light assignment runs but there is no imput values for the user to interact when running the program. Kindly fix this issue. import java.util.*; class Flight { String airlineCode; int flightNumber; char type; // D for domestic, I for international String departureAirportCode; String departureGate; char departureDay; int departureTime; String arrivalAirportCode; String arrivalGate; char arrivalDay; int arrivalTime; char status; // S for scheduled, A for arrived, D for departed public Flight(String airlineCode, int flightNumber, char type, String departureAirportCode, String departureGate, char departureDay, int departureTime, String arrivalAirportCode, String arrivalGate, char arrivalDay, int arrivalTime) { this.airlineCode = airlineCode; this.flightNumber = flightNumber; this.type = type; this.departureAirportCode = departureAirportCode; this.departureGate = departureGate; this.departureDay = departureDay; this.departureTime = departureTime; this.arrivalAirportCode = arrivalAirportCode; this.arrivalGate = arrivalGate; this.arrivalDay = arrivalDay; this.arrivalTime = arrivalTime; this.status = 'S'; // Default status is scheduled } } class Airline { String name; String code; int firstClassCapacity; int businessClassCapacity; int economyClassCapacity; public Airline(String name, String code, int firstClassCapacity, int businessClassCapacity, int economyClassCapacity) { this.name = name; this.code = code; this.firstClassCapacity = firstClassCapacity; this.businessClassCapacity = businessClassCapacity; this.economyClassCapacity = economyClassCapacity; } } class FlightScheduler { static Scanner scanner = new Scanner(System.in); static List flights = new ArrayList<>(); static List airlines = new ArrayList<>(); static char currentDay; static int currentTime; public static void main(String[] args) { displayWelcomeScreen(); handleMenu(); } private static void displayWelcomeScreen() { System.out.println("Welcome to the Flight Scheduler!"); System.out.println("Please remember to always use U, M, T, W, R, F, S for entering the day of the week,"); System.out.println("and to always use military time for entering the time."); System.out.println("\nPlease make your choice by entering the corresponding menu number:"); } private static void handleMenu() { while (true) { System.out.println("\n1. Set Clock 2. Clear Schedule 3. Add Airline 4. Add Flight"); System.out.println("5. Cancel Flight 6. Show Flight Info 7. Show Departures 8. Show Arrivals 9. Find Flights Between Two Airports 10. Exit"); System.out.print("\nEnter your choice: "); int choice = scanner.nextInt(); scanner.nextLine(); // Consume the newline character switch (choice) { case 1: setClock(); break; case 2: clearSchedule(); break; case 3: addAirline(); break; case 4: addFlight(); break; case 10: System.out.println("Exiting program. Goodbye!"); System.exit(0); break; default: System.out.println("Invalid choice. Please try again."); } } } private static void setClock() { System.out.print("Enter U, M, T, W, R, F, or S for the corresponding day of the week: "); currentDay = scanner.next().charAt(0);
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Flight assignment runs but there is no imput values for the user to interact when running the program. Kindly fix this issue.
