IN C++ Add a constructor to your class that you created in previous activities. A screenshot showing the code in Visual Studio and the output (on the console screen). previous activities code:- #include using namespace std; class HUMAN { public: string name; int age; }; class Student : public HUMAN { public: string major; int studentID; void printInfo() { cout << "Name: " << name << endl; cout << "Age: " << age << endl; cout << "Major: " << major << endl; cout << "Student ID: " << studentID << endl; } }; int main() { Student s; s.name = "John Doe"; s.age = 20; s.major = "Computer Science"; s.studentID = 12345; s.printInfo(); return 0; }
IN C++
Add a constructor to your class that you created in previous activities.
A screenshot showing the code in Visual Studio and the output (on the console screen).
previous activities code:-
#include <iostream>
using namespace std;
class HUMAN {
public:
string name;
int age;
};
class Student : public HUMAN {
public:
string major;
int studentID;
void printInfo() {
cout << "Name: " << name << endl;
cout << "Age: " << age << endl;
cout << "Major: " << major << endl;
cout << "Student ID: " << studentID << endl;
}
};
int main() {
Student s;
s.name = "John Doe";
s.age = 20;
s.major = "Computer Science";
s.studentID = 12345;
s.printInfo();
return 0;
}

Step by step
Solved in 3 steps with 3 images

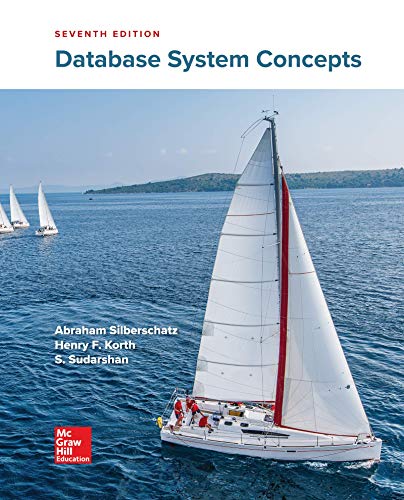
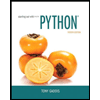
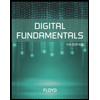
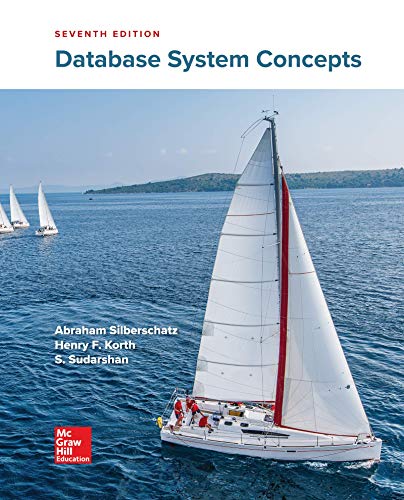
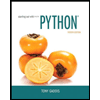
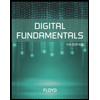
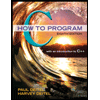
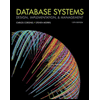
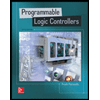