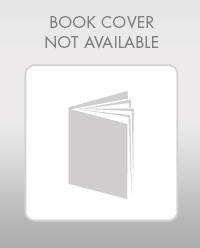
Starting Out With C++: Early Objects (10th Edition)
10th Edition
ISBN: 9780135235003
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 10, Problem 34RQE
Program Plan Intro
Pointer:
Pointer, the name itself references the purpose of the pointer. Pointers point to a location in memory.
- Pointer is a special type of variable to store the address of the memory location, which can be accessed later.
- If an asterisk “*” operator is present before the variable, then that variable is referred as pointer variable.
- It is also called as dereferencing or indirection operator.
- Pointer is just a type of variable that stores the addresses of other variables.
- Using pointers, we can access the address of a variable; the data stored in that variable can be retrieved.
Syntax of pointer variable declaration:
<variable-type> *<variable-name>;
Example for pointer variable declaration:
//definition of pointer variable
int *ptrvar;
Where,
- “int” is the variable type.
- “* ptrvar” is the pointer variable name.
Pointer will allow the user to indirectly access and manipulate the data contents of the variable. A pointer variable will hold the address of the data contents.
Note:
- When the symbol “&” is placed prior the pointer variable, it will hold address of the pointer variable.
- When the symbol “*” is placed prior the pointer variable, it will hold the value of the pointer variable.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Consider the function
void change(int *p)
{
*p = 20;
}Show how to call the change function so that it sets the integer variable int i;to 20.
In c++
int p =5 , q =6;
void foo ( int b , int c ) {
b = 2 * c ;
p = p + c ;
c = 1 + p ;
q = q * 2;
print ( b + c );
}
main () {
foo (p , q );
print p , q ;
}
Explain and print the output of the above code when the parameters to the foo function are passed by value. Explain and print the output of the above code when the parameters to the foo function are passed by reference. Explain and print the output of the above code when the parameters to the foo function are passed by value result. Explain and print the output of the above code when the parameters to the foo function are passed by name.
Chapter 10 Solutions
Starting Out With C++: Early Objects (10th Edition)
Ch. 10.5 - Prob. 10.1CPCh. 10.5 - Write a statement defining a variable dPtr. The...Ch. 10.5 - List three uses of the symbol in C++.Ch. 10.5 - What is the output of the following program?...Ch. 10.5 - Rewrite the following loop so it uses pointer...Ch. 10.5 - Prob. 10.6CPCh. 10.5 - Assume pint is a pointer variable. For each of the...Ch. 10.5 - For each of the following variable definitions,...Ch. 10.10 - Assuming array is an array of ints, which of the...Ch. 10.10 - Give an example of the proper way to call the...
Ch. 10.10 - Complete the following program skeleton. When...Ch. 10.10 - Look at the following array definition: const int...Ch. 10.10 - Assume ip is a pointer to an int. Write a...Ch. 10.10 - Assume ip is a pointer to an int. Write a...Ch. 10.10 - Prob. 10.15CPCh. 10.10 - Prob. 10.16CPCh. 10.10 - Prob. 10.17CPCh. 10.12 - Prob. 10.18CPCh. 10.12 - Assume the following structure declaration exists...Ch. 10.12 - Prob. 10.20CPCh. 10 - Each byte in memory is assigned a unique _____Ch. 10 - The _____ operator can be used to determine a...Ch. 10 - Prob. 3RQECh. 10 - The _____ operator can be used to work with the...Ch. 10 - Prob. 5RQECh. 10 - Creating variables while a program is running is...Ch. 10 - Prob. 7RQECh. 10 - If the new operator cannot allocate the amount of...Ch. 10 - Prob. 9RQECh. 10 - When a program is finished with a chunk of...Ch. 10 - You should only use the delete operator to...Ch. 10 - What does the indirection operator do?Ch. 10 - Look at the following code. int X = 7; int ptr =...Ch. 10 - Name two different uses for the C++ operator.Ch. 10 - Prob. 15RQECh. 10 - Prob. 16RQECh. 10 - Prob. 17RQECh. 10 - What is the purpose of the new operator?Ch. 10 - What happens when a program uses the new operator...Ch. 10 - Prob. 20RQECh. 10 - Prob. 21RQECh. 10 - Prob. 22RQECh. 10 - Prob. 23RQECh. 10 - Prob. 24RQECh. 10 - Prob. 25RQECh. 10 - Prob. 26RQECh. 