Create a collection of ArrayList with a size of 100 String elements.
// The language is java, please take a screenshot of your output, and make sure your code is run.


a )Creation of collection of Array List
public class JExample{
public static void main(String args[]) {
//creating ArrayList of type String
ArrayList<String> obj = new ArrayList<String>();
/Add elements to an ArrayList
obj.add("Ajeeth");
obj.add("Harray");
obj.add("Chaitanya");
obj.add("steve");
obj.add("Anuj");
//Displaying elements
System.out.println("Original ArrayList:");
for(String str:obj)
System.out.println(str);
//Add elements at the index
obj.add(0,"Rahul");
obj.add(1,"Justin");
//Displaying elements
System.out.println("ArrayList after add operation:");
for(String str: obj)
System.out.println(str);
//Remove elements from ArrayList like this
obj.remove("Chaitanya");
obj.remove("Harry");
//Displaying elements
System.out.println("ArrayList after remove operation:");
for(String str : obj)
System.out.println(str);
//Remove a element from the specified index
obj.remove(1);
//Displaying elements
System.out.println("Final ArrayList:");
for(String str:obj)
System.out.println(str);
}
}
OUTPUT:
c ) Create a collection of linked list and initialize
import java.util.*;
public class JavaExample{
public static void main(String args[]) {
LinkedList<String> list= new LinkedList<String>();
//Adding elements to the Linked list
list.add("Steve");
list.add("Carl");
list.add("Raj");
//Adding an element to the first position
list.addFirst("Negan");
//Adding an element to the last position
list.addLast("Rick");
//Adding an element to the 3rd position
list.add(2,"Glenn");
//Iterating LinkedList
Iterator<String>iterator = list.iterator();
while(iterator.hasNext()) {
System.out.println(iterator.next());
}
}
}
OUTPUT:
Step by step
Solved in 3 steps with 2 images

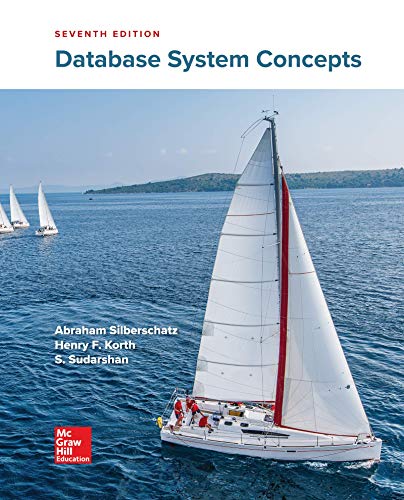
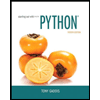
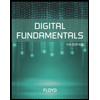
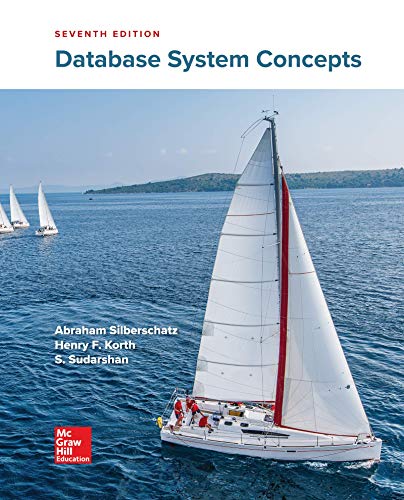
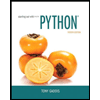
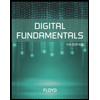
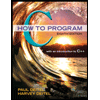
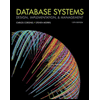
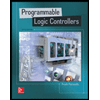