Implement a superclass named Car that contains a price instance variable, a getPrice method, and a 1-parameter constructor. The getPrice method is a simple accessor method that returns the price instance variable’s value. The 1-parameter constructor receives a cost parameter and assigns a value to the price instance variable based on this formula: price = cost * 2; Implement two classes named NewCar and UsedCar; they are both derived from the Car superclass. NewCar should contain a color instance variable (the car’s color). UsedCar should contain a mileage instance variable (the car’s odometer reading). The NewCar and UsedCar classes should each contain a 2-parameter constructor, an equals method, and a toString() method. In the interest of elegance and maintainability, don’t forget to have your subclass constructors call your superclass constructors when appropriate. The toString() method should print the values of all the instance variables within its class. Provide a driver class that tests your three car classes. Your driver class should contain this main method: public static void main(String[] args) { NewCar new1 = new NewCar(8000.33, "silver"); NewCar new2 = new NewCar(8000.33, "silver"); if (new1.equals(new2)) { System.out.println(new1); } UsedCar used1 = new UsedCar(2500, 100000); UsedCar used2 = new UsedCar(2500, 100000); if (used1.equals(used2)) { System.out.println(used1); } } // end main Output: price = $16,000.66, color = silver price = $5,000.00, mileage = 100,000
In Java
Your uncle is trying to keep track of his new-car and used-car lots by writing a Java program. He
needs your help in setting up his classes.
Implement a superclass named Car that contains a price instance variable, a getPrice method, and
a 1-parameter constructor. The getPrice method is a simple accessor method that returns the price
instance variable’s value. The 1-parameter constructor receives a cost parameter and assigns a
value to the price instance variable based on this formula:
price = cost * 2;
Implement two classes named NewCar and UsedCar; they are both derived from the Car
superclass. NewCar should contain a color instance variable (the car’s color). UsedCar should
contain a mileage instance variable (the car’s odometer reading). The NewCar and UsedCar
classes should each contain a 2-parameter constructor, an equals method, and a toString() method.
In the interest of elegance and maintainability, don’t forget to have your subclass constructors call
your superclass constructors when appropriate. The toString() method should print the values of
all the instance variables within its class.
Provide a driver class that tests your three car classes. Your driver class should contain this main
method:
public static void main(String[] args)
{
NewCar new1 = new NewCar(8000.33, "silver");
NewCar new2 = new NewCar(8000.33, "silver");
if (new1.equals(new2))
{
System.out.println(new1);
}
UsedCar used1 = new UsedCar(2500, 100000);
UsedCar used2 = new UsedCar(2500, 100000);
if (used1.equals(used2))
{
System.out.println(used1);
}
} // end main
Output:
price = $16,000.66, color = silver
price = $5,000.00, mileage = 100,000

Step by step
Solved in 3 steps with 1 images

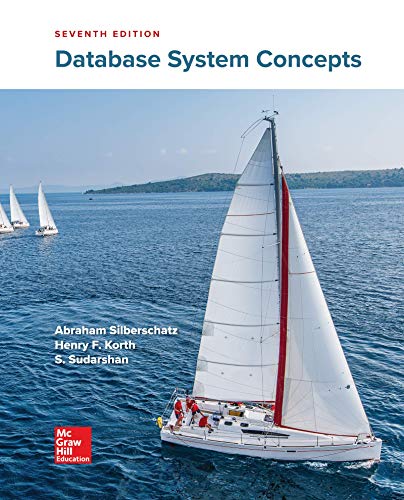
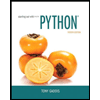
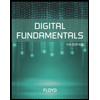
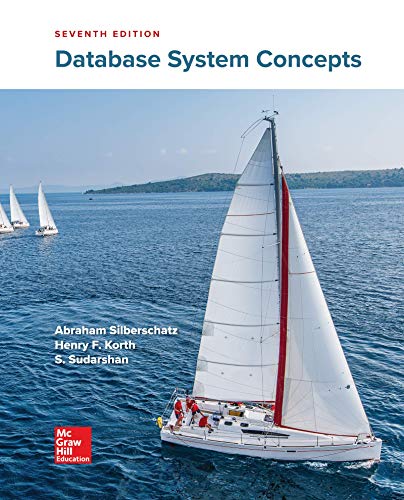
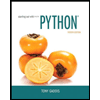
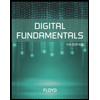
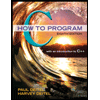
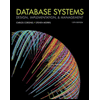
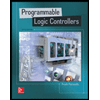