ow to answer the following question in java screen shot shows the ABList class Suppose a list names contains 8 elements. A call to names.set(8, "Albert") results in: A. an exception being thrown. B. an 8 element list with "Albert" as the last element. C. a 9 element list with "Albert" as the last element. D. a 9 element list with "Albert" as the next to last element. The only index-related method of the ABList class that can make good use of the protected find method is: A. add B. set C. get D. indexOf E. remove An application can indicate that it wants to use the "natural order" to order the elements of a SortedABList list by: A. passing the constructor an appropriate compareTo method. B. using the parameter-less constructor. C. using the standard list iteration approach. D. always adding elements to the front of the list. Suppose a list names contains 8 elements. A call to names.add(9, "Albert") results in: A. an exception being thrown. B. an 8 element list with "Albert" as the last element. C. a 9 element list with "Albert" as the last element. D. a 9 element list with null as the next to last element.
ow to answer the following question in java screen shot shows the ABList class Suppose a list names contains 8 elements. A call to names.set(8, "Albert") results in: A. an exception being thrown. B. an 8 element list with "Albert" as the last element. C. a 9 element list with "Albert" as the last element. D. a 9 element list with "Albert" as the next to last element. The only index-related method of the ABList class that can make good use of the protected find method is: A. add B. set C. get D. indexOf E. remove An application can indicate that it wants to use the "natural order" to order the elements of a SortedABList list by: A. passing the constructor an appropriate compareTo method. B. using the parameter-less constructor. C. using the standard list iteration approach. D. always adding elements to the front of the list. Suppose a list names contains 8 elements. A call to names.add(9, "Albert") results in: A. an exception being thrown. B. an 8 element list with "Albert" as the last element. C. a 9 element list with "Albert" as the last element. D. a 9 element list with null as the next to last element.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
how to answer the following question in java screen shot shows the ABList class
Suppose a list names contains 8 elements. A call to names.set(8, "Albert") results in:
A. an exception being thrown.
B. an 8 element list with "Albert" as the last element.
C. a 9 element list with "Albert" as the last element.
D. a 9 element list with "Albert" as the next to last element.
The only index-related method of the ABList class that can make good use of the protected find method is:
A. add
B. set
C. get
D. indexOf
E. remove
An application can indicate that it wants to use the "natural order" to order the elements of a SortedABList list by:
A. passing the constructor an appropriate compareTo method.
B. using the parameter-less constructor.
C. using the standard list iteration approach.
D. always adding elements to the front of the list.
Suppose a list names contains 8 elements. A call to names.add(9, "Albert") results in:
A. an exception being thrown.
B. an 8 element list with "Albert" as the last element.
C. a 9 element list with "Albert" as the last element.
D. a 9 element list with null as the next to last element.
![```java
//page 1
import java.util.Iterator;
public class AList<T> implements ListInterface<T>
{
protected final int DEFCAP = 100; // default capacity
protected int origCap; // original capacity
protected T[] elements; // array to hold this list’s elements
protected int numElements = 0; // number of elements in this list
// set by find method
protected boolean found; // true if target found, otherwise false
protected int location; // indicates location of target if found
public AList()
{
elements = (T[]) new Object[DEFCAP];
origCap = DEFCAP;
}
public AList(int origCap)
{
elements = (T[]) new Object[origCap];
this.origCap = origCap;
}
protected void enlarge()
// Increments the capacity of the list by an amount
// equal to the original capacity.
{
// Create the larger array.
T[] larger = (T[]) new Object[elements.length + origCap];
// Copy the contents from the smaller array into the larger array.
for (int i = 0; i < numElements; i++)
{
larger[i] = elements[i];
}
// Reassign elements reference.
elements = larger;
}
protected void find(T target)
// Searches list for an occurence of an element e such that
// e.equals(target). If successful, sets instance variables
// found to true and location to the array index of e. If
// not successful, sets found to false.
{
location = 0;
found = false;
while (location < numElements)
{
if (elements[location].equals(target))
{
found = true;
return;
}
else
location++;
}
}
public boolean add(T element)
// Adds element to end of this list.
{
if (numElements == elements.length)
enlarge();
elements[numElements] = element;
numElements++;
return true;
}
}
//page 2
{
public boolean remove (T target)
// Removes an element e from this list such that e.equals(target)
// and returns true; if no such element exists, returns false.
{](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1b2c1e36-9974-49eb-8a0c-fe4d3c635f06%2F2afbdcfc-a6fc-4aee-8cac-ca6ae00ba3bc%2Fvk624k3_processed.png&w=3840&q=75)
Transcribed Image Text:```java
//page 1
import java.util.Iterator;
public class AList<T> implements ListInterface<T>
{
protected final int DEFCAP = 100; // default capacity
protected int origCap; // original capacity
protected T[] elements; // array to hold this list’s elements
protected int numElements = 0; // number of elements in this list
// set by find method
protected boolean found; // true if target found, otherwise false
protected int location; // indicates location of target if found
public AList()
{
elements = (T[]) new Object[DEFCAP];
origCap = DEFCAP;
}
public AList(int origCap)
{
elements = (T[]) new Object[origCap];
this.origCap = origCap;
}
protected void enlarge()
// Increments the capacity of the list by an amount
// equal to the original capacity.
{
// Create the larger array.
T[] larger = (T[]) new Object[elements.length + origCap];
// Copy the contents from the smaller array into the larger array.
for (int i = 0; i < numElements; i++)
{
larger[i] = elements[i];
}
// Reassign elements reference.
elements = larger;
}
protected void find(T target)
// Searches list for an occurence of an element e such that
// e.equals(target). If successful, sets instance variables
// found to true and location to the array index of e. If
// not successful, sets found to false.
{
location = 0;
found = false;
while (location < numElements)
{
if (elements[location].equals(target))
{
found = true;
return;
}
else
location++;
}
}
public boolean add(T element)
// Adds element to end of this list.
{
if (numElements == elements.length)
enlarge();
elements[numElements] = element;
numElements++;
return true;
}
}
//page 2
{
public boolean remove (T target)
// Removes an element e from this list such that e.equals(target)
// and returns true; if no such element exists, returns false.
{
![The images display portions of a Java class implementation, likely part of a data structure class such as `ArrayList`. Below is a transcription of the provided code:
---
**Page 3**
```java
if (index < 0 || index > size())
throw new IndexOutOfBoundsException("Illegal index of " + index + " passed to AList add method.\n");
if (numElements == elements.length)
enlarge();
for (int i = numElements; i > index; i--)
elements[i] = elements[i - 1];
elements[index] = newElement;
numElements++;
```
```java
public T set(int index, T newElement)
// Throws IndexOutOfBoundsException if passed an index argument
// such that index < 0 or index >= size().
// Otherwise, replaces element on this list at position index with
// newElement and returns the replaced element.
{
if (index < 0 || index >= size())
throw new IndexOutOfBoundsException("Illegal index of " + index + " passed to AList set method.\n");
T hold = elements[index];
elements[index] = newElement;
return hold;
}
```
```java
public T get(int index)
// Throws IndexOutOfBoundsException if passed an index argument
// such that index < 0 or index >= size().
// Otherwise, returns the element on this list at position index.
{
if (index < 0 || index >= size())
throw new IndexOutOfBoundsException("Illegal index of " + index + " passed to AList get method.\n");
return elements[index];
}
```
```java
public int indexOf(T target)
// If this list contains an element e such that e.equals(target),
// then returns the index of the first such element.
// Otherwise, returns -1.
{
find(target);
if (found)
return location;
else
return -1;
}
```
```java
public T remove(int index)
// Throws IndexOutOfBoundsException if passed an index argument
// such that index < 0 or index >= size().
// Otherwise, removes element on this list at position index and
// returns the removed element; all current elements at positions
// higher than index have 1 subtracted from their position.
{
if (index < 0 || index >= size())
throw new IndexOutOfBoundsException("](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1b2c1e36-9974-49eb-8a0c-fe4d3c635f06%2F2afbdcfc-a6fc-4aee-8cac-ca6ae00ba3bc%2Fenmxywr_processed.png&w=3840&q=75)
Transcribed Image Text:The images display portions of a Java class implementation, likely part of a data structure class such as `ArrayList`. Below is a transcription of the provided code:
---
**Page 3**
```java
if (index < 0 || index > size())
throw new IndexOutOfBoundsException("Illegal index of " + index + " passed to AList add method.\n");
if (numElements == elements.length)
enlarge();
for (int i = numElements; i > index; i--)
elements[i] = elements[i - 1];
elements[index] = newElement;
numElements++;
```
```java
public T set(int index, T newElement)
// Throws IndexOutOfBoundsException if passed an index argument
// such that index < 0 or index >= size().
// Otherwise, replaces element on this list at position index with
// newElement and returns the replaced element.
{
if (index < 0 || index >= size())
throw new IndexOutOfBoundsException("Illegal index of " + index + " passed to AList set method.\n");
T hold = elements[index];
elements[index] = newElement;
return hold;
}
```
```java
public T get(int index)
// Throws IndexOutOfBoundsException if passed an index argument
// such that index < 0 or index >= size().
// Otherwise, returns the element on this list at position index.
{
if (index < 0 || index >= size())
throw new IndexOutOfBoundsException("Illegal index of " + index + " passed to AList get method.\n");
return elements[index];
}
```
```java
public int indexOf(T target)
// If this list contains an element e such that e.equals(target),
// then returns the index of the first such element.
// Otherwise, returns -1.
{
find(target);
if (found)
return location;
else
return -1;
}
```
```java
public T remove(int index)
// Throws IndexOutOfBoundsException if passed an index argument
// such that index < 0 or index >= size().
// Otherwise, removes element on this list at position index and
// returns the removed element; all current elements at positions
// higher than index have 1 subtracted from their position.
{
if (index < 0 || index >= size())
throw new IndexOutOfBoundsException("
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
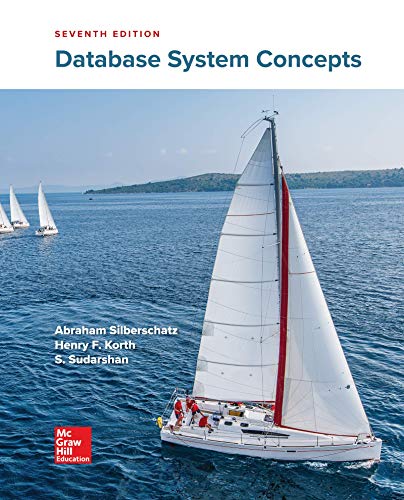
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
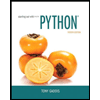
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
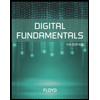
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
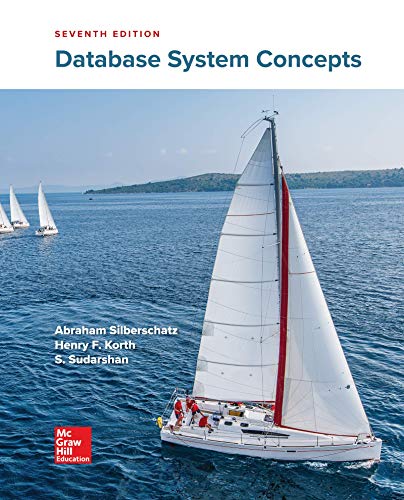
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
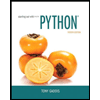
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
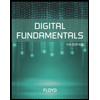
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
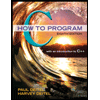
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
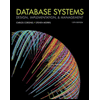
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
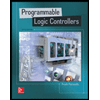
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education