myClassObject is an instance of MyClass. What is printed when myClassObject.doSomething(2); is run? public class MyClass { private int num; } public MyClass() { num= 5; 25 } public void doSomething (int num) { O 52 } an error will be thrown System.out.print(num); System.out.print(this.num); none of these is correct nothing because the MyClass class will not compile
myClassObject is an instance of MyClass. What is printed when myClassObject.doSomething(2); is run? public class MyClass { private int num; } public MyClass() { num= 5; 25 } public void doSomething (int num) { O 52 } an error will be thrown System.out.print(num); System.out.print(this.num); none of these is correct nothing because the MyClass class will not compile
Chapter9: Using Classes And Objects
Section: Chapter Questions
Problem 19RQ
Related questions
Question

Transcribed Image Text:myClassObject is an instance of MyClass. What is printed when myClassObject.doSomething(2); is
run?
public class MyClass {
private int num;
}
O 25
public MyClass() {
num = 5;
O 52
}
public void doSomething (int num) {
}
an error will be thrown
none of these is correct
System.out.print(num);
System.out.print(this.num);
nothing because the MyClass class will not compile

Transcribed Image Text:What happens when "this" is used in a constructor's body to call another constructor of the same
class but the call is not the first statement in the constructor?
For example:
public Student (String name, String id) {
id;
}
this.id
this (name);
=
a runtime error
O a logic error (meaning the program will run without error, but not work correctly)
nothing happens- the program compiles and runs fine
O a compiler error
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
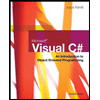
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
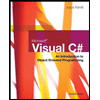
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,