Modify pipe4.cpp so that it accepts a message from the keyboard and sends it to pipe5. //pipe4.cpp (data producer) #include #include #include #include int main() { int data_processed; int file_pipes[2]; const char some_data[] = "123"; char buffer[BUFSIZ + 1]; pid_t fork_result; memset(buffer, '\0', sizeof(buffer)); if (pipe(file_pipes) == 0) { //creates pipe fork_result = fork(); if (fork_result == (pid_t)-1) { //fork fails fprintf(stderr, "Fork failure"); exit(EXIT_FAILURE); } if (fork_result == 0) { //child sprintf(buffer, "%d", file_pipes[0]); (void)execl("pipe5", "pipe5", buffer, (char *)0); exit(EXIT_FAILURE); } else { //parent data_processed = write(file_pipes[1], some_data, strlen(some_data)); printf("%d - wrote %d bytes\n", getpid(), data_processed); } } exit(EXIT_SUCCESS); }
Modify pipe4.cpp so that it accepts a message from the keyboard and sends it to pipe5.
//pipe4.cpp (data producer) #include <unistd.h> #include <stdlib.h> #include <stdio.h> #include <string.h>
int main() { int data_processed; int file_pipes[2]; const char some_data[] = "123"; char buffer[BUFSIZ + 1]; pid_t fork_result;
memset(buffer, '\0', sizeof(buffer));
if (pipe(file_pipes) == 0) { //creates pipe fork_result = fork(); if (fork_result == (pid_t)-1) { //fork fails fprintf(stderr, "Fork failure"); exit(EXIT_FAILURE); }
if (fork_result == 0) { //child sprintf(buffer, "%d", file_pipes[0]); (void)execl("pipe5", "pipe5", buffer, (char *)0); exit(EXIT_FAILURE); } else { //parent data_processed = write(file_pipes[1], some_data, strlen(some_data)); printf("%d - wrote %d bytes\n", getpid(), data_processed); } } exit(EXIT_SUCCESS); } |
// The 'consumer' program, pipe5.cpp, that reads the data is much simpler. #include <unistd.h> #include <stdlib.h> #include <stdio.h> #include <string.h> int main(int argc, char *argv[]) { int data_processed; char buffer[BUFSIZ + 1]; int file_descriptor; memset(buffer, '\0', sizeof(buffer)); sscanf(argv[1], "%d", &file_descriptor); data_processed = read(file_descriptor, buffer, BUFSIZ);
printf("%d - read %d bytes: %s\n", getpid(), data_processed, buffer); exit(EXIT_SUCCESS); }
|
Compile and execute:
$ g++ -o pipe4 pipe4.cpp $ g++ -o pipe5 pipe5.cpp $ ./pipe4
|
The output should look similar to the following.
6403 - wrote 3 bytes 6404 - read 3 bytes: 123
|

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

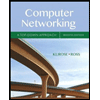
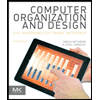
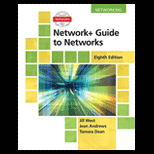
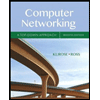
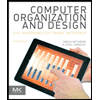
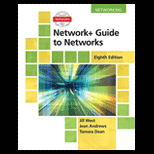
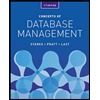
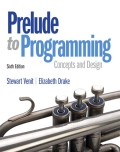
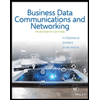