The Animal class has a default constructor with no parameters. Define a public overloaded constructor that takes two string parameters and an integer parameter for the type, the color, and the age of the animal. Ex: If the input is fox orange 5, then the output is: Animal: Unspecified, Unspecified, -1 Animal: fox, orange, 5 Animal.java public class Animal { privateStringtype; privateStringcolor; privateintage; publicAnimal() { // Default constructor type="Unspecified"; color="Unspecified"; age=-1; } /* Your code goes here */ publicvoidprint() { System.out.println("Animal: "+type+", "+color+", "+age); } } Zoo.java import java.util.Scanner; public class Zoo { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); String animalType; String animalColor; int animalAge; animalType = scnr.next(); animalColor = scnr.next(); animalAge = scnr.nextInt(); Animal emptyAnimal = new Animal(); Animal animal1 = new Animal(animalType, animalColor, animalAge); emptyAnimal.print(); animal1.print(); }}
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
The Animal class has a default constructor with no parameters. Define a public overloaded constructor that takes two string parameters and an integer parameter for the type, the color, and the age of the animal.
Ex: If the input is fox orange 5, then the output is:
Animal: Unspecified, Unspecified, -1 Animal: fox, orange, 5
import java.util.Scanner;
public class Zoo {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
String animalType;
String animalColor;
int animalAge;
animalType = scnr.next();
animalColor = scnr.next();
animalAge = scnr.nextInt();
Animal emptyAnimal = new Animal();
Animal animal1 = new Animal(animalType, animalColor, animalAge);
emptyAnimal.print();
animal1.print();
}
}
Unlock instant AI solutions
Tap the button
to generate a solution
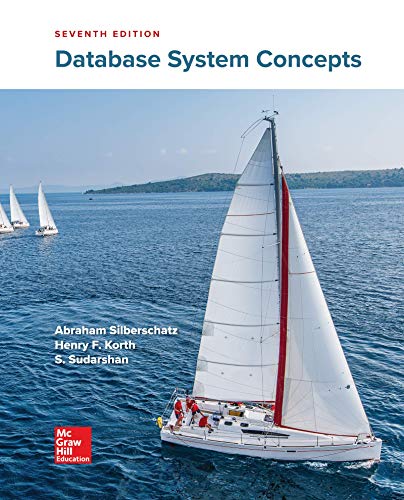
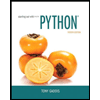
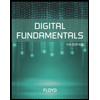
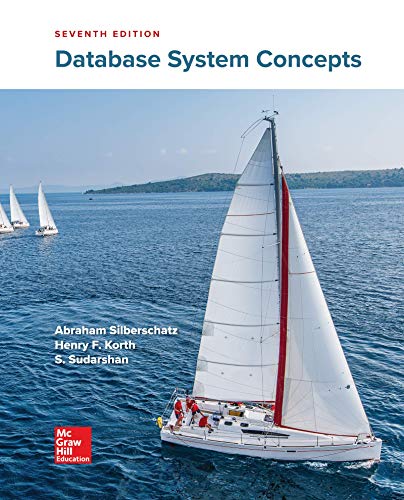
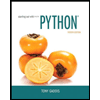
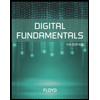
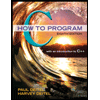
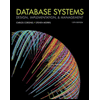
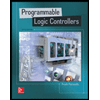