include #include // Function to display the game board void displayBoard(char board[3][3]) { printf("\n"); printf(" %c | %c | %c \n", board[0][0], board[0][1], board[0][2]); printf(" ---|---|---\n"); printf(" %c | %c | %c \n", board[1][0], board[1][1], board[1][2]); printf(" ---|---|---\n"); printf(" %c | %c | %c \n"
GENERATE THE CODE BELOW FROM THE UNIX ENVIRONMENT
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
// Function to display the game board
void displayBoard(char board[3][3]) {
printf("\n");
printf(" %c | %c | %c \n", board[0][0], board[0][1], board[0][2]);
printf(" ---|---|---\n");
printf(" %c | %c | %c \n", board[1][0], board[1][1], board[1][2]);
printf(" ---|---|---\n");
printf(" %c | %c | %c \n", board[2][0], board[2][1], board[2][2]);
printf("\n");
}
// Function to check if the game is over (i.e., if someone has won or if there are no more moves)
int isGameOver(char board[3][3]) {
// Check rows for a win
for (int i = 0; i < 3; i++) {
if (board[i][0] != ' ' && board[i][0] == board[i][1] && board[i][1] == board[i][2]) {
return 1;
}
}
// Check columns for a win
for (int i = 0; i < 3; i++) {
if (board[0][i] != ' ' && board[0][i] == board[1][i] && board[1][i] == board[2][i]) {
return 1;
}
}
// Check diagonals for a win
if (board[0][0] != ' ' && board[0][0] == board[1][1] && board[1][1] == board[2][2]) {
return 1;
}
if (board[0][2] != ' ' && board[0][2] == board[1][1] && board[1][1] == board[2][0]) {
return 1;
}
// Check if there are any more moves
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
if (board[i][j] == ' ') {
return 0;
}
}
}
// If we get here, the game is over with no winner
return 1;
}
// Function to get the user's move
void getUserMove(char board[3][3], char player) {
int row, col;
do {
printf("Enter row (1-3) for %c: ", player);
scanf("%d", &row);
printf("Enter column (1-3) for %c: ", player);
scanf("%d", &col);
row--; // adjust to 0-indexed array
col--; // adjust to 0-indexed array
if (row < 0 || row > 2 || col < 0 || col > 2) {
printf("Invalid input! Please enter row and column numbers between 1 and 3.\n");
} else if (board[row][col] != ' ') {
printf("That spot is already taken! Please choose a different spot.\n");
}
} while (row < 0 || row > 2 || col < 0 || col > 2 || board[row][col] != ' ');
board[row][col] = player;
}
int main() {
// Set up random number generator
srand(time(NULL));
// Get player name
char playerName[50];
printf("Enter your name: ");
scanf("%s", playerName);
// Initialize game board
char board[3][3] = {
{' ', ' ', ' '},
{' ', ' ', ' '},
{' ', ' ', ' '}
};
// Print welcome message and empty board
printf("Welcome, %s! Let's play Tic Tac Toe.\n", playerName);
displayBoard(board);
// Game loop
char currentPlayer = 'X';
while (!isGameOver(board)) {
if (currentPlayer == 'X') {
getUserMove(board, currentPlayer);
} else {
// Computer's turn
int row, col;
do {
row = rand() % 3;
col = rand() % 3;
} while (board[row][col] != ' ');
board[row][col] = currentPlayer;
printf("Computer placed an %c at row %d, column %d.\n", currentPlayer, row + 1, col + 1);
}
// Print updated board
displayBoard(board);
// Switch players
if (currentPlayer == 'X') {
currentPlayer = 'O';
} else {
currentPlayer = 'X';
}
}
// Game over
if (isGameOver(board)) {
if (currentPlayer == 'X') {
printf("Computer wins!\n");
} else {
printf("%s wins!\n", playerName);
}
} else {
printf("It's a tie!\n");
}
return 0;
}

Step by step
Solved in 3 steps with 3 images

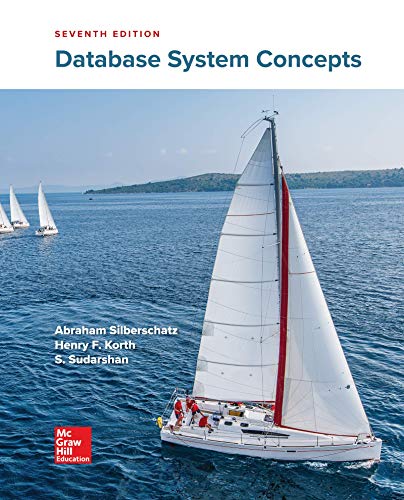
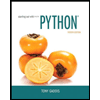
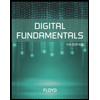
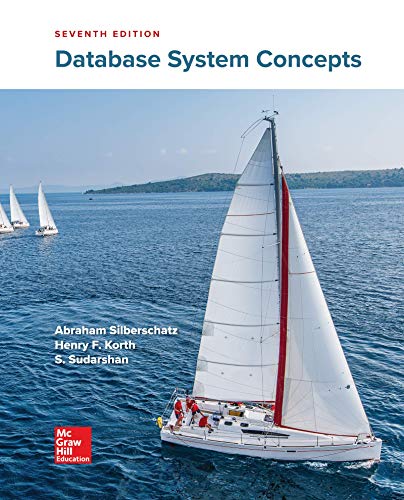
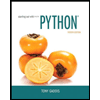
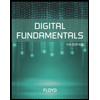
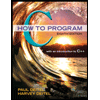
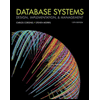
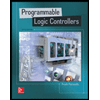