Using the objects you just created, as shown below, call the method to change the access level to the next in the sequence (in C++). CODE: #include using namespace std; class ParkingLot { private: bool Paid; bool Up; bool Down; public: ParkingLot() { Paid = false; Up = false; Down = true; } ParkingLot(bool paid, bool up) { Paid = paid; Up = up; Down = !up; } void togglePaid() { Paid = !Paid; if (Paid) { Up = true; Down = false; } else { Up = false; Down = true; } } bool isPaid() { return Paid; } bool isUp() { return Up; } bool isDown() { return Down; } }; int main() { // create a ParkingLot object using the default constructor ParkingLot lot1; // create a ParkingLot object using the overloaded constructor ParkingLot lot2(true, false); // display initial state of the barricades for both parking lots cout << "Parking Lot 1: Paid = " << lot1.isPaid() << ", Up = " << lot1.isUp() << ", Down = " << lot1.isDown() << endl; cout << "Parking Lot 2: Paid = " << lot2.isPaid() << ", Up = " << lot2.isUp() << ", Down = " << lot2.isDown() << endl; return 0; }
Using the objects you just created, as shown below, call the method to change the access level to the next in the sequence (in C++).
CODE:
#include <iostream>
using namespace std;
class ParkingLot {
private:
bool Paid;
bool Up;
bool Down;
public:
ParkingLot() {
Paid = false;
Up = false;
Down = true;
}
ParkingLot(bool paid, bool up) {
Paid = paid;
Up = up;
Down = !up;
}
void togglePaid() {
Paid = !Paid;
if (Paid) {
Up = true;
Down = false;
} else {
Up = false;
Down = true;
}
}
bool isPaid() {
return Paid;
}
bool isUp() {
return Up;
}
bool isDown() {
return Down;
}
};
int main() {
// create a ParkingLot object using the default constructor
ParkingLot lot1;
// create a ParkingLot object using the overloaded constructor
ParkingLot lot2(true, false);
// display initial state of the barricades for both parking lots
cout << "Parking Lot 1: Paid = " << lot1.isPaid() << ", Up = " << lot1.isUp() << ", Down = " << lot1.isDown() << endl;
cout << "Parking Lot 2: Paid = " << lot2.isPaid() << ", Up = " << lot2.isUp() << ", Down = " << lot2.isDown() << endl;
return 0;
}

Step by step
Solved in 2 steps

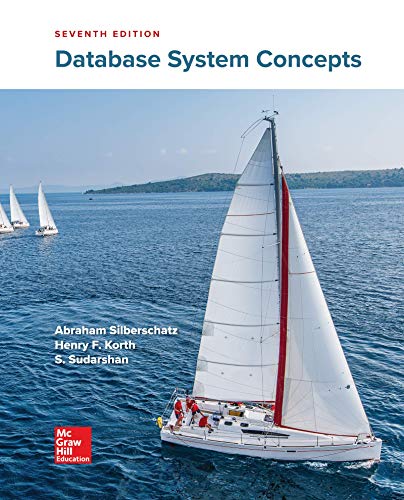
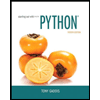
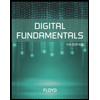
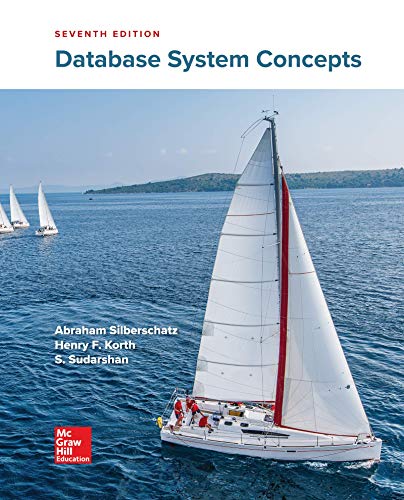
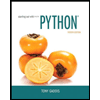
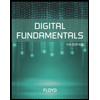
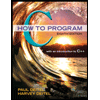
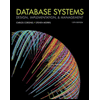
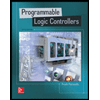