OCaml Code: Please fix the pop command error that is attached. Don't reject the question because this is not a graded work. Below is the code along with the test cases. Make sure to show the correct code with the screenshot of the test cases being passed. interpreter.ml type stackValue = BOOL of bool | INT of int | ERROR | STRING of string | NAME of string | UNIT type command = ADD | SUB | MUL | DIV | PUSH of stackValue | POP | REM | NEG | TOSTRING | SWAP | PRINTLN | QUIT letinterpreter (input, output) = let ic = open_in input in let oc = open_out output in letrec loop_read acc = try let l = String.trim(input_line ic) in loop_read (l::acc) with | End_of_file -> List.rev acc in let strList = loop_read [] in let str2sv s = match s with | ":true:" -> BOOL true | ":false:" -> BOOL false | ":error:" -> ERROR | ":unit:" -> UNIT | _-> let rec try_parse_int str = try INT (int_of_string str) with | Failure _ -> try_parse_name str and try_parse_name str= NAME str in try_parse_int s in (* Helper function: file output function. It takes a bool value and write it to the output file. *) let file_write bool_val = Printf.fprintf oc "%s\n" bool_val in letstr2com s = match s with | "Add" -> ADD | "Sub" -> SUB | "Mul" -> MUL | "Div" -> DIV | "Swap" -> SWAP | "Neg" -> NEG | "Rem" -> REM | "ToString" -> TOSTRING | "Println" -> PRINTLN | "Quit" -> QUIT | _ -> PUSH (str2sv s) in let com2str c = match c with | "Add" -> ADD | "Sub" -> SUB | "Mul" -> MUL | "Div" -> DIV | "Swap" -> SWAP | "Neg" -> NEG | "Rem" -> REM | "ToString" -> TOSTRING | "Println" -> PRINTLN | "Quit" -> QUIT | _ -> PUSH (str2sv c) in let sv2str sv = match sv with | BOOL b -> string_of_bool b | INT i -> string_of_int i | ERROR -> ":error:" | STRING s -> s | NAME n -> n in let comList = List.map str2com strList in let stack = ref [] in (* Initialize an empty stack *) letprocessor cl stack = match (cl, stack) with | (ADD::restOfCommands, INT(a)::INT(b)::restOfStack) -> processor restOfCommands ( INT(a+b)::restOfStack) | (ADD::restOfCommands, s) -> processor restOfCommands (ERROR::stack) | (SUB::restOfCommands, INT(a)::INT(b)::restOfStack) -> processor restOfCommands ( INT(a-b)::restOfStack) | (SUB::restOfCommands, s) -> processor restOfCommands (ERROR::stack) | (MUL::restOfCommands, INT(a)::INT(b)::restOfStack) -> processor restOfCommands ( INT(a*b)::restOfStack) | (MUL::restOfCommands, s) -> processor restOfCommands (ERROR::stack) | (DIV::restOfCommands, INT(a)::INT(b)::restOfStack) -> processor restOfCommands ( INT(a/b)::restOfStack) | (DIV::restOfCommands, s) -> processor restOfCommands (ERROR::stack) | (REM::restOfCommands, INT(a)::INT(b)::restOfStack) -> processor restOfCommands ( INT(a%b)::restOfStack) | (REM::restOfCommands, s) -> processor restOfCommands (ERROR::stack) | (SWAP::restOfCommands, x::y::restOfStack) -> processor restOfCommands y::x::restOfStack | (SWAP::restOfCommands, s) -> processor restOfCommands (ERROR::stack) | (NEG::restOfCommands, INT(a)::restOfStack) -> processor restOfCommands ( INT(-a)::restOfStack) | (NEG::restOfCommands, s) -> processor restOfCommands (ERROR::stack) | (TOSTRING::restOfCommands, x::restOfStack) -> processor restOfCommands ( (sv2str x)::restOfStack) | (TOSTRING::restOfCommands, s) -> processor restOfCommands (ERROR::stack) | (PUSH sv::restOfCommands, _) -> processor restOfCommands (sv::stack) | (POP sv::restOfCommands, hd::restOfStack) -> processor restOfCommands restOfStack | (POP sv::restOfCommands, _) -> processor restOfCommands (ERROR::stack) | (PRINTLN::restOfCommands, STRING(a)::restOfStack) -> file_write (a);processor restOfCommands (ERROR::stack) | (PRINTLN::restOfCommands, []) -> processor restOfCommands (ERROR::stack) in processor comList [] Test cases Test cases: input1.txt: push 1 toString println quit
OCaml Code: Please fix the pop command error that is attached. Don't reject the question because this is not a graded work. Below is the code along with the test cases. Make sure to show the correct code with the screenshot of the test cases being passed. interpreter.ml type stackValue = BOOL of bool | INT of int | ERROR | STRING of string | NAME of string | UNIT type command = ADD | SUB | MUL | DIV | PUSH of stackValue | POP | REM | NEG | TOSTRING | SWAP | PRINTLN | QUIT letinterpreter (input, output) = let ic = open_in input in let oc = open_out output in letrec loop_read acc = try let l = String.trim(input_line ic) in loop_read (l::acc) with | End_of_file -> List.rev acc in let strList = loop_read [] in let str2sv s = match s with | ":true:" -> BOOL true | ":false:" -> BOOL false | ":error:" -> ERROR | ":unit:" -> UNIT | _-> let rec try_parse_int str = try INT (int_of_string str) with | Failure _ -> try_parse_name str and try_parse_name str= NAME str in try_parse_int s in (* Helper function: file output function. It takes a bool value and write it to the output file. *) let file_write bool_val = Printf.fprintf oc "%s\n" bool_val in letstr2com s = match s with | "Add" -> ADD | "Sub" -> SUB | "Mul" -> MUL | "Div" -> DIV | "Swap" -> SWAP | "Neg" -> NEG | "Rem" -> REM | "ToString" -> TOSTRING | "Println" -> PRINTLN | "Quit" -> QUIT | _ -> PUSH (str2sv s) in let com2str c = match c with | "Add" -> ADD | "Sub" -> SUB | "Mul" -> MUL | "Div" -> DIV | "Swap" -> SWAP | "Neg" -> NEG | "Rem" -> REM | "ToString" -> TOSTRING | "Println" -> PRINTLN | "Quit" -> QUIT | _ -> PUSH (str2sv c) in let sv2str sv = match sv with | BOOL b -> string_of_bool b | INT i -> string_of_int i | ERROR -> ":error:" | STRING s -> s | NAME n -> n in let comList = List.map str2com strList in let stack = ref [] in (* Initialize an empty stack *) letprocessor cl stack = match (cl, stack) with | (ADD::restOfCommands, INT(a)::INT(b)::restOfStack) -> processor restOfCommands ( INT(a+b)::restOfStack) | (ADD::restOfCommands, s) -> processor restOfCommands (ERROR::stack) | (SUB::restOfCommands, INT(a)::INT(b)::restOfStack) -> processor restOfCommands ( INT(a-b)::restOfStack) | (SUB::restOfCommands, s) -> processor restOfCommands (ERROR::stack) | (MUL::restOfCommands, INT(a)::INT(b)::restOfStack) -> processor restOfCommands ( INT(a*b)::restOfStack) | (MUL::restOfCommands, s) -> processor restOfCommands (ERROR::stack) | (DIV::restOfCommands, INT(a)::INT(b)::restOfStack) -> processor restOfCommands ( INT(a/b)::restOfStack) | (DIV::restOfCommands, s) -> processor restOfCommands (ERROR::stack) | (REM::restOfCommands, INT(a)::INT(b)::restOfStack) -> processor restOfCommands ( INT(a%b)::restOfStack) | (REM::restOfCommands, s) -> processor restOfCommands (ERROR::stack) | (SWAP::restOfCommands, x::y::restOfStack) -> processor restOfCommands y::x::restOfStack | (SWAP::restOfCommands, s) -> processor restOfCommands (ERROR::stack) | (NEG::restOfCommands, INT(a)::restOfStack) -> processor restOfCommands ( INT(-a)::restOfStack) | (NEG::restOfCommands, s) -> processor restOfCommands (ERROR::stack) | (TOSTRING::restOfCommands, x::restOfStack) -> processor restOfCommands ( (sv2str x)::restOfStack) | (TOSTRING::restOfCommands, s) -> processor restOfCommands (ERROR::stack) | (PUSH sv::restOfCommands, _) -> processor restOfCommands (sv::stack) | (POP sv::restOfCommands, hd::restOfStack) -> processor restOfCommands restOfStack | (POP sv::restOfCommands, _) -> processor restOfCommands (ERROR::stack) | (PRINTLN::restOfCommands, STRING(a)::restOfStack) -> file_write (a);processor restOfCommands (ERROR::stack) | (PRINTLN::restOfCommands, []) -> processor restOfCommands (ERROR::stack) in processor comList [] Test cases Test cases: input1.txt: push 1 toString println quit
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
OCaml Code: Please fix the pop command error that is attached. Don't reject the question because this is not a graded work. Below is the code along with the test cases. Make sure to show the correct code with the screenshot of the test cases being passed.
interpreter.ml
type stackValue = BOOL of bool | INT of int | ERROR | STRING of string | NAME of string | UNIT
type command = ADD | SUB | MUL | DIV | PUSH of stackValue | POP | REM | NEG | TOSTRING | SWAP | PRINTLN | QUIT
letinterpreter (input, output) =
let ic = open_in input
in
let oc = open_out output
in
letrec loop_read acc =
try
let l = String.trim(input_line ic) in loop_read (l::acc)
with
| End_of_file -> List.rev acc
in
let strList = loop_read []
in
let str2sv s =
match s with
| ":true:" -> BOOL true
| ":false:" -> BOOL false
| ":error:" -> ERROR
| ":unit:" -> UNIT
| _->
let rec try_parse_int str =
try
INT (int_of_string str)
with
| Failure _ -> try_parse_name str
and try_parse_name str=
NAME str
in
try_parse_int s
in
(* Helper function: file output function. It takes a bool value and
write it to the output file. *)
let file_write bool_val = Printf.fprintf oc "%s\n" bool_val in
letstr2com s =
match s with
| "Add" -> ADD
| "Sub" -> SUB
| "Mul" -> MUL
| "Div" -> DIV
| "Swap" -> SWAP
| "Neg" -> NEG
| "Rem" -> REM
| "ToString" -> TOSTRING
| "Println" -> PRINTLN
| "Quit" -> QUIT
| _ -> PUSH (str2sv s)
in
let com2str c =
match c with
| "Add" -> ADD
| "Sub" -> SUB
| "Mul" -> MUL
| "Div" -> DIV
| "Swap" -> SWAP
| "Neg" -> NEG
| "Rem" -> REM
| "ToString" -> TOSTRING
| "Println" -> PRINTLN
| "Quit" -> QUIT
| _ -> PUSH (str2sv c)
in
let sv2str sv =
match sv with
| BOOL b -> string_of_bool b
| INT i -> string_of_int i
| ERROR -> ":error:"
| STRING s -> s
| NAME n -> n
in
let comList = List.map str2com strList
in
let stack = ref [] in (* Initialize an empty stack *)
letprocessor cl stack =
match (cl, stack) with
| (ADD::restOfCommands, INT(a)::INT(b)::restOfStack) -> processor restOfCommands ( INT(a+b)::restOfStack)
| (ADD::restOfCommands, s) -> processor restOfCommands (ERROR::stack)
| (SUB::restOfCommands, INT(a)::INT(b)::restOfStack) -> processor restOfCommands ( INT(a-b)::restOfStack)
| (SUB::restOfCommands, s) -> processor restOfCommands (ERROR::stack)
| (MUL::restOfCommands, INT(a)::INT(b)::restOfStack) -> processor restOfCommands ( INT(a*b)::restOfStack)
| (MUL::restOfCommands, s) -> processor restOfCommands (ERROR::stack)
| (DIV::restOfCommands, INT(a)::INT(b)::restOfStack) -> processor restOfCommands ( INT(a/b)::restOfStack)
| (DIV::restOfCommands, s) -> processor restOfCommands (ERROR::stack)
| (REM::restOfCommands, INT(a)::INT(b)::restOfStack) -> processor restOfCommands ( INT(a%b)::restOfStack)
| (REM::restOfCommands, s) -> processor restOfCommands (ERROR::stack)
| (SWAP::restOfCommands, x::y::restOfStack) -> processor restOfCommands y::x::restOfStack
| (SWAP::restOfCommands, s) -> processor restOfCommands (ERROR::stack)
| (NEG::restOfCommands, INT(a)::restOfStack) -> processor restOfCommands ( INT(-a)::restOfStack)
| (NEG::restOfCommands, s) -> processor restOfCommands (ERROR::stack)
| (TOSTRING::restOfCommands, x::restOfStack) -> processor restOfCommands ( (sv2str x)::restOfStack)
| (TOSTRING::restOfCommands, s) -> processor restOfCommands (ERROR::stack)
| (PUSH sv::restOfCommands, _) -> processor restOfCommands (sv::stack)
| (POP sv::restOfCommands, hd::restOfStack) -> processor restOfCommands restOfStack
| (POP sv::restOfCommands, _) -> processor restOfCommands (ERROR::stack)
| (PRINTLN::restOfCommands, STRING(a)::restOfStack) -> file_write (a);processor restOfCommands (ERROR::stack)
| (PRINTLN::restOfCommands, []) -> processor restOfCommands (ERROR::stack)
in processor comList []
Test cases
Test cases:
input1.txt:
push 1
toString
println
quit
output1.txt:
1
![---
### Understanding OCaml Pattern Matching and Constructor Errors
When working with OCaml, pattern matching is a fundamental concept that helps in deconstructing values and data types. However, developers often encounter warnings and errors related to pattern matching and constructors. Let's examine and understand a typical scenario.
#### Warning: Non-Exhaustive Pattern Matching
**Message:**
```
File "interpreter.ml", line 77, characters 2-142:
Warning 8: this pattern-matching is not exhaustive.
Here is an example of a case that is not matched:
UNIT
```
**Explanation:**
This warning indicates that the pattern matching in your code does not cover all possible cases. Specifically, the case `UNIT` is an example of a value that is not accounted for in your pattern matching blocks. In OCaml, ensuring exhaustive pattern matches is critical to avoid runtime errors.
**Solution:**
To resolve this warning, you should review your pattern-matching code at the specified location (line 77, characters 2-142) and ensure all possible cases are handled. Adding a wildcard pattern (`_`) can help match all remaining cases, but explicit patterns for each case are preferred for clarity and safety.
#### Error: Constructor Argument Mismatch
**Message:**
```
File "interpreter.ml", line 118, characters 5-11:
Error: The constructor POP expects 0 argument(s),
but is applied here to 1 argument(s)
```
**Explanation:**
This error occurs when the constructor `POP` is used incorrectly in your code. According to the type definition, `POP` does not take any arguments. However, your code attempts to pass one argument to it.
**Solution:**
To fix this error, locate the code at line 118, characters 5-11, and correct the application of `POP`. Ensure that `POP` is used without any arguments. For example:
```ocaml
match x with
| POP -> ...
```
instead of:
```ocaml
match x with
| POP(arg) -> ...
```
#### Makefile Error
**Message:**
```
Makefile:3: recipe for target 'all' failed
make: *** [all] Error 2
```
**Explanation:**
This error indicates that the `make` utility encountered an error while executing the `all` target defined in your `Makefile`. The specific error stems from the issues in the](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe7ddc10c-4670-40fd-b02c-6a60c5fcc2f2%2F5b117c40-4686-4ef1-b2f9-ab4803328289%2F4r69mh9_processed.png&w=3840&q=75)
Transcribed Image Text:---
### Understanding OCaml Pattern Matching and Constructor Errors
When working with OCaml, pattern matching is a fundamental concept that helps in deconstructing values and data types. However, developers often encounter warnings and errors related to pattern matching and constructors. Let's examine and understand a typical scenario.
#### Warning: Non-Exhaustive Pattern Matching
**Message:**
```
File "interpreter.ml", line 77, characters 2-142:
Warning 8: this pattern-matching is not exhaustive.
Here is an example of a case that is not matched:
UNIT
```
**Explanation:**
This warning indicates that the pattern matching in your code does not cover all possible cases. Specifically, the case `UNIT` is an example of a value that is not accounted for in your pattern matching blocks. In OCaml, ensuring exhaustive pattern matches is critical to avoid runtime errors.
**Solution:**
To resolve this warning, you should review your pattern-matching code at the specified location (line 77, characters 2-142) and ensure all possible cases are handled. Adding a wildcard pattern (`_`) can help match all remaining cases, but explicit patterns for each case are preferred for clarity and safety.
#### Error: Constructor Argument Mismatch
**Message:**
```
File "interpreter.ml", line 118, characters 5-11:
Error: The constructor POP expects 0 argument(s),
but is applied here to 1 argument(s)
```
**Explanation:**
This error occurs when the constructor `POP` is used incorrectly in your code. According to the type definition, `POP` does not take any arguments. However, your code attempts to pass one argument to it.
**Solution:**
To fix this error, locate the code at line 118, characters 5-11, and correct the application of `POP`. Ensure that `POP` is used without any arguments. For example:
```ocaml
match x with
| POP -> ...
```
instead of:
```ocaml
match x with
| POP(arg) -> ...
```
#### Makefile Error
**Message:**
```
Makefile:3: recipe for target 'all' failed
make: *** [all] Error 2
```
**Explanation:**
This error indicates that the `make` utility encountered an error while executing the `all` target defined in your `Makefile`. The specific error stems from the issues in the
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
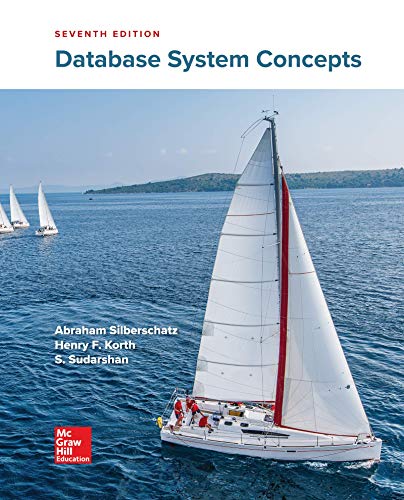
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
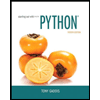
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
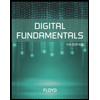
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
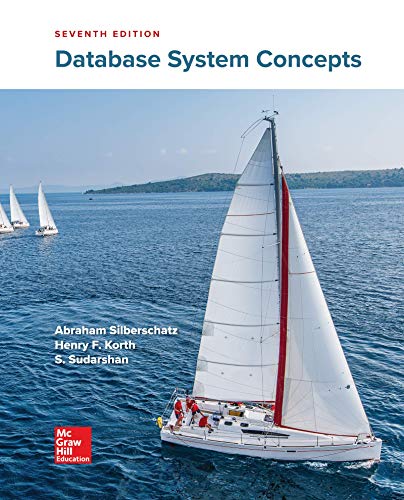
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
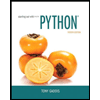
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
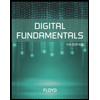
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
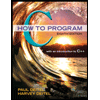
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
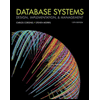
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
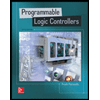
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education