import java.util.*;
class Flight {
String airlineCode;
int flightNumber;
char type; // D for domestic, I for international
String departureAirportCode;
String departureGate;
char departureDay;
int departureTime;
String arrivalAirportCode;
String arrivalGate;
char arrivalDay;
int arrivalTime;
char status; // S for scheduled, A for arrived, D for departed
public Flight(String airlineCode, int flightNumber, char type, String departureAirportCode,
String departureGate, char departureDay, int departureTime,
String arrivalAirportCode, String arrivalGate, char arrivalDay, int arrivalTime) {
this.airlineCode = airlineCode;
this.flightNumber = flightNumber;
this.type = type;
this.departureAirportCode = departureAirportCode;
this.departureGate = departureGate;
this.departureDay = departureDay;
this.departureTime = departureTime;
this.arrivalAirportCode = arrivalAirportCode;
this.arrivalGate = arrivalGate;
this.arrivalDay = arrivalDay;
this.arrivalTime = arrivalTime;
this.status = 'S'; // Default status is scheduled
}
}
class Airline {
String name;
String code;
int firstClassCapacity;
int businessClassCapacity;
int economyClassCapacity;
public Airline(String name, String code, int firstClassCapacity, int businessClassCapacity, int economyClassCapacity) {
this.name = name;
this.code = code;
this.firstClassCapacity = firstClassCapacity;
this.businessClassCapacity = businessClassCapacity;
this.economyClassCapacity = economyClassCapacity;
}
}
class FlightScheduler {
static Scanner scanner = new Scanner(System.in);
static List<Flight> flights = new ArrayList<>();
static List<Airline> airlines = new ArrayList<>();
static char currentDay;
static int currentTime;
public static void main(String[] args) {
displayWelcomeScreen();
handleMenu();
}
private static void displayWelcomeScreen() {
System.out.println("Welcome to the Flight Scheduler!");
System.out.println("Please remember to always use U, M, T, W, R, F, S for entering the day of the week,");
System.out.println("and to always use military time for entering the time.");
System.out.println("\nPlease make your choice by entering the corresponding menu number:");
}
private static void handleMenu() {
while (true) {
System.out.println("\n1. Set Clock 2. Clear Schedule 3. Add Airline 4. Add Flight");
System.out.println("5. Cancel Flight 6. Show Flight Info 7. Show Departures 8. Show Arrivals 9. Find Flights Between Two Airports 10. Exit");
System.out.print("\nEnter your choice: ");
int choice = scanner.nextInt();
scanner.nextLine(); // Consume the newline character
switch (choice) {
case 1:
setClock();
break;
case 2:
clearSchedule();
break;
case 3:
addAirline();
break;
case 4:
addFlight();
break;
case 10:
System.out.println("Exiting program. Goodbye!");
System.exit(0);
break;
default:
System.out.println("Invalid choice. Please try again.");
}
}
}
private static void setClock() {
System.out.print("Enter U, M, T, W, R, F, or S for the corresponding day of the week: ");
currentDay = scanner.next().charAt(0);
System.out.print("Please enter the time in military notation: ");
currentTime = scanner.nextInt();
System.out.println("Day set to " + currentDay + " and time set to " + currentTime);
scanner.nextLine(); // Consume the newline character
System.out.print("Press Enter to continue...");
scanner.nextLine(); // Wait for Enter key
}
private static void clearSchedule() {
System.out.print("Are you sure you want to clear the schedule and start over? (y/n) ");
char response = scanner.next().charAt(0);
if (response == 'y' || response == 'Y') {
flights.clear();
System.out.println("Schedule cleared. Press Enter to continue...");
scanner.nextLine(); // Consume the newline character
scanner.nextLine(); // Wait for Enter key
}
}



Trending now
This is a popular solution!
Step by step
Solved in 3 steps

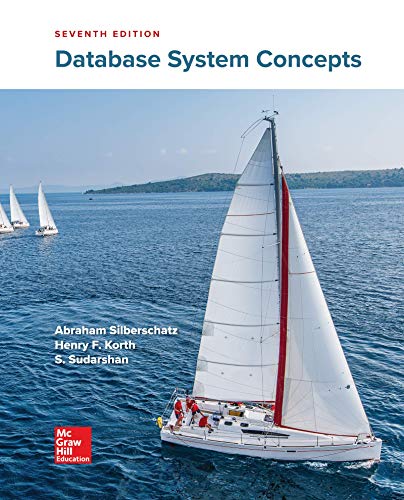
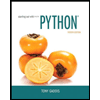
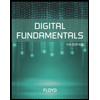
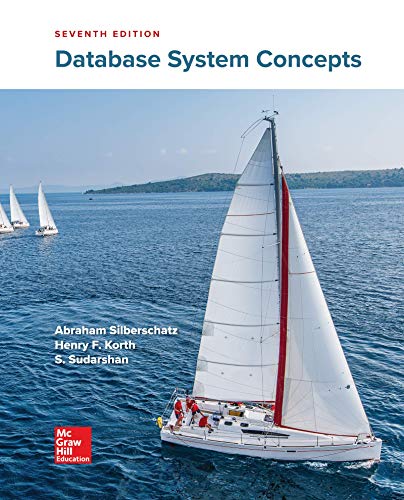
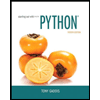
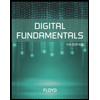
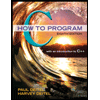
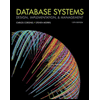
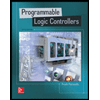