I am struggling to make the output to 2 decimal spaces and the error messages to show on the exception portions, when an improper input is made. public partial class Form1 : Form { public List list = new List(); public double totalGross = 0; public double totalNetPay = 0; public double totalSSWithheld = 0; public double totalMedicareWithHeld = 0; public double totalStateIncomeTaxWithheld = 0; public double totalFederalIncomeTaxWithheld = 0; public Form1() { InitializeComponent(); } private void button1_Click(object sender, EventArgs e) { try { for (int i = 0; i < list.Count; i++) richTextBox1.Text += "Employee: " + list[i].Name + "\n" + "Gross Pay: $" + list[i].GrossPay + "\n" + "Net Pay: $" + list[i].NetPay + "\n" + "SS Withheld: $" + list[i].SSWithheld + "\n" + "Medicare Withheld: $" + list[i].MedicareWithheld + "\n" + "State Tax Withheld: $" + list[i].StateIncomeTaxWithheld + "\n" + "Federal Tax Withheld: $" + list[i].FederalIncomeTaxWithheld; richTextBox1.Text += "\n" + "\n"; } catch (ArgumentException) { MessageBox.Show("Error! Please try again."); } catch(FormatException fex) { Console.WriteLine("Invalid format. Please try again."); Console.WriteLine(fex.StackTrace); } } private void button2_Click(object sender, EventArgs e) { try { Employee emp = new Employee(); emp.Name = textBox1.Text + " " + textBox2.Text; emp.GrossPay = (Convert.ToDouble(textBox3.Text) * Convert.ToDouble(textBox4.Text)); emp.MedicareWithheld = emp.GrossPay * 0.0145; emp.SSWithheld = emp.GrossPay * 0.062; //summary //Calculates State and Federal tax depending on gross pay //summary if (emp.GrossPay < 500 && emp.GrossPay > 0) { emp.StateIncomeTaxWithheld = (emp.GrossPay * 0.02); emp.FederalIncomeTaxWithheld = (emp.GrossPay * 0.05); } else if (emp.GrossPay < 999.99 && emp.GrossPay > 499.99) { emp.StateIncomeTaxWithheld = (emp.GrossPay * 0.04); emp.FederalIncomeTaxWithheld = (emp.GrossPay * 0.10); } else if (emp.GrossPay < 1499.99 && emp.GrossPay > 999.99) { emp.StateIncomeTaxWithheld = (emp.GrossPay * 0.06); emp.FederalIncomeTaxWithheld = (emp.GrossPay * 0.15); } else if (emp.GrossPay < 1999.99 && emp.GrossPay > 1499.99) { emp.StateIncomeTaxWithheld = (emp.GrossPay * 0.06); emp.FederalIncomeTaxWithheld = (emp.GrossPay * 0.20); } else if (emp.GrossPay < 2999.99 && emp.GrossPay > 1999.99) { emp.StateIncomeTaxWithheld = (emp.GrossPay * 0.06); emp.FederalIncomeTaxWithheld = (emp.GrossPay * 0.25); } else if (emp.GrossPay >= 3000) { emp.StateIncomeTaxWithheld = (emp.GrossPay * 0.06); emp.FederalIncomeTaxWithheld = (emp.GrossPay * 0.30); } emp.NetPay = emp.GrossPay - ((emp.MedicareWithheld) + (emp.SSWithheld) + (emp.StateIncomeTaxWithheld) + (emp.FederalIncomeTaxWithheld)); //summary //list Called in the loop //summary list.Add(emp); totalFederalIncomeTaxWithheld += emp.FederalIncomeTaxWithheld; totalGross += emp.GrossPay; totalMedicareWithHeld += emp.MedicareWithheld; totalNetPay += emp.NetPay; totalSSWithheld += emp.SSWithheld; totalStateIncomeTaxWithheld += emp.StateIncomeTaxWithheld; textBox1.Clear(); textBox2.Clear(); textBox3.Clear(); textBox4.Clear(); } catch (ArgumentException aex) { Console.WriteLine("Invalid input. Please try again."); Console.WriteLine(aex.StackTrace); } catch (FormatException fex) { Console.WriteLine("Invalid format. Please try again."); Console.WriteLine(fex.StackTrace); } } } } public class Employee { public string Name; public double GrossPay; public double NetPay; public double SSWithheld; public double MedicareWithheld; public double StateIncomeTaxWithheld; public double FederalIncomeTaxWithheld; }
I am struggling to make the output to 2 decimal spaces and the error messages to show on the exception portions, when an improper input is made.
public partial class Form1 : Form
{
public List<Employee> list = new List<Employee>();
public double totalGross = 0;
public double totalNetPay = 0;
public double totalSSWithheld = 0;
public double totalMedicareWithHeld = 0;
public double totalStateIncomeTaxWithheld = 0;
public double totalFederalIncomeTaxWithheld = 0;
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
try
{
for (int i = 0; i < list.Count; i++)
richTextBox1.Text += "Employee: " + list[i].Name + "\n" + "Gross Pay: $" + list[i].GrossPay +
"\n" + "Net Pay: $" + list[i].NetPay + "\n" + "SS Withheld: $" + list[i].SSWithheld +
"\n" + "Medicare Withheld: $" + list[i].MedicareWithheld + "\n" + "State Tax Withheld: $" +
list[i].StateIncomeTaxWithheld + "\n" + "Federal Tax Withheld: $" + list[i].FederalIncomeTaxWithheld;
richTextBox1.Text += "\n" + "\n"; }
catch (ArgumentException)
{
MessageBox.Show("Error! Please try again.");
}
catch(FormatException fex)
{
Console.WriteLine("Invalid format. Please try again.");
Console.WriteLine(fex.StackTrace);
}
}
private void button2_Click(object sender, EventArgs e)
{
try
{
Employee emp = new Employee();
emp.Name = textBox1.Text + " " + textBox2.Text;
emp.GrossPay = (Convert.ToDouble(textBox3.Text) * Convert.ToDouble(textBox4.Text));
emp.MedicareWithheld = emp.GrossPay * 0.0145;
emp.SSWithheld = emp.GrossPay * 0.062;
//summary
//Calculates State and Federal tax depending on gross pay
//summary
if (emp.GrossPay < 500 && emp.GrossPay > 0)
{
emp.StateIncomeTaxWithheld = (emp.GrossPay * 0.02);
emp.FederalIncomeTaxWithheld = (emp.GrossPay * 0.05);
}
else if (emp.GrossPay < 999.99 && emp.GrossPay > 499.99)
{
emp.StateIncomeTaxWithheld = (emp.GrossPay * 0.04);
emp.FederalIncomeTaxWithheld = (emp.GrossPay * 0.10);
}
else if (emp.GrossPay < 1499.99 && emp.GrossPay > 999.99)
{
emp.StateIncomeTaxWithheld = (emp.GrossPay * 0.06);
emp.FederalIncomeTaxWithheld = (emp.GrossPay * 0.15);
}
else if (emp.GrossPay < 1999.99 && emp.GrossPay > 1499.99)
{
emp.StateIncomeTaxWithheld = (emp.GrossPay * 0.06);
emp.FederalIncomeTaxWithheld = (emp.GrossPay * 0.20);
}
else if (emp.GrossPay < 2999.99 && emp.GrossPay > 1999.99)
{
emp.StateIncomeTaxWithheld = (emp.GrossPay * 0.06);
emp.FederalIncomeTaxWithheld = (emp.GrossPay * 0.25);
}
else if (emp.GrossPay >= 3000)
{
emp.StateIncomeTaxWithheld = (emp.GrossPay * 0.06);
emp.FederalIncomeTaxWithheld = (emp.GrossPay * 0.30);
}
emp.NetPay = emp.GrossPay - ((emp.MedicareWithheld) + (emp.SSWithheld) + (emp.StateIncomeTaxWithheld) +
(emp.FederalIncomeTaxWithheld));
//summary
//list Called in the loop
//summary
list.Add(emp);
totalFederalIncomeTaxWithheld += emp.FederalIncomeTaxWithheld;
totalGross += emp.GrossPay;
totalMedicareWithHeld += emp.MedicareWithheld;
totalNetPay += emp.NetPay;
totalSSWithheld += emp.SSWithheld;
totalStateIncomeTaxWithheld += emp.StateIncomeTaxWithheld;
textBox1.Clear();
textBox2.Clear();
textBox3.Clear();
textBox4.Clear();
}
catch (ArgumentException aex)
{
Console.WriteLine("Invalid input. Please try again.");
Console.WriteLine(aex.StackTrace);
}
catch (FormatException fex)
{
Console.WriteLine("Invalid format. Please try again.");
Console.WriteLine(fex.StackTrace);
}
}
}
}
public class Employee
{
public string Name;
public double GrossPay;
public double NetPay;
public double SSWithheld;
public double MedicareWithheld;
public double StateIncomeTaxWithheld;
public double FederalIncomeTaxWithheld;
}

Step by step
Solved in 5 steps with 3 images

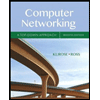
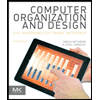
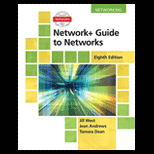
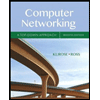
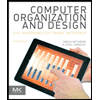
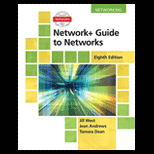
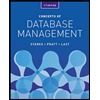
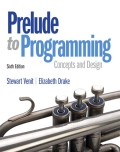
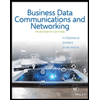