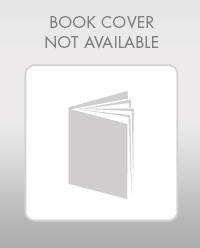
Starting Out With C++: Early Objects (10th Edition)
10th Edition
ISBN: 9780135235003
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 10, Problem 32RQE
Program Plan Intro
Shared pointers:
- A “shared_ptr” is a smart pointer which maintains shared ownership of an object using pointer.
- Shared pointers are used in managing the ownership of the dynamically allocated object.
- Shared pointers are used by more than one owner available for the objects that are allocated dynamically.
Example:
The following code illustrates the use and creation of shared pointer:
//creating a shared pointer of integer data type
shared_ptr<int> sDPtr1 (new int);
//creating an array of shared pointer
shared_ptr<int[]> sDPtr2 (new int[5]);
Explanation:
In the above code snippet, a smart pointer of integer data type is assigned directly.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
using r language to answer question 4. Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.
using r language
using r language
Chapter 10 Solutions
Starting Out With C++: Early Objects (10th Edition)
Ch. 10.5 - Prob. 10.1CPCh. 10.5 - Write a statement defining a variable dPtr. The...Ch. 10.5 - List three uses of the symbol in C++.Ch. 10.5 - What is the output of the following program?...Ch. 10.5 - Rewrite the following loop so it uses pointer...Ch. 10.5 - Prob. 10.6CPCh. 10.5 - Assume pint is a pointer variable. For each of the...Ch. 10.5 - For each of the following variable definitions,...Ch. 10.10 - Assuming array is an array of ints, which of the...Ch. 10.10 - Give an example of the proper way to call the...
Ch. 10.10 - Complete the following program skeleton. When...Ch. 10.10 - Look at the following array definition: const int...Ch. 10.10 - Assume ip is a pointer to an int. Write a...Ch. 10.10 - Assume ip is a pointer to an int. Write a...Ch. 10.10 - Prob. 10.15CPCh. 10.10 - Prob. 10.16CPCh. 10.10 - Prob. 10.17CPCh. 10.12 - Prob. 10.18CPCh. 10.12 - Assume the following structure declaration exists...Ch. 10.12 - Prob. 10.20CPCh. 10 - Each byte in memory is assigned a unique _____Ch. 10 - The _____ operator can be used to determine a...Ch. 10 - Prob. 3RQECh. 10 - The _____ operator can be used to work with the...Ch. 10 - Prob. 5RQECh. 10 - Creating variables while a program is running is...Ch. 10 - Prob. 7RQECh. 10 - If the new operator cannot allocate the amount of...Ch. 10 - Prob. 9RQECh. 10 - When a program is finished with a chunk of...Ch. 10 - You should only use the delete operator to...Ch. 10 - What does the indirection operator do?Ch. 10 - Look at the following code. int X = 7; int ptr =...Ch. 10 - Name two different uses for the C++ operator.Ch. 10 - Prob. 15RQECh. 10 - Prob. 16RQECh. 10 - Prob. 17RQECh. 10 - What is the purpose of the new operator?Ch. 10 - What happens when a program uses the new operator...Ch. 10 - Prob. 20RQECh. 10 - Prob. 21RQECh. 10 - Prob. 22RQECh. 10 - Prob. 23RQECh. 10 - Prob. 24RQECh. 10 - Prob. 25RQECh. 10 - Prob. 26RQECh. 10 - What happens when a unique_ptr that is managing an...Ch. 10 - What does the get ( ) method of the unique_ptr...Ch. 10 - Prob. 29RQECh. 10 - Prob. 30RQECh. 10 - Prob. 31RQECh. 10 - Prob. 32RQECh. 10 - Consider the function void change(int p) { P = 20;...Ch. 10 - Prob. 34RQECh. 10 - Write a function whose prototype is void...Ch. 10 - Write a function void switchEnds(int array, int...Ch. 10 - Given the variable initializations int a[5] = {0,...Ch. 10 - Each of the following declarations and program...Ch. 10 - Prob. 39RQECh. 10 - Test Scores #1 Write a program that dynamically...Ch. 10 - Test Scores #2 Modify the program of Programming...Ch. 10 - Indirect Sorting Through Pointers #1 Consider a...Ch. 10 - Indirect Sorting Through Pointers #2 Write a...Ch. 10 - Pie a la Mode In statistics the mode of a set of...Ch. 10 - Median Function In statistics the median of a set...Ch. 10 - Movie Statistics Write a program that can be used...Ch. 10 - Days in Current Month Write a program that can...Ch. 10 - Age Write a program that asks for the users name...Ch. 10 - Prob. 10PC
Knowledge Booster
Similar questions
- using r language Obtain a bootstrap t confidence interval estimate for the correlation statistic in Example 8.2 (law data in bootstrap).arrow_forwardusing r language Compute a jackknife estimate of the bias and the standard error of the correlation statistic in Example 8.2.arrow_forwardusing r languagearrow_forward
- using r languagearrow_forwardThe assignment here is to write an app using a database named CIT321 with a collection named students; we will provide a CSV file of the data. You need to use Vue.js to display 2 pages. You should know that this assignment is similar, all too similar in fact, to the cars4sale2 example in the lecture notes for Vue.js 2. You should study that program first. If you figure out cars4sale2, then program 6 will be extremely straightforward. It is not my intent do drop a ton of new material here in the last few days of class. The database contains 51 documents. The first rows of the CSV file look like this: sid last_name 1 Astaire first_name Humphrey CIT major hrs_attempted gpa_points 10 34 2 Bacall Katharine EET 40 128 3 Bergman Bette EET 42 97 4 Bogart Cary CIT 11 33 5 Brando James WEB 59 183 6 Cagney Marlon CIT 13 40 GPA is calculated as gpa_points divided by hrs_attempted. GPA points would have been arrived at by adding 4 points for each credit hour of A, 3 points for each credit hour of…arrow_forwardI need help to solve the following case, thank youarrow_forward
- You will write a program that allows the user to keep track of college locations and details about each location. To begin you will create a College python class that keeps track of the csollege's unique id number, name, address, phone number, maximum students, and average tuition cost. Once you have built the College class, you will write a program that stores College objects in a dictionary while using the College's unique id number as the key. The program should display a menu in this order that lets the user: 1) Add a new College 2) Look up a College 4) Delete an existing College 5) Change an existing College's name, address, phone number, maximum guests, and average tuition cost. 6) Exit the programarrow_forwardShow all the workarrow_forwardConstruct a frequency polygon density estimate for the sample in Question 1, using bin width determined by Sturges’ Rule.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
- Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
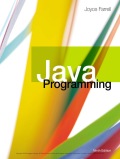
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
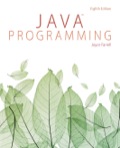
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
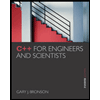
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
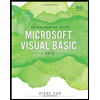
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
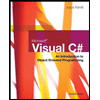
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage