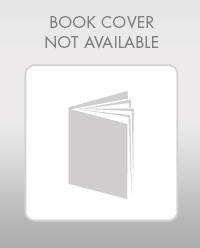
Complete the following program skeleton. When finished, the program should ask the user for a length (in inches), convert that value to centimeters, and display the result. You are to write the function convert. (Note: 1 inch = 2.54 cm. Do not modify function main.)
#include <iostream>
#include <iomanip>
using namespace std;
// Write your function prototype here.
int main()
{
double measurement;
cout << "Enter a length in inches, and I will convert\n";
cout << "it to centimeters: ";
cin >> measurement;
convert(&measurement );
cout << setprecision(4);
cout << fixed << showpoint;
cout << "Value in centimeters: "<< measurement << endl;
return 0;
}
//
// Write the function convert here.
//

Want to see the full answer?
Check out a sample textbook solution
Chapter 10 Solutions
Starting Out With C++: Early Objects (10th Edition)
Additional Engineering Textbook Solutions
Modern Database Management (12th Edition)
Software Engineering (10th Edition)
Problem Solving with C++ (9th Edition)
Web Development and Design Foundations with HTML5 (9th Edition) (What's New in Computer Science)
Experiencing MIS
Computer Science: An Overview (12th Edition)
- C++ The function prototype of a function that recieves two integer values and computes their sum and difference is given as follows: void computeSum (int num1, int num2, int & sum, int & diff); write the statments to call this function to compute and print the sum and the differnce of 167 and 84.arrow_forwardCalling a function with multiple arguments requires careful order.arrow_forwardHow can we pass argument to a function by reference instead of pass by value?arrow_forward
- Computer sciencearrow_forwardwrite a function that prints your name and ID. include a function definition, declaration, and function callarrow_forwardLAB: User-Defined Functions: Miles to track laps One lap around a standard high-school running track is exactly 0.25 miles. Define a function named MilesToLaps that takes a float as a parameter, representing the number of miles, and returns a float that represents the number of laps.Then, write a main program that takes a number of miles as an input, calls function MilesToLaps() to calculate the number of laps, and outputs the number of laps. Ex: If the input is: 1.5 the output is: 6.0 Ex: If the input is: 2.2 the output is: 8.8 Your program should define and call a function: Function MilesToLaps(float userMiles) returns float userLaps Note: Remove all comments outside any functions before running the program.arrow_forward
- Note: Write programs in c language format use in programs (Printf & Scanf)arrow_forwardWrite a function that parses a decimal number into an octal number. The function header is as follows: int dec2Octal(const int& decimal) Write a test program that prompts the user to enter a decimal number, uses the dec2Octal function to parse it into an equivalent octal number and displays the octal number.arrow_forward1. What is this paramters that are only accessible in the body of the function, like all variables used in the function? 2. What is the part of the program that creates function? 3. It performs operations like union, difference and intersectionarrow_forward
- 1. Write function printGrade in void function to compute and output the course grade. The course score is passed as a parameter to the function printGrade. (The program will take 5 inputs for 5 subjects. And show the grade of each subject. And calculate the CGPA) TTTE Percentage Score Quality Point Renark Letter Grade 8e - 100 75 - 79 70- 74 65- 69 6e- 64 55- 59 se - 54 45 - 49 40 - 44 35 - 39 e. 34 Excellent Extrenely Good Very Good Good Fairly Good Satisfactory Quite Satisfactory Poor 4.00 3.67 3.33 3.00 2.67 2.33 2.e0 1.67 1.33 Very Poor Extremely Poor Fail 1.00 Expected Output: Inputs 1. Mark for the programming is: 2. Mark for the Math is: 80 85 3. Mark for the Database System is: 4. Mark for the Software Engineering is: 5. Mark for the Business Fundamental is: 74 76 65 Output Letter Grade for Programming: A Letter Grade for Math: A Letter Grade for Database System: Letter Grade for Software Engineering: Letter Grade for Business Fundamental: Remark: B+ A- в Extremely Good 2.…arrow_forwardC language for solution Note: soution this program without use Struct 1. Write a program to do the following: a. Write a Cover Page function to print the HW details passing the needed parameters and has no return. b. Write a function that gets student grades and ID (maximum number of students is 20) then the function has a switch statement to enable the user to choose one of the following operation: i. Call MaxMin function to find and print the maximum and minimum grades as well as their ID ii. Call function Average to return the average grade of the class. iii. Call mark function to print students ID, their grades, and marks (as in the below table) iv. Call Sort function to sort the student ascending or descending based on their grades. The program will repeat the operation tile the user wish to terminate the program. Note: Student's grades are between 0 and 100 (use data validation technique). • You will get zero if you don't use comments and print HW details (your name, course name,…arrow_forwardC++ The function declaration part of this program is missing. Your job is to insert the function prototypes for all user-defined functions into the program. First, decide how many and what are the user-defined functions (exclude the main function) in this program. Then, declare the function prototype of each function in the appropriate place in the program.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
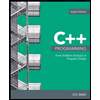