Use main.cxx and dnode.h to modify dnode.cxx to come out with the output: main.cxx: #include "dnode.h" int main() { dnode* head_ptr = NULL; int data[] = {5,9,11,2,3,8,7}; for (int i=0; i<(sizeof(data)/sizeof(int)); ++i) dlist_head_insert(head_ptr, data[i]); print_fore(head_ptr); dnode* cursor = dlist_search(head_ptr, 2); dlist_remove(cursor->back()); print_fore(head_ptr); dlist_head_remove(head_ptr); print_fore(head_ptr); print_back(head_ptr); dlist_head_remove(head_ptr); print_fore(head_ptr); dnode* cursor2 = dlist_search(head_ptr, 5); dlist_remove(cursor2->back()); print_fore(head_ptr); dnode* cursor3 = dlist_search(head_ptr, 9); dlist_insert(cursor3,13); print_fore(head_ptr); dlist_remove(head_ptr); print_fore(head_ptr); print_back(head_ptr); dlist_remove(head_ptr); print_fore(head_ptr); dlist_head_remove(head_ptr); print_fore(head_ptr); return 0; } dnode.h: #ifndef DNODE_H #define DNODE_H #include class dnode { public: typedef int value_type; dnode( const value_type& init_data = value_type( ), dnode* init_fore = NULL, dnode* init_back = NULL ) { data_field = init_data; link_fore = init_fore; link_back = init_back;} void set_data(const value_type& new_data) { data_field = new_data; } void set_fore(dnode* new_fore) { link_fore = new_fore; } void set_back(dnode* new_back) { link_back = new_back; } value_type data( ) const { return data_field; } const dnode* fore( ) const { return link_fore; } dnode* fore( ) { return link_fore; } const dnode* back( ) const { return link_back; } dnode* back( ) { return link_back; } private: value_type data_field; dnode* link_fore; dnode* link_back; }; void dlist_head_insert(dnode*& head_ptr, const dnode::value_type& entry); void dlist_insert(dnode* previous_ptr, const dnode::value_type& entry); void dlist_head_remove(dnode*& head_ptr); void dlist_remove(dnode* previous_ptr); dnode* dlist_search(dnode* head_ptr, const dnode::value_type& target); void print_fore(const dnode* head_ptr); void print_back(const dnode* head_ptr); #endif dnode.cxx: #include #include "dnode.h" void dlist_head_insert(dnode*& head_ptr, const dnode::value_type& entry) { } void dlist_insert(dnode* previous_ptr, const dnode::value_type& entry) { } void dlist_head_remove(dnode*& head_ptr) { } void dlist_remove(dnode* previous_ptr) { } dnode* dlist_search(dnode* head_ptr, const dnode::value_type& target) { } void print_fore(const dnode* head_ptr) { const dnode *cursor; for (cursor = head_ptr; cursor != NULL; cursor = cursor->fore()) std::cout << cursor->data() << " "; std::cout << std::endl; } void print_back(const dnode* head_ptr) { const dnode *cursor = head_ptr; while (cursor->fore() != NULL) cursor = cursor->fore(); for (;cursor != NULL; cursor = cursor->back()) std::cout << cursor->data() << " "; std::cout << std::endl; } Output: 7 8 3 2 11 9 5 7 8 3 11 9 5 8 3 11 9 5 5 9 11 3 8 3 11 9 5 3 11 9 3 11 9 13 3 9 13 13 9 3 3 13 13
Use main.cxx and dnode.h to modify dnode.cxx to come out with the output:
main.cxx:
#include "dnode.h"
int main()
{
dnode* head_ptr = NULL;
int data[] = {5,9,11,2,3,8,7};
for (int i=0; i<(sizeof(data)/sizeof(int)); ++i)
