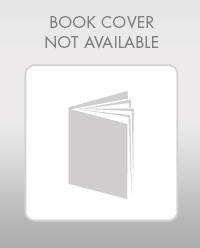
Starting Out With C++: Early Objects (10th Edition)
10th Edition
ISBN: 9780135235003
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Expert Solution & Answer
Chapter 10.10, Problem 10.16CP
Explanation of Solution
Returning a pointer from the function:
Pointers can be returned from the function only if the reference pointer variable that points exists in the function.
Circumstance for successful return of a pointer:
The circumstances for returning pointer successful are listed below:
- When a pointer is passed as an argument in a function that is called.
- When a pointer variable is defined locally within the function there may be possibility for the contents of the variables being destructed after execution of the function. This can cause unexpected results.
- When pointer gets chunk of memory allocated dynamically.
- When a variable is allocated dynamically, the contents will be available until the compiler executes the delete operation.
Example:
// Include the necessary headers
#include <iostream>
using namespace std;
//function prototype to get alphabets
char *getalphabets();
//function prototype to display the array elements
void disparray(char[], int);
//main method
int main()
{
//variable declaration
const int SIZE = 3;
// get alphabets from the user
char *chkname = getalphabets();
//displays the content of array
cout << "The alphabets you entered...
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Suppose your computer is responding very slowly to information requests from the Internet. You observe that your network gateway shows high levels of network activity even though you have closed your e-mail client, Web browser, and all other programs that access the Internet.
What types of malwares could cause such symptoms? What steps can you take to check whether malware has gained access to your system? What tools can you use at each step? If you identify malware, what ways might it have entered your system? How can you restore your PC to safe operation, including the special software tools you may use?
R language
Using R language
Chapter 10 Solutions
Starting Out With C++: Early Objects (10th Edition)
Ch. 10.5 - Prob. 10.1CPCh. 10.5 - Write a statement defining a variable dPtr. The...Ch. 10.5 - List three uses of the symbol in C++.Ch. 10.5 - What is the output of the following program?...Ch. 10.5 - Rewrite the following loop so it uses pointer...Ch. 10.5 - Prob. 10.6CPCh. 10.5 - Assume pint is a pointer variable. For each of the...Ch. 10.5 - For each of the following variable definitions,...Ch. 10.10 - Assuming array is an array of ints, which of the...Ch. 10.10 - Give an example of the proper way to call the...
Ch. 10.10 - Complete the following program skeleton. When...Ch. 10.10 - Look at the following array definition: const int...Ch. 10.10 - Assume ip is a pointer to an int. Write a...Ch. 10.10 - Assume ip is a pointer to an int. Write a...Ch. 10.10 - Prob. 10.15CPCh. 10.10 - Prob. 10.16CPCh. 10.10 - Prob. 10.17CPCh. 10.12 - Prob. 10.18CPCh. 10.12 - Assume the following structure declaration exists...Ch. 10.12 - Prob. 10.20CPCh. 10 - Each byte in memory is assigned a unique _____Ch. 10 - The _____ operator can be used to determine a...Ch. 10 - Prob. 3RQECh. 10 - The _____ operator can be used to work with the...Ch. 10 - Prob. 5RQECh. 10 - Creating variables while a program is running is...Ch. 10 - Prob. 7RQECh. 10 - If the new operator cannot allocate the amount of...Ch. 10 - Prob. 9RQECh. 10 - When a program is finished with a chunk of...Ch. 10 - You should only use the delete operator to...Ch. 10 - What does the indirection operator do?Ch. 10 - Look at the following code. int X = 7; int ptr =...Ch. 10 - Name two different uses for the C++ operator.Ch. 10 - Prob. 15RQECh. 10 - Prob. 16RQECh. 10 - Prob. 17RQECh. 10 - What is the purpose of the new operator?Ch. 10 - What happens when a program uses the new operator...Ch. 10 - Prob. 20RQECh. 10 - Prob. 21RQECh. 10 - Prob. 22RQECh. 10 - Prob. 23RQECh. 10 - Prob. 24RQECh. 10 - Prob. 25RQECh. 10 - Prob. 26RQECh. 10 - What happens when a unique_ptr that is managing an...Ch. 10 - What does the get ( ) method of the unique_ptr...Ch. 10 - Prob. 29RQECh. 10 - Prob. 30RQECh. 10 - Prob. 31RQECh. 10 - Prob. 32RQECh. 10 - Consider the function void change(int p) { P = 20;...Ch. 10 - Prob. 34RQECh. 10 - Write a function whose prototype is void...Ch. 10 - Write a function void switchEnds(int array, int...Ch. 10 - Given the variable initializations int a[5] = {0,...Ch. 10 - Each of the following declarations and program...Ch. 10 - Prob. 39RQECh. 10 - Test Scores #1 Write a program that dynamically...Ch. 10 - Test Scores #2 Modify the program of Programming...Ch. 10 - Indirect Sorting Through Pointers #1 Consider a...Ch. 10 - Indirect Sorting Through Pointers #2 Write a...Ch. 10 - Pie a la Mode In statistics the mode of a set of...Ch. 10 - Median Function In statistics the median of a set...Ch. 10 - Movie Statistics Write a program that can be used...Ch. 10 - Days in Current Month Write a program that can...Ch. 10 - Age Write a program that asks for the users name...Ch. 10 - Prob. 10PC
Knowledge Booster
Similar questions
