List manipulation with exception handling! In this assignment, you are given one list to perform different types of operations in each function. All the functions are provided, you need to find out the kind of exceptions that can happen while performing different operations on the elements and your task is to fill out the code with python exception handling mechanism wherever appropriate.
List manipulation with exception handling! In this assignment, you are given one list to perform different types of operations in each function. All the functions are provided, you need to find out the kind of exceptions that can happen while performing different operations on the elements and your task is to fill out the code with python exception handling mechanism wherever appropriate.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
make sure the code follows template code

Transcribed Image Text:# Topics: Handling exceptions, list
## List manipulation with exception handling!
In this assignment, you are given one list to perform different types of operations in each function. All the functions are provided, and you need to find out the kind of exceptions that can happen while performing different operations on the elements, and your task is to fill out the code with Python exception handling mechanisms wherever appropriate.
## Lab Scenario:
1. In this lab, a list is provided that you need to obtain from an input file. The elements of the list are separated with a comma. The code is provided to get the list from the file. The format of the file is as follows:
`1,2,3,"NA",0,10`
2. The following functions are provided. You need to fill out the exception handling blocks in the appropriate places.
| Function name | Parameter: data type | Return: data type | Description |
|-----------------------|----------------------|--------------------------------------|--------------------------------------------------------------------------------------------------------------------------------------------------------------|
| summation(sequence) | sequence: python list| Total summation of numeric elements: float | takes a list ‘sequence’ and returns the summation of the sequence. |
| reciprocal(n) | n: any python data type | Reciprocal of n if n is numeric otherwise returns a string: *"Zero and string not allowed"* | gets the list element n and returns the reciprocal of the number. For example: if n is a number then reciprocal of n is 1/n. |
| getlist(filename) | filename: string | Returns a list of elements if file can be opened otherwise returns False | stores the content of the file ‘filename’ in a list. raises an IOError if the file cannot be open. |
![```plaintext
def summation(sequence):
"""
Input: sequence: python list
Return: summation of the numeric elements: int or float
"""
total_sum = 0
for s in sequence:
total_sum += float(s)
return total_sum
def reciprocal(n):
"""
Input: n: int or float
Returns: reciprocal of n if n is int or float otherwise
returns error message
"""
return 1/float(n)
def getList(filename):
"""
Input: filename: string
Returns: list: content of the file in list
if file can be opened otherwise returns false
"""
f = open(filename)
temp = f.readline()
return [t.split('\n')[0] for t in temp.split(',')] # returns the sequence
def main():
sequence = getList('LA9.txt')
if sequence == False:
print ("File I/O error")
else:
print("The sequence is: ", sequence)
print ("**** Calculating Summation *****")
print (summation(sequence))
print (" **** Calculating Reciprocal *****")
for s in sequence:
print ('{} : {}'.format(s , reciprocal(s)))
6. For this lab, you are expected to use python exception handling mechanism try-except block. The
above functions are provided, you need to fill out the functions with try-except statements wherever needed. One sample code can be as follows:
W/O exception handling (user might enter W/o try-except block some non-number values)
x = input("Enter a number: ")
print ("You entered a number") try:
x = input("Enter a number: ")
print ("You entered a number")
except:
print ("Not a valid number. ")
Sample Output for the given Input file:
This is just a sample output, you can choose to print other messages as you like, but the code should
have exception handling implemented properly so that it gives you the right output.
The sequence is: ['1', '2', '3', '"NA"', '0', '10','100.0', '23']
**** Calculating Summation ****
Not a number, continue to next element
139.0
**** Calculating Reciprocal ****
1 : 1.0
2 : 0.5
3 : 0.333333333](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F9eb2eb60-aea6-4c2b-bdb3-b0b3ca45de12%2F1a2bb158-456c-487b-9d4e-dcc0037e696c%2Fbrr46c6_processed.png&w=3840&q=75)
Transcribed Image Text:```plaintext
def summation(sequence):
"""
Input: sequence: python list
Return: summation of the numeric elements: int or float
"""
total_sum = 0
for s in sequence:
total_sum += float(s)
return total_sum
def reciprocal(n):
"""
Input: n: int or float
Returns: reciprocal of n if n is int or float otherwise
returns error message
"""
return 1/float(n)
def getList(filename):
"""
Input: filename: string
Returns: list: content of the file in list
if file can be opened otherwise returns false
"""
f = open(filename)
temp = f.readline()
return [t.split('\n')[0] for t in temp.split(',')] # returns the sequence
def main():
sequence = getList('LA9.txt')
if sequence == False:
print ("File I/O error")
else:
print("The sequence is: ", sequence)
print ("**** Calculating Summation *****")
print (summation(sequence))
print (" **** Calculating Reciprocal *****")
for s in sequence:
print ('{} : {}'.format(s , reciprocal(s)))
6. For this lab, you are expected to use python exception handling mechanism try-except block. The
above functions are provided, you need to fill out the functions with try-except statements wherever needed. One sample code can be as follows:
W/O exception handling (user might enter W/o try-except block some non-number values)
x = input("Enter a number: ")
print ("You entered a number") try:
x = input("Enter a number: ")
print ("You entered a number")
except:
print ("Not a valid number. ")
Sample Output for the given Input file:
This is just a sample output, you can choose to print other messages as you like, but the code should
have exception handling implemented properly so that it gives you the right output.
The sequence is: ['1', '2', '3', '"NA"', '0', '10','100.0', '23']
**** Calculating Summation ****
Not a number, continue to next element
139.0
**** Calculating Reciprocal ****
1 : 1.0
2 : 0.5
3 : 0.333333333
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
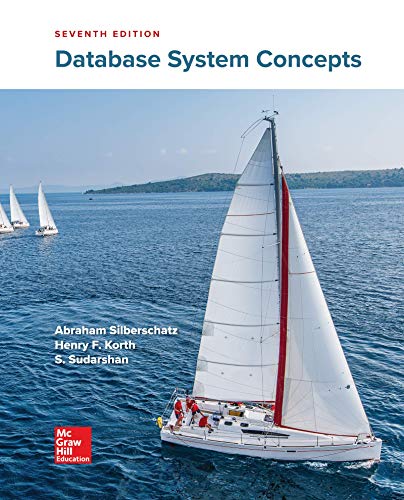
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
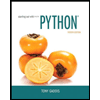
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
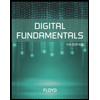
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
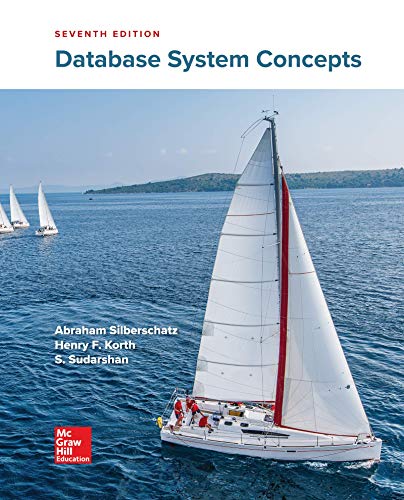
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
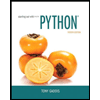
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
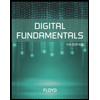
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
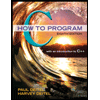
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
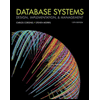
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
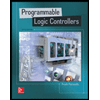
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education