10 - What happens when a unique_ptr that is managing an...Ch. 10 - What does the get ( ) method of the unique_ptr...Ch. 10 - Prob. 29RQECh. 10 - Prob. 30RQECh. 10 - Prob. 31RQECh. 10 - Prob. 32RQECh. 10 - Consider the function void change(int p) { P = 20;...Ch. 10 - Prob. 34RQECh. 10 - Write a function whose prototype is void...Ch. 10 - Write a function void switchEnds(int array, int...Ch. 10 - Given the variable initializations int a[5] = {0,...Ch. 10 - Each of the following declarations and program...Ch. 10 - Prob. 39RQECh. 10 - Test Scores #1 Write a program that dynamically...Ch. 10 - Test Scores #2 Modify the program of Programming...Ch. 10 - Indirect Sorting Through Pointers #1 Consider a...Ch. 10 - Indirect Sorting Through Pointers #2 Write a...Ch. 10 - Pie a la Mode In statistics the mode of a set of...Ch. 10 - Median Function In statistics the median of a set...Ch. 10 - Movie Statistics Write a program that can be used...Ch. 10 - Days in Current Month Write a program that can...Ch. 10 - Age Write a program that asks for the users name...Ch. 10 - Prob. 10PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- void getChar(char& c, istream& infile, int& i){ infile.get(c); if (c == '\n') i++;} COULD YOU REWRITTE THE FUNCTION ABOVE. AND BY REWRITE. I MEAN CHANGE THE FUNCTION NAME, PARAMETERS, AND ITS IMPLEMENTATION. HOWEVER, THE FUNCTION MUST STILL ACHEIVE THE SAME GOAL.arrow_forwardConsider the following function. int my_func(int a) { return(a+3); } If the function is called using my_func(5), what will the function return?arrow_forwardIN C++arrow_forward
- Homework ● Write a function C++ void switchEnds(int *array, int size); that is passed the address of the beginning of an array and the size of the array. The function swaps the values in the first and last entries of the array.arrow_forwardC PROGRAM Reverse + Random Formula In the code editor, there's already an initial code that contains the function declaration of the function, computeReverseNumber(int n) and an empty main() function. For this problem, you are task to implement the computeReverseNumber function. This function will take in an integer n as its parameter. This will get the reverse of the passed integer and will then compute and return the value: result = (original_number + reverse_of_the_number) / 3 In the main() function, ask the user to input the value of n, call the function computeReverseNumber and pass the inputted value of n, and print the result in the main() with two (2) decimal places. SAMPLE: Input n: 20 Output: 7.33 Input n: 123 Output: 148.00arrow_forwardIn C++ Write the definition of a void function that has two parameters: an array, and an integer parameter that specifies the number of elements in the array. The functions swaps the first and last elements of the array.arrow_forward
- In C++, define a “Conflict” function that accepts an array of Course object pointers. It will return two numbers:- the count of courses with conflicting days. If there is more than one course scheduled in the same day, it is considered a conflict. It will return if there is no conflict.- which day of the week has the most conflict. It will return 0 if there is none. Show how this function is being called and returning proper values. you may want to define a local integer array containing the count for each day of the week with the initial value of 0.Whenever you have a Course object with a specific day, you can increment that count for that corresponding index in the schedule array.arrow_forwardin C++ mathematical functions languagearrow_forwardWhat does the function f do? struct Point2D { double x; double y; struct Triangle { Point2D v1; Point2D v2; Point2D v3; }; void f(Triangle&t) { } int temp = 12.5; temp = t.v1.x; t.v1.x = t.v1.y; t.v1.y = temp; } int main () { Triangle mytri; mytri.v1.x = 1.0; mytri.v1.y = 22.5; f (mytri); Swaps values of x and y in vertex 1 of an argument of type Triangle Initializes value of x in vertex 1 of an argument of type Triangle Sets all x,y values in all vertices of an argument of type Triangle Swaps value of x in vertex 1 with value of x in vertex 2, for an argument of typearrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
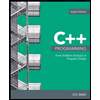
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning