You will build two classes, Mammal and Dog. Dog will inherit from Mammal. Below is the Mammal class code. Once you have the Mammal class built, build a second class Dog that will inherit publicly from Mammal. The Dog class should also override the move() and speak() methods from Mammal.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
in c++
-
You will build two classes, Mammal and Dog. Dog will inherit from Mammal. Below is the Mammal class code. Once you have the Mammal class built, build a second class Dog that will inherit publicly from Mammal. The Dog class should also override the move() and speak() methods from Mammal.
// Mammal.h
class Mammal
{
public:
Mammal();
~Mammal();
virtual void move() const;
virtual void speak() const;
protected:
int itsAge;
};
// Mammal.cpp
#include "Mammal.h"
Mammal::Mammal():itsAge(1)
{
cout << "Mammal constructor..." << endl;
}
Mammal::~Mammal()
{
cout << "Mammal destructor..." << endl;
}
void Mammal::move() const
{
cout << "Mammal moves a step!" << endl;
}
void Mammal::speak() const
{
cout << "What does a mammal speak? Mammilian!" << endl;
}
Once you have completed class Mammal and Dog, build the following main program.
#include "Mammal.h"
#include "Dog.h"
int main ()
{
Mammal *pDog = new Dog;
pDog->move();
pDog->speak();
//Dog *pDog2 = new Dog;
//pDog2->move();
//pDog2->speak();
delete pDog;
//delete pDog2;
return 0;
}
What does it output, and is that what you expected? Remove the keyword virtual from the Mammal class and try it again. Now what happens? Next, put in another pointer to pDog2 in the main program, but this time make it a pointer to a Dog, not a Mammal and create a new Dog (as seen in code comments). Now what happens? What you should realize is that by making the method speak() virtual, we can have a bit different behavior through dynamic (runtime) binding.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

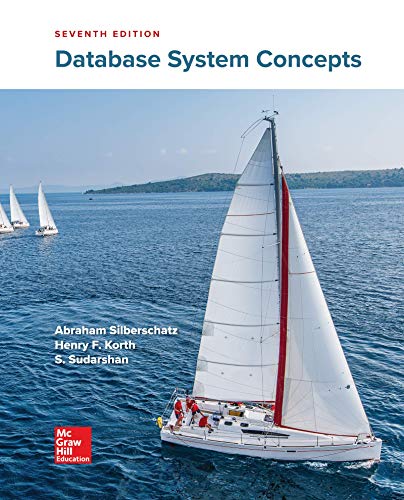
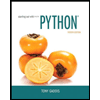
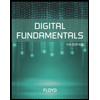
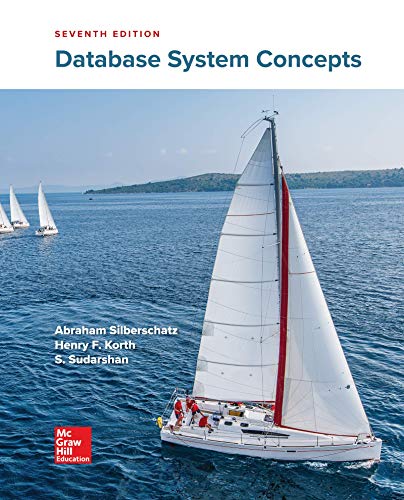
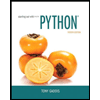
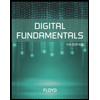
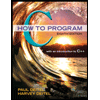
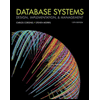
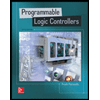