Write a java program that has: A class RentedVehicle that has: - One private instance variable baseFee of type double - One constructor to initialize the instance variable - One instance method getCost () that returns the base fee - Accessor methods for the instance variables A subclass FuelVehicle that : - has one additional private instance variable Kms indicating the total number of kilometers traveled. - one constructor to initialize the instance variables. - one instance method getMileageFees to return the fees due to mileage based on the following: If Kms < 100 mileagefees=0.2*kms If 100<=Kms<= 400 mileagefees=0.3*kms If Kms>400 mileagefees=0.3 times 400 plus 0.5 times the extra kilometers above 400. - accessor methods A Car class which is a subclass of FuelVehicle that : - has one additional private instance variable nbSeats - has one constructor to initialize the instance variables - overrides getCost method by adding nbseats*baseFee to mileageFees - accessors A Truck class which is a subclass of FuelVehicle that: - has one private instance variable capacity - has one constructor to initialize the instance variables - overrides getCost method by adding baseFee*capacity to mileageFees - accessors A Bicycle class that extends RentedVehicle that: - has one additional private instance variable nDays indicating the number of days it is rented. - has one constructor to initialize the instance variables - overrides getCost method to return baseFee * nDays - accessors Implement all five classes with their accessor and mutator methods. Write an application class that generates 3 objects; one each from Car, or a Truck, or a Bicycle class. Your generated objects will be stored in a RentedVehicle array. Write a static method in your test class that takes an array of RentedVehicles and prints the following for each element of the array: - if the object is a car print its name and the number of seats and cost. - if it is a Truck, print its name and capacity and cost. - if it is a Bicycle, print its name and how many days it is rented and cost. Test your class with appropriate data.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Write a java program that has:
A class RentedVehicle that has:
- One private instance variable baseFee of type double
- One constructor to initialize the instance variable
- One instance method getCost () that returns the base fee
- Accessor methods for the instance variables
A subclass FuelVehicle that :
- has one additional private instance variable Kms indicating the total number of kilometers traveled.
- one constructor to initialize the instance variables.
- one instance method getMileageFees to return the fees due to mileage based on the following:
If Kms < 100 mileagefees=0.2*kms
If 100<=Kms<= 400 mileagefees=0.3*kms
If Kms>400 mileagefees=0.3 times 400 plus 0.5 times the extra kilometers above 400.
- accessor methods
A Car class which is a subclass of FuelVehicle that :
- has one additional private instance variable nbSeats
- has one constructor to initialize the instance variables
- overrides getCost method by adding nbseats*baseFee to mileageFees
- accessors
A Truck class which is a subclass of FuelVehicle that:
- has one private instance variable capacity
- has one constructor to initialize the instance variables
- overrides getCost method by adding baseFee*capacity to mileageFees
- accessors
A Bicycle class that extends RentedVehicle that:
- has one additional private instance variable nDays indicating the number of days it is rented.
- has one constructor to initialize the instance variables
- overrides getCost method to return baseFee * nDays
- accessors
Implement all five classes with their accessor and mutator methods.
Write an application class that generates 3 objects; one each from Car, or a Truck, or a Bicycle class. Your generated
objects will be stored in a RentedVehicle array.
Write a static method in your test class that takes an array of RentedVehicles and prints the following for each element of
the array:
- if the object is a car print its name and the number of seats and cost.
- if it is a Truck, print its name and capacity and cost.
- if it is a Bicycle, print its name and how many days it is rented and cost.
Test your class with appropriate data.

Step by step
Solved in 7 steps with 1 images

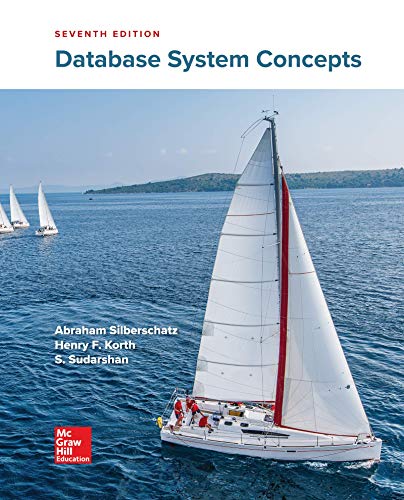
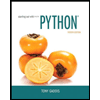
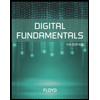
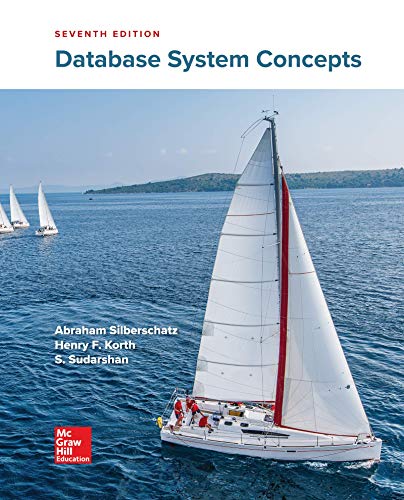
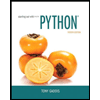
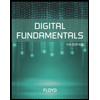
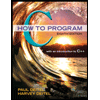
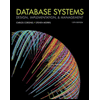
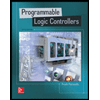