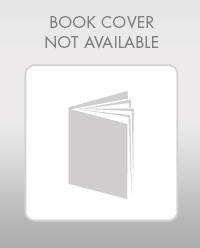
Starting Out With C++: Early Objects (10th Edition)
10th Edition
ISBN: 9780135235003
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 10.12, Problem 10.18CP
Program Plan Intro
Pointer:
- Pointer is a special type of variable used to store the address of the memory location, which can be accessed later.
- If an asterisk “*” operator is present before the variable, then that variable is referred as pointer variable.
- It is also called as dereferencing or indirection operator.
- Pointer is just a type of variable that stores the addresses of other variables.
- Using pointers, it is easy to access the address of a variable; the data stored in that variable can be retrieved.
Syntax of pointer variable declaration:
<variable-type> *<variable-name>;
Define the pointers to structures:
Define the pointers to structure is similar to pointer variable declaration.
Example:
//structure declaration
struct student
{
//variable declaration
string name;
//dynamically allocate array
int *score;
//variable declaration
int total;
};
Pointer is the definition to the above defined structure that can be given as follows:
//defining the pointer definition
student *stu1;
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Programming Language: C++
Create an Abstract Data Type, which will store 2 integer number. After that, make operations which will set the value of the two numbers, add, subtract, multiply and divide. After creating the abstract data type, use it in the main class. Ask the user for the value of two integers. Ask the user what operation to use, then call the necessary operation based on the user's input. Then display the answer with the correct label.
Do not copy paste codes from other sites
Activity 1
This program firstly initializes an object of struct Cirele with values and then updates those
using pointers. The program uses structure notation once and pointer notation another
afterwards.
#include
#include
#define MIN 1
#define MAX 30
struct Circle {
float xCoord;
float yCoord;
float radius;
};
/* prototypes */
float getRandomNumber (int min, int max);
void printByValue(struct Circle cirobj);
void setValue(float *target, float newValue);
void printByReference(struct Circle *cirObj);
int main(void) {
/* Declare a struct circle and name it circleobject */
struct Circle circleObject;
/* Fill circleObject's members with random numbers */
circleobject.xCoord = getRandomNumber(MIN, MAX);
circleobject.yCoord = getRandomNumber (MIN, MAX);
circleobject.radius = getRandomNumber (MIN, MAX);
/* Printing values of the circle by calling function print by value */
printByValue(circleobject);
/* update values of circle one by one */
setValue (&circleobject.xCoord, getRandomNumber(MIN,…
C++
Chapter 10 Solutions
Starting Out With C++: Early Objects (10th Edition)
Ch. 10.5 - Prob. 10.1CPCh. 10.5 - Write a statement defining a variable dPtr. The...Ch. 10.5 - List three uses of the symbol in C++.Ch. 10.5 - What is the output of the following program?...Ch. 10.5 - Rewrite the following loop so it uses pointer...Ch. 10.5 - Prob. 10.6CPCh. 10.5 - Assume pint is a pointer variable. For each of the...Ch. 10.5 - For each of the following variable definitions,...Ch. 10.10 - Assuming array is an array of ints, which of the...Ch. 10.10 - Give an example of the proper way to call the...
Ch. 10.10 - Complete the following program skeleton. When...Ch. 10.10 - Look at the following array definition: const int...Ch. 10.10 - Assume ip is a pointer to an int. Write a...Ch. 10.10 - Assume ip is a pointer to an int. Write a...Ch. 10.10 - Prob. 10.15CPCh. 10.10 - Prob. 10.16CPCh. 10.10 - Prob. 10.17CPCh. 10.12 - Prob. 10.18CPCh. 10.12 - Assume the following structure declaration exists...Ch. 10.12 - Prob. 10.20CPCh. 10 - Each byte in memory is assigned a unique _____Ch. 10 - The _____ operator can be used to determine a...Ch. 10 - Prob. 3RQECh. 10 - The _____ operator can be used to work with the...Ch. 10 - Prob. 5RQECh. 10 - Creating variables while a program is running is...Ch. 10 - Prob. 7RQECh. 10 - If the new operator cannot allocate the amount of...Ch. 10 - Prob. 9RQECh. 10 - When a program is finished with a chunk of...Ch. 10 - You should only use the delete operator to...Ch. 10 - What does the indirection operator do?Ch. 10 - Look at the following code. int X = 7; int ptr =...Ch. 10 - Name two different uses for the C++ operator.Ch. 10 - Prob. 15RQECh. 10 - Prob. 16RQECh. 10 - Prob. 17RQECh. 10 - What is the purpose of the new operator?Ch. 10 - What happens when a program uses the new operator...Ch. 10 - Prob. 20RQECh. 10 - Prob. 21RQECh. 10 - Prob. 22RQECh. 10 - Prob. 23RQECh. 10 - Prob. 24RQECh. 10 - Prob. 25RQECh. 10 - Prob. 26RQECh. 10 - What happens when a unique_ptr that is managing an...Ch. 10 - What does the get ( ) method of the unique_ptr...Ch. 10 - Prob. 29RQECh. 10 - Prob. 30RQECh. 10 - Prob. 31RQECh. 10 - Prob. 32RQECh. 10 - Consider the function void change(int p) { P = 20;...Ch. 10 - Prob. 34RQECh. 10 - Write a function whose prototype is void...Ch. 10 - Write a function void switchEnds(int array, int...Ch. 10 - Given the variable initializations int a[5] = {0,...Ch. 10 - Each of the following declarations and program...Ch. 10 - Prob. 39RQECh. 10 - Test Scores #1 Write a program that dynamically...Ch. 10 - Test Scores #2 Modify the program of Programming...Ch. 10 - Indirect Sorting Through Pointers #1 Consider a...Ch. 10 - Indirect Sorting Through Pointers #2 Write a...Ch. 10 - Pie a la Mode In statistics the mode of a set of...Ch. 10 - Median Function In statistics the median of a set...Ch. 10 - Movie Statistics Write a program that can be used...Ch. 10 - Days in Current Month Write a program that can...Ch. 10 - Age Write a program that asks for the users name...Ch. 10 - Prob. 10PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- *Java code* Implement a data structure called RunningTotal supporting the following API: a) RunningTotal() - creates an empty running-total data structure b) void add(int value) - adds the given value to the data structure c) void remove() - removes the least-recently added value d) int sum() - returns the sum of values in the data structure e) double average() - returns the average of values in the data structure Here's a Example: RunningTotal rt = new RunningTotal ();rt . add (1); // 1 ( adds 1 )rt . add (2); // 1 2 ( adds 2 )rt . add (3); // 1 2 3 ( adds 3 )rt . sum (); // 1 2 3 ( returns 6 )rt . add (4); // 1 2 3 4 ( adds 4 )rt . remove (); // 2 3 4 ( removes 1 )rt . sum (); // 2 3 4 ( returns 9 )rt . average (); // 2 3 4 ( returns 3.0 )arrow_forwardLanguage: C++ Imagine that you are in the design stage of a software system for handling the data of the partici- pants of a major soccer tournament. As different roles will be present (players, coaches, referees, etc.) you are required to develop a class handling the data of a generic league member. For each person the following data is at least needed • first name as character array (36 characters characters including '\ 0') • last name as character array (36 characters) • date of birth as character array (11 characters, storage format is yyyy-mm-dd) In addition the whole team is located somewhere, so location is an additional static prop- erty of the class. Design and implement a class for holding these data. In addition add at least two other general properties to this class. The class, which will be called TournamentMember, should provide constructors (empty and parametric), a destructor and also a copy constructor. The class should also provide inline setter and getter methods…arrow_forwardC++ I need to create a 2D array map that can be displayed in the output like a 2D array visually.arrow_forward
- Attach code and screenshotarrow_forwardPlease code in C++ use comments and explain your code.arrow_forwardTopic: pointers, dynamic array and command line arguments Write a complete C++ program named “showHelp” that accepts command line arguments. It checks whether there is a command line argument of “/help” or“/?” or “-help” followed by a topic number. It will print out “yes, topic number(<number>) if there is one. Otherwise, it prints out “no, topic number(N/A) For example, if you run this program with correct arguments as follows, it willprint out yes and its associated topic number respectivelyshowHelp /? 101showHelp /debug /help 102showHelp /print /help 103 /verboseshowHelp -verbose -debug -help And if you run the program with invalid arguments, it will print no, in all casesshowHelp -helpshowHelp 101 /?showHelp 101 102 /help /verboseshowHelp /? /help -helpNote: command line arguments are simply an array of pointers to C-string. example code: #include <iostream> using namespace std; int main(int argc, char** argv){...}arrow_forward
- The Rectangle has two data members upperLeft and lowerRight points, that represents upper left and lower right coordinates, respectively. Therefore a rectangle can be represented by a nested structure having two structures of the type POINTS as defined below.typedef struct{Point upperLeft;Point lowerRight;}Rectangle; implement the following functions:a. float length (Rectangle rec); //returns the length of the rectangleb. float width (Rectangle rec); //returns the width of the rectanglec. float area (Rectangle rec); //returns the area of the rectangled. float perimeter(Rectangle rec); //returns the area of the rectanglearrow_forwardCreate an Organization class. Organization has 10 Employees (Hint: You will need an array of pointers to Employee class) Organization can calculate the total amount to be paid to all employees Organization can print the details(name & salary) of all employees note: write code in main,header and function.cpp filearrow_forwardLanguage: JAVA Script write a function 'keyValueDuplicates (obj) that takes an object as an argument and returns an array containing all keys that are also values in that object. Examples: obj1 = { orange: "juice", apple: "sauce", sauce: "pan" }; console.log(keyValueDuplicates (obj1)); // ["sauce"] obj2 = { big: "foot", foot: "ball", ball: "boy", boy: "scout"};console.log(keyValueDuplicates(obj2)); // ["foot", "ball", "boy"] obj3 = { pizza: "pie", apple: "pie" pumpkin: "pie"); console.log(keyValueDuplicates (obj3)); // [] *******************************************************************/function keyValueDuplicates (obj) { | // Your code here } /*****DO NOT MODIFY ANYTHING UNDER THIS LINE*********/ try { | module.exports = keyValueDuplicates; } catch (e) { | module.exports = null;}arrow_forward
- C++ Language Please add an execution chart for this code like the example below. I have provided the code and the example execution chart. : JUST NEED EXECUTION CHARTTT. Thanks Sample Execution Chart Template//your header files// constant size of the array// declare an array of BankAccount objects called accountsArray of size =SIZE// comments for other declarations with variable names etc and their functionality// function prototypes// method to fill array from file, details of input and output values and purpose of functionvoid fillArray (ifstream &input,BankAccount accountsArray[]);// method to find the largest account using balance as keyint largest(BankAccount accountsArray[]);// method to find the smallest account using balance as keyint smallest(BankAccount accountsArray[]);// method to display all elements of the accounts arrayvoid printArray(BankAccount accountsArray[]);int main() {// function calls come here:// give the function call and a comment about the purpose,//…arrow_forwardC++ Language Please add an execution chart for this code like the example below. I have provided the code and the example execution chart. : JUST NEED EXECUTION CHARTTT. Thanks Sample Execution Chart Template//your header files// constant size of the array// declare an array of BankAccount objects called accountsArray of size =SIZE// comments for other declarations with variable names etc and their functionality// function prototypes// method to fill array from file, details of input and output values and purpose of functionvoid fillArray (ifstream &input,BankAccount accountsArray[]);// method to find the largest account using balance as keyint largest(BankAccount accountsArray[]);// method to find the smallest account using balance as keyint smallest(BankAccount accountsArray[]);// method to display all elements of the accounts arrayvoid printArray(BankAccount accountsArray[]);int main() {// function calls come here:// give the function call and a comment about the purpose,//…arrow_forwardC++ Language Please add an execution chart for this code like the example below. I have provided the code and the example execution chart. : JUST NEED EXECUTION CHARTTT. Thanks Sample Execution Chart Template//your header files// constant size of the array// declare an array of BankAccount objects called accountsArray of size =SIZE// comments for other declarations with variable names etc and their functionality// function prototypes// method to fill array from file, details of input and output values and purpose of functionvoid fillArray (ifstream &input,BankAccount accountsArray[]);// method to find the largest account using balance as keyint largest(BankAccount accountsArray[]);// method to find the smallest account using balance as keyint smallest(BankAccount accountsArray[]);// method to display all elements of the accounts arrayvoid printArray(BankAccount accountsArray[]);int main() {// function calls come here:// give the function call and a comment about the purpose,//…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
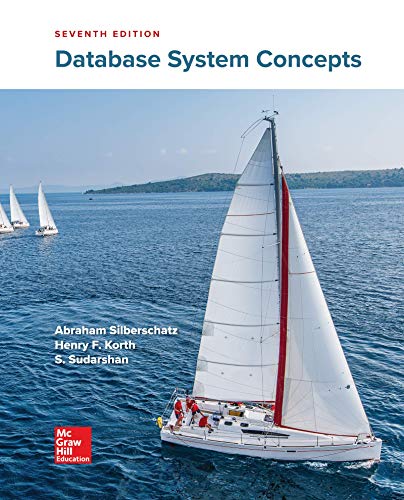
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
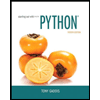
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
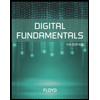
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
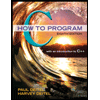
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
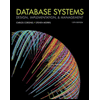
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
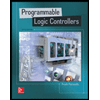
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education