dlist_head_insert(head_ptr, data[i]);
print_fore(head_ptr);
dnode* cursor = dlist_search(head_ptr, 2);
dlist_remove(cursor->back());
print_fore(head_ptr);
dlist_head_remove(head_ptr);
print_fore(head_ptr);
print_back(head_ptr);
dlist_head_remove(head_ptr);
print_fore(head_ptr);
dnode* cursor2 = dlist_search(head_ptr, 5);
dlist_remove(cursor2->back());
print_fore(head_ptr);
dnode* cursor3 = dlist_search(head_ptr, 9);
dlist_insert(cursor3,13);
print_fore(head_ptr);
dlist_remove(head_ptr);
print_fore(head_ptr);
print_back(head_ptr);
dlist_remove(head_ptr);
print_fore(head_ptr);
dlist_head_remove(head_ptr);
print_fore(head_ptr);
return 0;
}
dnode.h:
#ifndef DNODE_H
#define DNODE_H
#include <cstdlib>
class dnode
{
public:
typedef int value_type;
dnode(
const value_type& init_data = value_type( ),
dnode* init_fore = NULL,
dnode* init_back = NULL
)
{ data_field = init_data; link_fore = init_fore; link_back = init_back;}
void set_data(const value_type& new_data) { data_field = new_data; }
void set_fore(dnode* new_fore) { link_fore = new_fore; }
void set_back(dnode* new_back) { link_back = new_back; }
value_type data( ) const { return data_field; }
const dnode* fore( ) const { return link_fore; }
dnode* fore( ) { return link_fore; }
const dnode* back( ) const { return link_back; }
dnode* back( ) { return link_back; }
private:
value_type data_field;
dnode* link_fore;
dnode* link_back;
};
void dlist_head_insert(dnode*& head_ptr, const dnode::value_type& entry);
void dlist_insert(dnode* previous_ptr, const dnode::value_type& entry);
void dlist_head_remove(dnode*& head_ptr);
void dlist_remove(dnode* previous_ptr);
dnode* dlist_search(dnode* head_ptr, const dnode::value_type& target);
void print_fore(const dnode* head_ptr);
void print_back(const dnode* head_ptr);
#endif
dnode.cxx:
#include <iostream>
#include "dnode.h"
void dlist_head_insert(dnode*& head_ptr, const dnode::value_type& entry)
{
}
void dlist_insert(dnode* previous_ptr, const dnode::value_type& entry)
{
}
void dlist_head_remove(dnode*& head_ptr)
{
}
void dlist_remove(dnode* previous_ptr)
{
}
dnode* dlist_search(dnode* head_ptr, const dnode::value_type& target)
{
}
void print_fore(const dnode* head_ptr)
{
const dnode *cursor;
for (cursor = head_ptr; cursor != NULL; cursor = cursor->fore())
std::cout << cursor->data() << " ";
std::cout << std::endl;
}
void print_back(const dnode* head_ptr)
{
const dnode *cursor = head_ptr;
while (cursor->fore() != NULL)
cursor = cursor->fore();
for (;cursor != NULL; cursor = cursor->back())
std::cout << cursor->data() << " ";
std::cout << std::endl;
}
Output:
7 8 3 2 11 9 5
7 8 3 11 9 5
8 3 11 9 5
5 9 11 3 8
3 11 9 5
3 11 9
3 11 9 13
3 9 13
13 9 3
3 13
13

Step by step
Solved in 4 steps with 3 images

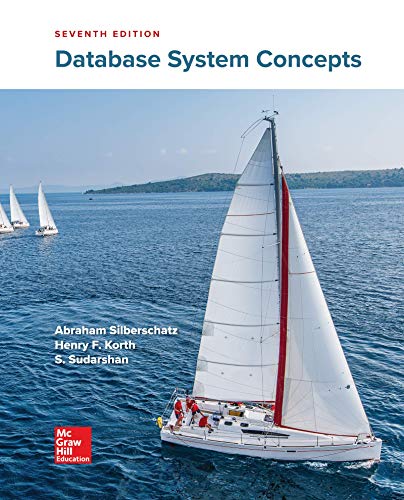
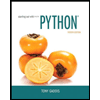
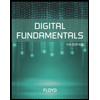
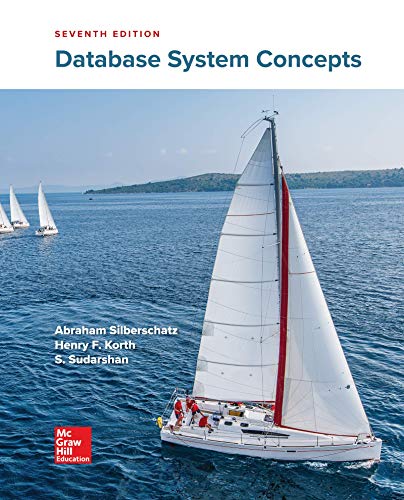
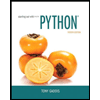
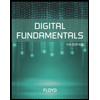
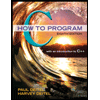
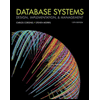
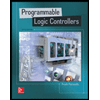