Question 10 Write both the move assignment operator and move constructor member functions for this templated CS30Vecto #include template class CS30Vector { public: // Constructs a vector size num CS30Vector(int num) {/*Implementation not shown*/} CS30Vector() {/*Implementation not shown*/} // your code will go here // additional member functions not shown private: T *arr=nullptr; // used to point to dynamic array int size; }; // number of elements in array void foo (CS3øVector v){/*Implementation not shown*/} int main ( ) { CS30Vector 11{6}; CS30Vector 12; // uses move assignment operator 12 = std::move(11); // uses move constructor below foo(CS30Vector(3)); return 0;
Question 10 Write both the move assignment operator and move constructor member functions for this templated CS30Vecto #include template class CS30Vector { public: // Constructs a vector size num CS30Vector(int num) {/*Implementation not shown*/} CS30Vector() {/*Implementation not shown*/} // your code will go here // additional member functions not shown private: T *arr=nullptr; // used to point to dynamic array int size; }; // number of elements in array void foo (CS3øVector v){/*Implementation not shown*/} int main ( ) { CS30Vector 11{6}; CS30Vector 12; // uses move assignment operator 12 = std::move(11); // uses move constructor below foo(CS30Vector(3)); return 0;
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question

Transcribed Image Text:### Question 10
**Task:** Write both the move assignment operator and move constructor member functions for this templated `CS30Vector` class.
```cpp
#include <iostream>
template <typename T>
class CS30Vector {
public:
// Constructs a vector size num
CS30Vector(int num) {/*Implementation not shown*/}
CS30Vector() {/*Implementation not shown*/}
// your code will go here
// additional member functions not shown
private:
T *arr=nullptr; // used to point to dynamic array
int size; // number of elements in array
};
void foo (CS30Vector<int> v){/*Implementation not shown*/}
int main ( ) {
CS30Vector<int> l1{6};
CS30Vector<int> l2;
// uses move assignment operator
l2 = std::move(l1);
// uses move constructor below
foo(CS30Vector<int>(3));
return 0;
}
```
### Explanation
This code provides a partially implemented template class `CS30Vector`, which represents a simple dynamic array (vector). Two constructors are declared but not implemented:
1. **Parameterized Constructor:** `CS30Vector(int num)` which initializes a vector of size `num`.
2. **Default Constructor:** `CS30Vector()` which serves as a default initializing constructor.
### Private Members
- **arr:** A pointer of type `T` (template type), intended to point to a dynamic array.
- **size:** An integer to store the number of elements in the array.
### Tasks to Implement
You are required to implement:
- **Move Assignment Operator:** Responsible for allowing an object to be moved rather than copied, useful to optimize performance when working with temporary objects.
- **Move Constructor:** An essential function to transfer resource ownership from one object to another (also optimizing performance), especially for temporary and rvalue objects.
### Usage in `main`
- **Move Assignment Operator** is demonstrated by:
```cpp
l2 = std::move(l1);
```
This line shows how the resources from `l1` are moved to `l2`.
- **Move Constructor** is utilized in:
```cpp
foo(CS30Vector<int>(3));
```
This line implicitly creates a temporary object of `CS30Vector<int>` which is then moved into the function `foo`.
This task
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
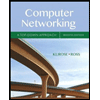
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
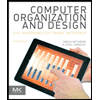
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
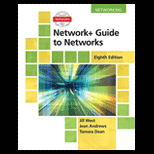
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
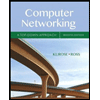
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
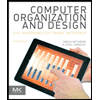
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
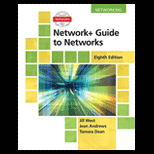
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
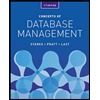
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
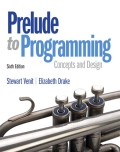
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
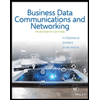
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY