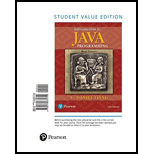
(Maximum element in ArrayList) Write the following method that returns the maximum value in an ArrayList of integers. The method returns null if the list is null or the list size is 0.
public static Integer max(ArrayList<Integer> list)
Write a test

Want to see the full answer?
Check out a sample textbook solution
Chapter 11 Solutions
Introduction to Java Programming and Data Structures: Brief Version (11th Global Edition)
Additional Engineering Textbook Solutions
Elementary Surveying: An Introduction To Geomatics (15th Edition)
Degarmo's Materials And Processes In Manufacturing
Electric Circuits. (11th Edition)
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
Web Development and Design Foundations with HTML5 (8th Edition)
- 6. See the code below and solve the following. import java.io.*; public class DataStream { } public static void main(String[] args) } DataOutputStream output = new DataOutputStream(new FileOutputStream("temp.dat")); output.writeUTF("Book1"); output.writeInt(85); output.writeUTF("Book2"); output.writeInt(125); output.writeUTF("Book3"); output.writeInt(70); output.close(); // ToDo: Read all data from temp.dat and print the data to the standard output (monitor) 6-1. This program has a compile error, and the message is “Unhandled exception type FileNotFoundException". How do you fix this error? (1 point) 6-2. Is FileNotFoundException a checked exception or an unchecked exception? (1 point) 6-3. What is the difference between checked exception and unchecked exception? (1 point) 6-4. Please complete the above program by reading all data from temp.dat and print the data to the standard output (monitor) by using System.out.print, System.out.println or System.out.printf method. (2 points)arrow_forwardWrite a program that reads a list of integers from input and determines if the list is a palindrome (values are identical from first to last and last to first). The input begins with an integer indicating the length of the list that follows. Assume the list will contain a maximum of 20 integers. Output "yes" if the list is a palindrome and "no" otherwise. The output ends with a newline. Hints: - use a for loop to populate the array based on the specified size (the first number entered) - use a for loop to check first value with last value, second value with second from end, etc. - if the values do not match, set a Boolean variable to flag which statement to output (yes or no) Ex: If the input is (remember to include spaces between the numbers): 6 1 5 9 9 5 1 the output is: yes Ex: If the input is: 5 1 2 3 4 5 the output is: C++ codingarrow_forwardDesign and draw a high-level "as-is" process diagram that illustrates a current process related to a product or service offered through the SSDCI.gov database.arrow_forward
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
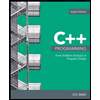
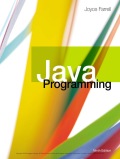
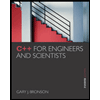
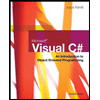
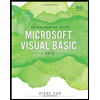