the sum of pixelAmount and the given element's
cssProperty value, and return the new "px" value.
Ex: If helloElem's width is 150px, then calling addPixels (hello Elem, "width", 50) should return 150px + 50px =
"200px".
SHOW EXPECTED
HTML JavaScript
1 function addPixels (element, cssProperty, pixelAmount) {
2
3
/* Your solution goes here *1
4 }
5
6 const helloElem = document.querySelector("# helloMessage");
7 const newVal = addPixels (helloElem, "width", 50);
8 helloElem.style.setProperty("width", newVal);
[
Solve in MATLAB
Hello please look at the attached picture. I need an detailed explanation of the architecture
Chapter 11 Solutions
Introduction to Java Programming and Data Structures: Brief Version (11th Global Edition)
Ch. 11.2 - True or false? A subclass is subset of a...Ch. 11.2 - What keyword do you use to define a subclass?Ch. 11.2 - What is single inheritance? What is multiple...Ch. 11.3 - What is the output of running the class C in (a)?...Ch. 11.3 - How does a subclass invoke its superclasss...Ch. 11.3 - True or false? When invoking a constructor from a...Ch. 11.4 - True or false? You can override a private method...Ch. 11.4 - True or false? You can override a static method...Ch. 11.4 - How do you explicitly invoke a superclasss...Ch. 11.4 - How do you invoke an overridden superclass method...
Ch. 11.5 - Identify the problems in the following code:...Ch. 11.5 - Prob. 11.5.2CPCh. 11.5 - If a method in a subclass has the same signature...Ch. 11.5 - If a method in a subclass has the same signature...Ch. 11.5 - If a method in a subclass has the same name as a...Ch. 11.5 - Prob. 11.5.6CPCh. 11.7 - Prob. 11.7.1CPCh. 11.8 - Prob. 11.8.1CPCh. 11.8 - Prob. 11.8.2CPCh. 11.8 - Can you assign new int[50], new Integer [50], new...Ch. 11.8 - Prob. 11.8.4CPCh. 11.8 - Show the output of the following code:Ch. 11.8 - Show the output of following program: 1public...Ch. 11.8 - Show the output of following program: public class...Ch. 11.9 - Indicate true or false for the following...Ch. 11.9 - For the GeometricObject and Circle classes in...Ch. 11.9 - Suppose Fruit, Apple, Orange, GoldenDelicious, and...Ch. 11.9 - What is wrong in the following code? 1public class...Ch. 11.10 - Prob. 11.10.1CPCh. 11.11 - Prob. 11.11.1CPCh. 11.11 - Prob. 11.11.2CPCh. 11.11 - Prob. 11.11.3CPCh. 11.11 - Prob. 11.11.4CPCh. 11.11 - Prob. 11.11.5CPCh. 11.12 - Correct errors in the following statements: int[]...Ch. 11.12 - Correct errors in the following statements: int[]...Ch. 11.13 - Prob. 11.13.1CPCh. 11.14 - What modifier should you use on a class so a class...Ch. 11.14 - Prob. 11.14.2CPCh. 11.14 - In the following code, the classes A and B are in...Ch. 11.14 - In the following code, the classes A and B are in...Ch. 11.15 - Prob. 11.15.1CPCh. 11.15 - Indicate true or false for the following...Ch. 11 - Sections 11.211.4 11.1(The Triangle class) Design...Ch. 11 - (Subclasses of Account) In Programming Exercise...Ch. 11 - (Maximum element in ArrayList) Write the following...Ch. 11 - Prob. 11.5PECh. 11 - (Use ArrayList) Write a program that creates an...Ch. 11 - (Shuffle ArrayList) Write the following method...Ch. 11 - (New Account class) An Account class was specified...Ch. 11 - (Largest rows and columns) Write a program that...Ch. 11 - Prob. 11.10PECh. 11 - (Sort ArrayList) Write the following method that...Ch. 11 - (Sum ArrayList) Write the following method that...Ch. 11 - (Remove duplicates) Write a method that removes...Ch. 11 - (Combine two lists) Write a method that returns...Ch. 11 - (Area of a convex polygon) A polygon is convex if...Ch. 11 - Prob. 11.16PECh. 11 - (Algebra: perfect square) Write a program that...Ch. 11 - (ArrayList of Character) Write a method that...Ch. 11 - (Bin packing using first fit) The bin packing...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
Give an example of a data constraint.
Database Concepts (8th Edition)
What populates the Smalltalk world?
Concepts Of Programming Languages
Pattern Displays Write a program that uses a loop to display Pattern A below, followed by another loop that dis...
Starting Out with C++ from Control Structures to Objects (9th Edition)
For the circuit shown, use the node-voltage method to find v1, v2, and i1.
How much power is delivered to the c...
Electric Circuits. (11th Edition)
2-1 List the five types of measurements that form the
basis of traditional ptane surveying-
Elementary Surveying: An Introduction To Geomatics (15th Edition)
Consider a Java class that you could use to get an acceptable integer value from the user. An object of this cl...
Java: An Introduction to Problem Solving and Programming (8th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Information Security Risk and Vulnerability Assessment 1- Which TCP/IP protocol is used to convert the IP address to the Mac address? Explain 2-What popular switch feature allows you to create communication boundaries between systems connected to the switch3- what types of vulnerability directly related to the programmer of the software?4- Who ensures the entity implements appropriate security controls to protect an asset? Please do not use AI and add refrencearrow_forwardFind the voltage V0 across the 4K resistor using the mesh method or nodal analysis. Note: I have already simulated it and the value it should give is -1.714Varrow_forwardResolver por superposicionarrow_forward
- Describe three (3) Multiplexing techniques common for fiber optic linksarrow_forwardCould you help me to know features of the following concepts: - commercial CA - memory integrity - WMI filterarrow_forwardBriefly describe the issues involved in using ATM technology in Local Area Networksarrow_forward
- For this question you will perform two levels of quicksort on an array containing these numbers: 59 41 61 73 43 57 50 13 96 88 42 77 27 95 32 89 In the first blank, enter the array contents after the top level partition. In the second blank, enter the array contents after one more partition of the left-hand subarray resulting from the first partition. In the third blank, enter the array contents after one more partition of the right-hand subarray resulting from the first partition. Print the numbers with a single space between them. Use the algorithm we covered in class, in which the first element of the subarray is the partition value. Question 1 options: Blank # 1 Blank # 2 Blank # 3arrow_forward1. Transform the E-R diagram into a set of relations. Country_of Agent ID Agent H Holds Is_Reponsible_for Consignment Number $ Value May Contain Consignment Transports Container Destination Ф R Goes Off Container Number Size Vessel Voyage Registry Vessel ID Voyage_ID Tonnagearrow_forwardI want to solve 13.2 using matlab please helparrow_forward
- a) Show a possible trace of the OSPF algorithm for computing the routing table in Router 2 forthis network.b) Show the messages used by RIP to compute routing tables.arrow_forwardusing r language to answer question 4 Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.arrow_forwardusing r language to answer question 4. Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
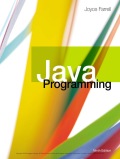
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
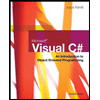
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
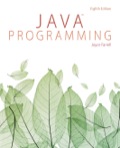
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
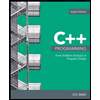
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
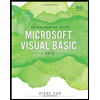
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
Introduction to Classes and Objects - Part 1 (Data Structures & Algorithms #3); Author: CS Dojo;https://www.youtube.com/watch?v=8yjkWGRlUmY;License: Standard YouTube License, CC-BY