Write a method that removes the value of a node with index i from a singly-linked list. Also, you must raise exceptions when necessary.
Write a method that removes the value of a node with index i from a singly-linked list. Also, you must raise exceptions when necessary.
You must NOT use any other methods that **you implemented** in the assignment (everything must be done inline). (In python
Consider the following class definition:
class SLLException(Exception):
"""
Custom exception class to be used by Singly Linked List
DO NOT CHANGE THIS CLASS IN ANY WAY
"""
pass
class SLNode:
"""
Singly Linked List Node class
DO NOT CHANGE THIS CLASS IN ANY WAY
"""
def __init__(self, value: object) -> None:
self._next = None
self._value = value
class LinkedList:
def __init__(self, start_list=None):
"""
Initializes a new linked list with front and back sentinels
DO NOT CHANGE THIS METHOD IN ANY WAY
"""
self._head = SLNode(None)
self._tail = SLNode(None)
self._head._next = self._tail
# populate SLL with initial values (if provided)
# before using this feature, implement add_back() method
if start_list is not None:
for value in start_list:
self.add_back(value)
def __str__(self) -> str:
"""
Return content of singly linked list in human-readable form
DO NOT CHANGE THIS METHOD IN ANY WAY
"""
out = 'SLL ['
if self._head._next != self._tail:
cur = self._head._next._next
out = out + str(self._head._next._value)
while cur != self._tail:
out = out + ' -> ' + str(cur._value)
cur = cur._next
out = out + ']'
return out
def length(self) -> int:
"""
Return the length of the linked list
DO NOT CHANGE THIS METHOD IN ANY WAY
"""
length = 0
cur = self._head
while cur._next != self._tail:
cur = cur._next
length += 1
return length
def is_empty(self) -> bool:
"""
Return True is list is empty, False otherwise
DO NOT CHANGE THIS METHOD IN ANY WAY
"""
return self._head._next == self._tail
# ------------------------------------------------------------------ #
def remove_at_index(self, index: int) -> None :
"""
TODO: Write this implementation
"""
return

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

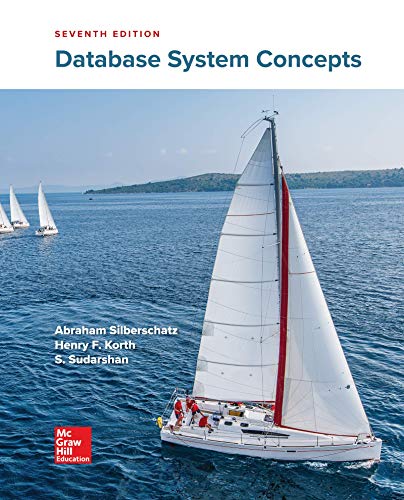
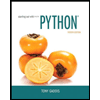
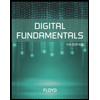
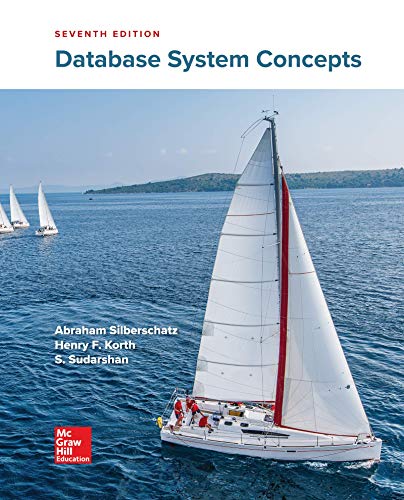
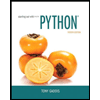
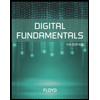
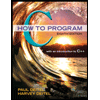
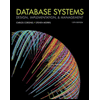
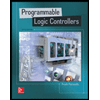