Write a java program that has: A class University that has: - Three private instance variables named firstName, lastName, and id - A constructor to initialize the three instance variables - A toString method to return: “Welcome,” + firstName +” “ + lastName + “Your ID is ” + id - Accessors A subclass Employee that has: - Five additional private instance variables named department, rate, bonus, hours, and salary - An appropriate constructor to use constructor of superclass in defining those of the subclass - A method named compteSalary ( ) to return salary - A method named computeBonus ( ) to return bonus - Accessors A subclass Staff that: - Overrides computeBonus ( ) such that if hours are greater than 40, there will be %10 bonus for each additional hour added to salary - Overrides compteSalary ( ) such that salary = (rate * hours) + bonus - Overrides toString method to add department and salary into the output A subclass Faculty that: - Overrides computeBonus ( ) such that if hours are greater than 40, there will be %25 bonus for each additional hour added to salary - Overrides compteSalary ( ) such that salary = (rate * hours) + bonus - Overrides toString method to add department and salary into the output A subclass Student that has: - Three additional private instance variables major, level, and grade - An appropriate constructor to use constructor of superclass in defining those of the subclass - One instance method computeGrade( ) to return the grade - Overrides toString method to add major and level into the output - Accessors A subclass Undergrade that has: - Three additional private instance variables named course1, course2, and course3 as double - An appropriate constructor to use constructor of superclass in defining those of the subclass - Overrides computeGade( ) such that grade = [(course1 + course2+ course3) / 300 ] * 100 - Overrides toString method to add grade into the output - Accessors A TestUniv class that has: - The main function to run your code. - Create objects to show two different outputs like: Output 1: Welcome, Ahmed Abdullah. Your ID is 123456 Your department is Computer Science and Engineering Your salary for this month is 10,000
Write a java program that has:
A class University that has:
- Three private instance variables named firstName, lastName, and id
- A constructor to initialize the three instance variables
- A toString method to return: “Welcome,” + firstName +” “ + lastName + “Your ID is ” + id
- Accessors
A subclass Employee that has:
- Five additional private instance variables named department, rate, bonus, hours, and salary
- An appropriate constructor to use constructor of superclass in defining those of the subclass
- A method named compteSalary ( ) to return salary
- A method named computeBonus ( ) to return bonus
- Accessors
A subclass Staff that:
- Overrides computeBonus ( ) such that if hours are greater than 40, there will be %10 bonus for each additional hour
added to salary
- Overrides compteSalary ( ) such that salary = (rate * hours) + bonus
- Overrides toString method to add department and salary into the output
A subclass Faculty that:
- Overrides computeBonus ( ) such that if hours are greater than 40, there will be %25 bonus for each additional hour
added to salary
- Overrides compteSalary ( ) such that salary = (rate * hours) + bonus
- Overrides toString method to add department and salary into the output
A subclass Student that has:
- Three additional private instance variables major, level, and grade
- An appropriate constructor to use constructor of superclass in defining those of the subclass
- One instance method computeGrade( ) to return the grade
- Overrides toString method to add major and level into the output
- Accessors
A subclass Undergrade that has:
- Three additional private instance variables named course1, course2, and course3 as double
- An appropriate constructor to use constructor of superclass in defining those of the subclass
- Overrides computeGade( ) such that grade = [(course1 + course2+ course3) / 300 ] * 100
- Overrides toString method to add grade into the output
- Accessors
A TestUniv class that has:
- The main function to run your code.
- Create objects to show two different outputs like:
Output 1:
Welcome, Ahmed Abdullah. Your ID is 123456
Your department is Computer Science and Engineering
Your salary for this month is 10,000

As per the given question, we need to create classes University, Employee, Staff, Faculty, Student, Undergrade, and TestUniv(This class tests the working of the remaining classes).
Attributes and methods of University class:
Attributes:
- firstName
- lastName
- id
Methods:
- Constructor
- Accessor methods i.e getter methods
- toString()
Attributes and methods of Employee class:
Attributes:
- firstName
- lastName
- id
- department
- rate
- bonus
- hours
- salary
Methods:
- Constructor
- Accessor methods i.e getter methods
- toString()
- compteSalary
- computeBonus
Attributes and methods of Staff class:
Attributes:
- firstName
- lastName
- id
- department
- rate
- bonus
- hours
- salary
Methods:
- Constructor
- toString()
- compteSalary
- computeBonus
Attributes and methods of Faculty class:
Attributes:
- firstName
- lastName
- id
- department
- rate
- bonus
- hours
- salary
Methods:
- Constructor
- toString()
- compteSalary
- computeBonus
Attributes and methods of Student class:
Attributes:
- firstName
- lastName
- id
- major
- level
- grade
Methods:
- Constructor
- toString()
- computeGrade
- Accessor methods
Attributes and methods of Undergrade class:
Attributes:
- firstName
- lastName
- id
- major
- level
- grade
- course1
- course2
- course3
Methods:
- Constructor
- toString()
- computeGrade
- Accessor methods
Step by step
Solved in 4 steps with 2 images

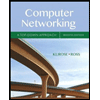
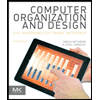
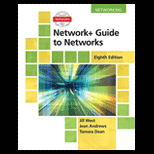
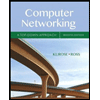
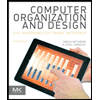
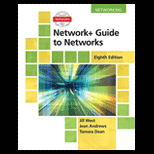
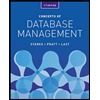
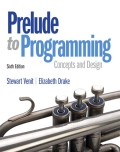
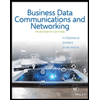