In C# using System; using static System.Console; using System.Globalization; class JobDemo4 { static void Main() { RushJob[] jobs = new RushJob[5]; int x, y; double grandTotal = 0; bool goodNum; for(x = 0 ; x < jobs.Length; ++x) { jobs[x] = new RushJob(); Write("Enter job number "); jobs[x].JobNumber = Convert.ToInt32(ReadLine()); goodNum = true; for(y = 0; y < x; ++y) { if(jobs[x].Equals(jobs[y])) goodNum = false; } while(!goodNum) { Write("Sorry, the job number " + jobs[x].JobNumber + " is a duplicate. " + "\nPlease reenter "); jobs[x].JobNumber = Convert.ToInt32(ReadLine()); goodNum = true; for(y = 0; y < x; ++y) { if(jobs[x].Equals(jobs[y])) goodNum = false; } } Write("Enter customer name "); jobs[x].Customer = ReadLine(); Write("Enter description "); jobs[x].Description = ReadLine(); Write("Enter estimated hours "); jobs[x].Hours = Convert.ToDouble(ReadLine()); } WriteLine("\nSummary:\n"); for(x = 0; x < jobs.Length; ++x) { WriteLine(jobs[x].ToString()); grandTotal += jobs[x].Price; } WriteLine("\nTotal for all jobs is " + grandTotal.ToString("C", CultureInfo.GetCultureInfo("en-US"))); } } class Job { protected double hours; protected double price; public const double RATE = 45.00; public Job(int num, string cust, string desc, double hrs) { JobNumber = num; Customer = cust; Description = desc; Hours = hrs; } public int JobNumber {get; set;} public string Customer {get; set;} public string Description {get; set;} public double Hours { get { return hours; } set { hours = value; price = hours * RATE; } } public double Price { get { return price; } } public override string ToString() { return(GetType() + " " + JobNumber + " " + Customer + " " + Description + " " + Hours + " hours @" + RATE.ToString("C") + " per hour. Total price is " + Price.ToString("C", CultureInfo.GetCultureInfo("en-US"))); } public override bool Equals(Object e) { bool equal; Job temp = (Job)e; if(JobNumber == temp.JobNumber) equal = true; else equal = false; return equal; } public override int GetHashCode() { return JobNumber; } } class RushJob : Job { public const double PREMIUM = 150.00; public RushJob() :base(0, "", "", 0) { } public override string ToString() { return(GetType() + " " + JobNumber + " " + Customer + " " + Description + " " + Hours + " hours @" + RATE.ToString("C") + " per hour. Rush job adds " + PREMIUM + " premium. Total price is " + Price.ToString("C", CultureInfo.GetCultureInfo("en-US"))); } public new double Hours { get { return hours; } set { hours = value; price = hours * RATE + PREMIUM; } } }
In C# using System; using static System.Console; using System.Globalization; class JobDemo4 { static void Main() { RushJob[] jobs = new RushJob[5]; int x, y; double grandTotal = 0; bool goodNum; for(x = 0 ; x < jobs.Length; ++x) { jobs[x] = new RushJob(); Write("Enter job number "); jobs[x].JobNumber = Convert.ToInt32(ReadLine()); goodNum = true; for(y = 0; y < x; ++y) { if(jobs[x].Equals(jobs[y])) goodNum = false; } while(!goodNum) { Write("Sorry, the job number " + jobs[x].JobNumber + " is a duplicate. " + "\nPlease reenter "); jobs[x].JobNumber = Convert.ToInt32(ReadLine()); goodNum = true; for(y = 0; y < x; ++y) { if(jobs[x].Equals(jobs[y])) goodNum = false; } } Write("Enter customer name "); jobs[x].Customer = ReadLine(); Write("Enter description "); jobs[x].Description = ReadLine(); Write("Enter estimated hours "); jobs[x].Hours = Convert.ToDouble(ReadLine()); } WriteLine("\nSummary:\n"); for(x = 0; x < jobs.Length; ++x) { WriteLine(jobs[x].ToString()); grandTotal += jobs[x].Price; } WriteLine("\nTotal for all jobs is " + grandTotal.ToString("C", CultureInfo.GetCultureInfo("en-US"))); } } class Job { protected double hours; protected double price; public const double RATE = 45.00; public Job(int num, string cust, string desc, double hrs) { JobNumber = num; Customer = cust; Description = desc; Hours = hrs; } public int JobNumber {get; set;} public string Customer {get; set;} public string Description {get; set;} public double Hours { get { return hours; } set { hours = value; price = hours * RATE; } } public double Price { get { return price; } } public override string ToString() { return(GetType() + " " + JobNumber + " " + Customer + " " + Description + " " + Hours + " hours @" + RATE.ToString("C") + " per hour. Total price is " + Price.ToString("C", CultureInfo.GetCultureInfo("en-US"))); } public override bool Equals(Object e) { bool equal; Job temp = (Job)e; if(JobNumber == temp.JobNumber) equal = true; else equal = false; return equal; } public override int GetHashCode() { return JobNumber; } } class RushJob : Job { public const double PREMIUM = 150.00; public RushJob() :base(0, "", "", 0) { } public override string ToString() { return(GetType() + " " + JobNumber + " " + Customer + " " + Description + " " + Hours + " hours @" + RATE.ToString("C") + " per hour. Rush job adds " + PREMIUM + " premium. Total price is " + Price.ToString("C", CultureInfo.GetCultureInfo("en-US"))); } public new double Hours { get { return hours; } set { hours = value; price = hours * RATE + PREMIUM; } } }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
In C#
using System;
using static System.Console;
using System.Globalization;
class JobDemo4
{
static void Main()
{
RushJob[] jobs = new RushJob[5];
int x, y;
double grandTotal = 0;
bool goodNum;
for(x = 0 ; x < jobs.Length; ++x)
{
jobs[x] = new RushJob();
Write("Enter job number ");
jobs[x].JobNumber = Convert.ToInt32(ReadLine());
goodNum = true;
for(y = 0; y < x; ++y)
{
if(jobs[x].Equals(jobs[y]))
goodNum = false;
}
while(!goodNum)
{
Write("Sorry, the job number " +
jobs[x].JobNumber + " is a duplicate. " +
"\nPlease reenter ");
jobs[x].JobNumber = Convert.ToInt32(ReadLine());
goodNum = true;
for(y = 0; y < x; ++y)
{
if(jobs[x].Equals(jobs[y]))
goodNum = false;
}
}
Write("Enter customer name ");
jobs[x].Customer = ReadLine();
Write("Enter description ");
jobs[x].Description = ReadLine();
Write("Enter estimated hours ");
jobs[x].Hours = Convert.ToDouble(ReadLine());
}
WriteLine("\nSummary:\n");
for(x = 0; x < jobs.Length; ++x)
{
WriteLine(jobs[x].ToString());
grandTotal += jobs[x].Price;
}
WriteLine("\nTotal for all jobs is " + grandTotal.ToString("C", CultureInfo.GetCultureInfo("en-US")));
}
}
class Job
{
protected double hours;
protected double price;
public const double RATE = 45.00;
public Job(int num, string cust, string desc, double hrs)
{
JobNumber = num;
Customer = cust;
Description = desc;
Hours = hrs;
}
public int JobNumber {get; set;}
public string Customer {get; set;}
public string Description {get; set;}
public double Hours
{
get
{
return hours;
}
set
{
hours = value;
price = hours * RATE;
}
}
public double Price
{
get
{
return price;
}
}
public override string ToString()
{
return(GetType() + " " + JobNumber + " " + Customer + " " +
Description + " " + Hours + " hours @" + RATE.ToString("C") +
" per hour. Total price is " + Price.ToString("C", CultureInfo.GetCultureInfo("en-US")));
}
public override bool Equals(Object e)
{
bool equal;
Job temp = (Job)e;
if(JobNumber == temp.JobNumber)
equal = true;
else
equal = false;
return equal;
}
public override int GetHashCode()
{
return JobNumber;
}
}
class RushJob : Job
{
public const double PREMIUM = 150.00;
public RushJob() :base(0, "", "", 0)
{
}
public override string ToString()
{
return(GetType() + " " + JobNumber + " " + Customer + " " +
Description + " " + Hours + " hours @" + RATE.ToString("C") +
" per hour. Rush job adds " + PREMIUM +
" premium. Total price is " + Price.ToString("C", CultureInfo.GetCultureInfo("en-US")));
}
public new double Hours
{
get
{
return hours;
}
set
{
hours = value;
price = hours * RATE + PREMIUM;
}
}
}

Transcribed Image Text:a. Create an application named JobDemo that declares and uses Job objects. The
Job class holds job information for a home repair service. The class has five
properties that include a job number, customer name, job description, estimated
hours, and price for the job. Create a constructor that requires parameters for all
the data except price. Include auto-implemented properties for the job number,
customer name, and job description, but not for hours or price; the price field value
is calculated as estimated hours times $45.00 whenever the hours value is set. Also
create the following for the class:
• An Equals() method that determines two Jobs are equal if they have the same
job number
• A GetHashCode () method that returns the job number
• A ToString() method that returns a string containing all job information
The JobDemo application declares a few Job objects, sets their values, and
demonstrates that all the methods work as expected.
b. Using the Job class you created in Exercise 3a, write a new application named
JobDemo2 that creates an array of five Job objects. Prompt the user for values for
each Job. Do not allow duplicate job numbers; force the user to reenter the job
when a duplicate job number is entered. When five valid objects have been entered,
display them all, plus a total of all prices.
c. Create a RushJob class that derives from Job. A RushJob has a $150.00 premium
that is added to the normal price of the job. Override any methods in the parent
class as necessary. Write a new application named JobDemo3 that creates an array
of five RushJobs. Prompt the user for values for each, and do not allow duplicate
job numbers. When five valid Rush Job objects have been entered, display them all,
plus a total of all prices.
d. Make any necessary modifications to the RushJob class so that it can be sorted by
job number. Modify the JobDemo3 application so the displayed orders have been
corted Saue the
lication
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 11 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
Recommended textbooks for you
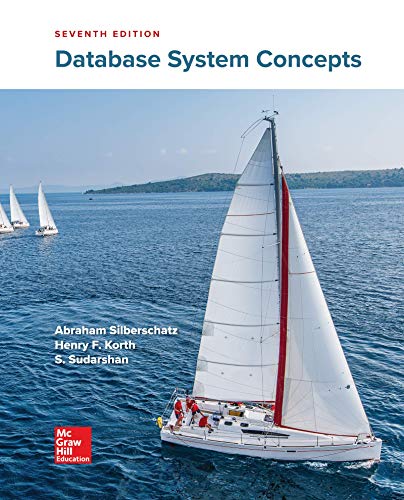
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
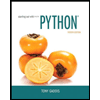
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
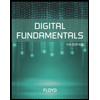
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
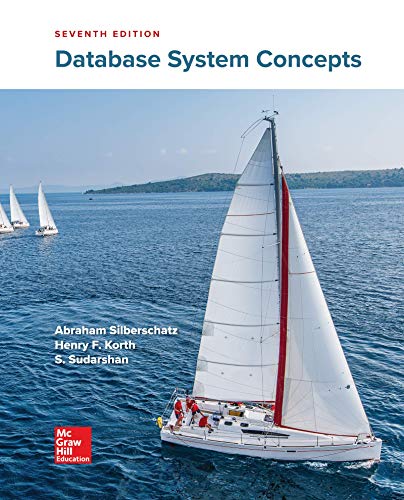
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
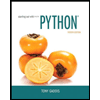
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
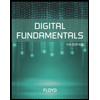
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
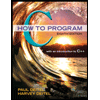
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
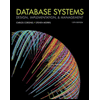
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
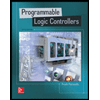
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education