- Compare the security services provided by a digital signature (DS) with those of a message authentication code (MAC). Assume that Oscar can observe all messages sent between Rina and Naseem. Oscar has no knowledge of any keys but the public one, in the case of DS. State whether DS and MAC protect against each attack and, if they do, how. The value auth(x) is computed with a DS or a MAC algorithm. In each scenario, assume the message M = x#####auth(x). (Message integrity) Rina has the textual data x = “Transfer $1000 to Mark” to send to Naseem. To ensure the integrity of the data, Rina generates auth(x), forms a message M, and then sends M in cleartext to Naseem. Oscar intercepts the message and replaces “Mark” with “Oscar.” Will Naseem detect this in the case of either DS or MAC? If yes, how will Naseem detect it? If not, why? (Replay) Rina has the textual data x = “Transfer $1000 to Mark” to send to Naseem. To ensure the integrity of the data, Rina generates auth(x), forms a message…arrow_forwardI need to resolve the following....You are trying to convince your boss that your company needs to invest in a license for MS-Project (project management software from Microsoft) before beginning a systems project. What arguments would you give her?arrow_forwardWhat are the four types of feasibility? what is the issues addressed by each feasibility component.arrow_forward
- I would like to get ab example of a situation where Agile Methods might be preferable versus the traditional SDLC? What are the characteristics of this situation that give Agile Methods an advantage?arrow_forwardWhat is a functional decomposition diagram? what is a good example of a high level task being broken down into tasks in at least two lower levels (three levels in all).arrow_forwardWhat are the advantages to using a Sytems Analysis and Design model like the SDLC vs. other approaches?arrow_forward
- 3. Problem Description: Define the Circle2D class that contains: Two double data fields named x and y that specify the center of the circle with get methods. • A data field radius with a get method. • A no-arg constructor that creates a default circle with (0, 0) for (x, y) and 1 for radius. • A constructor that creates a circle with the specified x, y, and radius. • A method getArea() that returns the area of the circle. • A method getPerimeter() that returns the perimeter of the circle. • • • A method contains(double x, double y) that returns true if the specified point (x, y) is inside this circle. See Figure (a). A method contains(Circle2D circle) that returns true if the specified circle is inside this circle. See Figure (b). A method overlaps (Circle2D circle) that returns true if the specified circle overlaps with this circle. See the figure below. р O со (a) (b) (c)< Figure (a) A point is inside the circle. (b) A circle is inside another circle. (c) A circle overlaps another…arrow_forward1. Explain in detail with examples each of the following fundamental security design principles: economy of mechanism, fail-safe default, complete mediation, open design, separation of privilege, least privilege, least common mechanism, psychological acceptability, isolation, encapsulation, modularity, layering, and least astonishment.arrow_forwardSecurity in general means the protection of an asset. In the context of computer and network security, explore and explain what assets must be protected within an online university. What the threats are to the security of these assets, and what countermeasures are available to mitigate and protect the organization from such threats. For each of the assets you identify, assign an impact level (low, moderate, or high) for the loss of confidentiality, availability, and integrity. Justify your answers.arrow_forward
- Please include comments and docs comments on the program. The two other classes are Attraction and Entertainment.arrow_forwardObject-Oriented Programming In this separate files. ent, you'll need to build and run a small Zoo in Lennoxville. All classes must be created in Animal (5) First, start by building a class that describes an Animal at a Zoo. It should have one private instance variable for the name of the animal, and one for its hunger status (fed or hungry). Add methods for setting and getting the hunger satus variable, along with a getter for the name. Consider how these should be named for code clarity. For instance, using a method called hungry () to make the animal hungry could be used as a setter for the hunger field. The same logic could be applied to when it's being fed: public void feed () { this.fed = true; Furthermore, the getter for the fed variable could be named is Fed as it is more descriptive about what it answers when compared to get Fed. Keep this technique in mind for future class designs. Zoo (10) Now we have the animals designed and ready for building a little Zoo! Build a class…arrow_forward1.[30 pts] Answer the following questions: a. [10 pts] Write a Boolean equation in sum-of-products canonical form for the truth table shown below: A B C Y 0 0 0 1 0 0 1 0 0 1 0 0 0 1 1 0 1 0 0 1 1 0 1 0 1 1 0 1 1 1 1 0 a. [10 pts] Minimize the Boolean equation you obtained in (a). b. [10 pts] Implement, using Logisim, the simplified logic circuit. Include an image of the circuit in your report. 2. [20 pts] Student A B will enjoy his picnic on sunny days that have no ants. He will also enjoy his picnic any day he sees a hummingbird, as well as on days where there are ants and ladybugs. a. Write a Boolean equation for his enjoyment (E) in terms of sun (S), ants (A), hummingbirds (H), and ladybugs (L). b. Implement in Logisim, the logic circuit of E function. Use the Circuit Analysis tool in Logisim to view the expression, include an image of the expression generated by Logisim in your report. 3.[20 pts] Find the minimum equivalent circuit for the one shown below (show your work): DAB C…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
- New Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
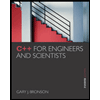
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
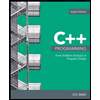
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
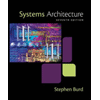
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
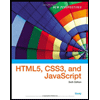
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
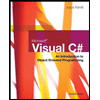
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage