This assignment will help you get accustomed to the C++ STL linked list class std::list. You may need to do a bit of research to complete a few of these activities. Please email your instructor if you have trouble and need assistance moving forward. You will need the following include files to complete the work below: • • Please complete the following activities: • Create a function called PrintList() that will print out all the elements of a list in a space-separated manner . Use this function to prove you have correctly completed your activities! • Create list a which is initialized to 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12 • Create list b which is initialized to 12, 11, 10, 9, 8, 7, 6, 5, 4, 3, 2, 1 . Reverse list b • Create list k which initializes with a copy of every element of a, using the copy constructor • Create list m which initializes with a copy of every element of b, using iterators of b • Merge list m into list k • Remove all duplicates of consecutive elements from k • Create list d which initializes with a copy of every element of a, using the copy constructor • Create list c which initializes with a copy of every element of b, using iterators of b • Create list e as a list of 7 elements all initialized to 9 • Create list of which uses a loop to set up the list into the squares of the first 30 numbers o 1, 4, 9, 25, 36, 49, 64, 81, 100, ... • Use std::stable_partition() and std::erase() to eliminate all odd values from linked list f • Create list h which uses a loop to initialize the list to the squares of the first 50 numbers o 1, 4, 9, 25, 36, 49, 64, 81, 100, .. • "Customize" and create your own custom STL std::sort() operation to sort first by even/odd status, and then sort values high to low . All even numbers move to the left of the list . All odd numbers move to the right of the list . Even grouping is sorted from high to low ■ Odd grouping is sorted from high to low • Find a way to use std::erase() to eliminate all odd values from linked list h
This assignment will help you get accustomed to the C++ STL linked list class std::list. You may need to do a bit of research to complete a few of these activities. Please email your instructor if you have trouble and need assistance moving forward. You will need the following include files to complete the work below: • • Please complete the following activities: • Create a function called PrintList() that will print out all the elements of a list in a space-separated manner . Use this function to prove you have correctly completed your activities! • Create list a which is initialized to 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12 • Create list b which is initialized to 12, 11, 10, 9, 8, 7, 6, 5, 4, 3, 2, 1 . Reverse list b • Create list k which initializes with a copy of every element of a, using the copy constructor • Create list m which initializes with a copy of every element of b, using iterators of b • Merge list m into list k • Remove all duplicates of consecutive elements from k • Create list d which initializes with a copy of every element of a, using the copy constructor • Create list c which initializes with a copy of every element of b, using iterators of b • Create list e as a list of 7 elements all initialized to 9 • Create list of which uses a loop to set up the list into the squares of the first 30 numbers o 1, 4, 9, 25, 36, 49, 64, 81, 100, ... • Use std::stable_partition() and std::erase() to eliminate all odd values from linked list f • Create list h which uses a loop to initialize the list to the squares of the first 50 numbers o 1, 4, 9, 25, 36, 49, 64, 81, 100, .. • "Customize" and create your own custom STL std::sort() operation to sort first by even/odd status, and then sort values high to low . All even numbers move to the left of the list . All odd numbers move to the right of the list . Even grouping is sorted from high to low ■ Odd grouping is sorted from high to low • Find a way to use std::erase() to eliminate all odd values from linked list h
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
In C++

Transcribed Image Text:This assignment will help you get accustomed to the C++ STL linked list class std::list.
You may need to do a bit of research to complete a few of these activities.
Please email your instructor if you have trouble and need assistance moving forward.
You will need the following include files to complete the work below:
<algorithm>
• <iostream>
• <list>
Please complete the following activities:
• Create a function called PrintList() that will print out all the elements of a list in a space-separated manner
• Use this function to prove you have correctly completed your activities!
• Create list a which is initialized to 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12
• Create list b which is initialized to 12, 11, 10, 9, 8, 7, 6, 5, 4, 3, 2, 1
• Reverse list b
• Create list k which initializes with a copy of every element of a, using the copy constructor
• Create list m which initializes with a copy of every element of b, using iterators of b
• Merge list m into list k
• Remove all duplicates of consecutive elements from k
• Create list d which initializes with a copy of every element of a, using the copy constructor
• Create list c which initializes with a copy of every element of b, using iterators of b
• Create list e as a list of 7 elements all initialized to 9
• Create list of which uses a loop to set up the list into the squares of the first 30 numbers
o 1, 4, 9, 25, 36, 49, 64, 81, 100, ...
• Use std::stable_partition() and std::erase() to eliminate all odd values from linked list f
• Create list h which uses a loop to initialize the list to the squares of the first 50 numbers
o 1, 4, 9, 25, 36, 49, 64, 81, 100, ...
• "Customize" and create your own custom STL std::sort() operation to sort first by even/odd status, and then sort values high to low
■ All even numbers move to the left of the list
. All odd numbers move to the right of the list
■ Even grouping is sorted from high to low
■ Odd grouping is sorted from high to low
• Find a way to use std::erase() to eliminate all odd values from linked list h
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
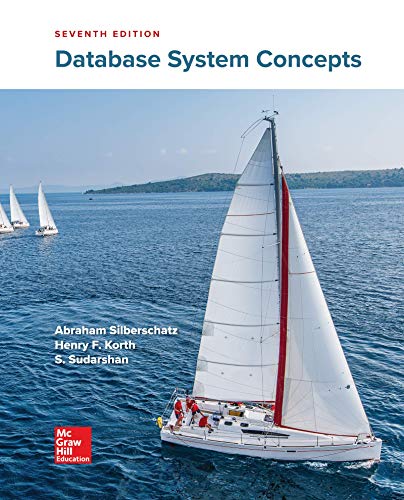
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
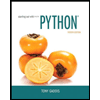
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
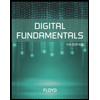
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
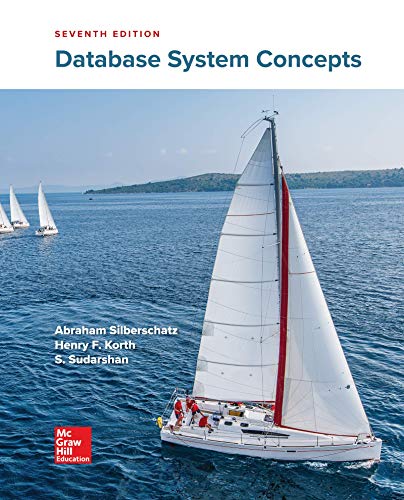
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
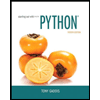
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
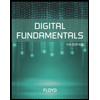
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
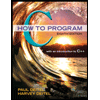
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
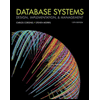
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
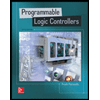
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education