Make the isFull and isEmpty member functions private. Define a queue overflow exception and modify enqueue so that it throws this exception when the queue runs out of space. Define a queue underflow exception and modify dequeue so that it throws this exception when the queue is empty. Rewrite the main program so that it catches overflow exceptions when they occur. The exception handler for queue overflow should print an appropriate error message and then terminate the program. Here's the code so far: data.h: #pragma once class IntQueue { private: int *queueArray; int queueSize; int front; int rear; int numItems; public: IntQueue(int); ~IntQueue(); void enqueue(int); void dequeue(int &); bool isEmpty(); bool isFull(); void clear(); }; implementation: #include #include "Data.h" using namespace std; //************************* // Constructor * //************************* IntQueue::IntQueue(int s) { queueArray = new int[s]; queueSize = s; front = -1; rear = -1; numItems = 0; } //************************* // Destructor * //************************* IntQueue::~IntQueue() { delete [] queueArray; } //******************************************** // Function enqueue inserts the value in num * // at the rear of the queue. * //******************************************** void IntQueue::enqueue(int num) { if (isFull()) cout << "The queue is full.\n"; else { // Calculate the new rear position rear = (rear + 1) % queueSize; // Insert new item queueArray[rear] = num; // Update item count numItems++; } } //********************************************* // Function dequeue removes the value at the * // front of the queue, and copies t into num. * //********************************************* void IntQueue::dequeue(int &num) { if (isEmpty()) cout << "The queue is empty.\n"; else { // Move front front = (front + 1) % queueSize; // Retrieve the front item num = queueArray[front]; // Update item count numItems--; } } //********************************************* // Function isEmpty returns true if the queue * // is empty, and false otherwise. * //********************************************* bool IntQueue::isEmpty() { bool status; if (numItems) status = false; else status = true; return status; } //******************************************** // Function isFull returns true if the queue * // is full, and false otherwise. * //******************************************** bool IntQueue::isFull() { bool status; if (numItems < queueSize) status = false; else status = true; return status; } //******************************************* // Function clear resets the front and rear * // indices, and sets numItems to 0. * //******************************************* void IntQueue::clear() { front = queueSize - 1; rear = queueSize - 1; numItems = 0; } main: // This program demonstrates the IntQeue class #include #include "Data.h" using namespace std; int main() { IntQueue iQueue(5); cout << "Enqueuing 5 items...\n"; // Enqueue 5 items. for (int x = 0; x < 5; x++) iQueue.enqueue(x); // Attempt to enqueue a 6th item. cout << "Now attempting to enqueue again...\n"; iQueue.enqueue(5); // Deqeue and retrieve all items in the queue cout << "The values in the queue were:\n"; while (!iQueue.isEmpty()) { int value; iQueue.dequeue(value); cout << value << endl; } }
- Make the isFull and isEmpty member functions private.
- Define a queue overflow exception and modify enqueue so that it throws this exception when the queue runs out of space.
- Define a queue underflow exception and modify dequeue so that it throws this exception when the queue is empty.
- Rewrite the main program so that it catches overflow exceptions when they occur. The exception handler for queue overflow should print an appropriate error message and then terminate the program.
Here's the code so far:
data.h:
#pragma once
class IntQueue
{
private:
int *queueArray;
int queueSize;
int front;
int rear;
int numItems;
public:
IntQueue(int);
~IntQueue();
void enqueue(int);
void dequeue(int &);
bool isEmpty();
bool isFull();
void clear();
};
implementation:#include <iostream>
#include "Data.h"
using namespace std;//*************************
// Constructor *
//*************************
IntQueue::IntQueue(int s)
{
queueArray = new int[s];
queueSize = s;
front = -1;
rear = -1;
numItems = 0;
}//*************************
// Destructor *
//*************************IntQueue::~IntQueue()
{
delete [] queueArray;
}//********************************************
// Function enqueue inserts the value in num *
// at the rear of the queue. *
//********************************************void IntQueue::enqueue(int num)
{
if (isFull())
cout << "The queue is full.\n";
else
{
// Calculate the new rear position
rear = (rear + 1) % queueSize;
// Insert new item
queueArray[rear] = num;
// Update item count
numItems++;
}
}//*********************************************
// Function dequeue removes the value at the *
// front of the queue, and copies t into num. *
//*********************************************void IntQueue::dequeue(int &num)
{
if (isEmpty())
cout << "The queue is empty.\n";
else
{
// Move front
front = (front + 1) % queueSize;
// Retrieve the front item
num = queueArray[front];
// Update item count
numItems--;
}
}
//*********************************************
// Function isEmpty returns true if the queue *
// is empty, and false otherwise. *
//*********************************************bool IntQueue::isEmpty()
{
bool status;if (numItems)
status = false;
else
status = true;return status;
}//********************************************
// Function isFull returns true if the queue *
// is full, and false otherwise. *
//********************************************bool IntQueue::isFull()
{
bool status;if (numItems < queueSize)
status = false;
else
status = true;return status;
}
//*******************************************
// Function clear resets the front and rear *
// indices, and sets numItems to 0. *
//*******************************************void IntQueue::clear()
{
front = queueSize - 1;
rear = queueSize - 1;
numItems = 0;
}
main:
// This program demonstrates the IntQeue class
#include <iostream>
#include "Data.h"
using namespace std;int main()
{
IntQueue iQueue(5);cout << "Enqueuing 5 items...\n";
// Enqueue 5 items.
for (int x = 0; x < 5; x++)
iQueue.enqueue(x);// Attempt to enqueue a 6th item.
cout << "Now attempting to enqueue again...\n";
iQueue.enqueue(5);
// Deqeue and retrieve all items in the queue
cout << "The values in the queue were:\n";
while (!iQueue.isEmpty())
{
int value;
iQueue.dequeue(value);
cout << value << endl;
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

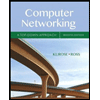
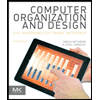
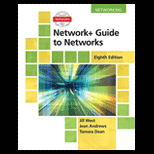
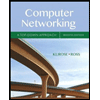
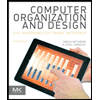
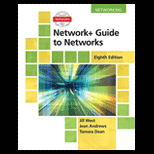
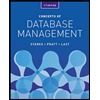
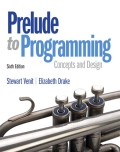
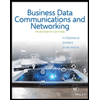