Please complete the following guidelines and hints. Using C language. Please use this template:
Please complete the following guidelines and hints. Using C language. Please use this template:
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Please complete the following guidelines and hints. Using C language.
Please use this template:
#include <stdio.h>
#define MAX 100
struct cg { // structure to hold x and y coordinates and mass
float x, y, mass;
}masses[MAX];
int readin(void)
{
/* Write this function to read in the data
into the array masses
note that this function should return the number of
masses read in from the file */
}
void computecg(int n_masses)
{
/* Write this function to compute the C of G
and print the result */
}
int main(void)
{
int number;
if((number = readin()) > 0)
computecg(number);
return 0;
}
Testing your work
Typical Input from keyboard:
4
0 0 1
0 1 1
1 0 1
1 1 1
Typical Output to screen:
CoG coordinates are: x = 0.50 y = 0.50
![1. Assignment: Calculate Centre of Gravity
We are concerned with finding the location of the combined centre of gravity of a
arbitrary number of weights. Each weight has a x coordinate, a y coordinate and a
mass associated with it. The data for a set of weights is provided by the user of a
program using functions to calculate the centre of gravity for these weights
combined. The program should ask the user to provide the number of weights and
subsequently to enter separately (one by one) the information for each weight in the
form
"x_coordinate y_coordinate mass" in one line.
You are required to write two functions to complete a program which will read the
information (data) from the standard input (keyboard), calculate and display at the
standard output (screen/display) the resultant centre of gravity location.
1) In the first function you should use formatted input (hint: scanf) to read the data.
You must use the following structure for the x and y coordinates and mass:
struct cg {
float x, y, mass;
};
and an array:
struct cg masses[MAX ];
to hold the data.
2) In the second function:
Compute:
sum_of_xmass = sum of the (masses[i].x * masses[i].mass)
sum_of_ymass = sum of the (masses[i].y * masses[i].mass)
sum_of_mass = sum of the (masses[i].mass)
for all i (i.e. for all the masses provided).
And calculate and print the centre of gravity, found from
cg_x = sum_of_xmass / sum_of_mass
cg_y = sum_of_ymass / sum_of_mass.
Template
Use the following template for your program:
#include <stdio.h>
#define MAX 100](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F3dce11ee-c8b0-4dfc-9b83-d8620fb2ae4c%2Fdd76b942-0e2b-4c14-aec5-dc50a97e3478%2Fbe4w4g9_processed.png&w=3840&q=75)
Transcribed Image Text:1. Assignment: Calculate Centre of Gravity
We are concerned with finding the location of the combined centre of gravity of a
arbitrary number of weights. Each weight has a x coordinate, a y coordinate and a
mass associated with it. The data for a set of weights is provided by the user of a
program using functions to calculate the centre of gravity for these weights
combined. The program should ask the user to provide the number of weights and
subsequently to enter separately (one by one) the information for each weight in the
form
"x_coordinate y_coordinate mass" in one line.
You are required to write two functions to complete a program which will read the
information (data) from the standard input (keyboard), calculate and display at the
standard output (screen/display) the resultant centre of gravity location.
1) In the first function you should use formatted input (hint: scanf) to read the data.
You must use the following structure for the x and y coordinates and mass:
struct cg {
float x, y, mass;
};
and an array:
struct cg masses[MAX ];
to hold the data.
2) In the second function:
Compute:
sum_of_xmass = sum of the (masses[i].x * masses[i].mass)
sum_of_ymass = sum of the (masses[i].y * masses[i].mass)
sum_of_mass = sum of the (masses[i].mass)
for all i (i.e. for all the masses provided).
And calculate and print the centre of gravity, found from
cg_x = sum_of_xmass / sum_of_mass
cg_y = sum_of_ymass / sum_of_mass.
Template
Use the following template for your program:
#include <stdio.h>
#define MAX 100
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
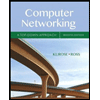
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
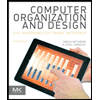
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
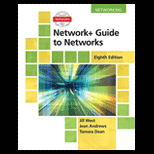
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
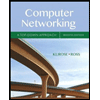
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
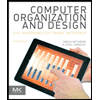
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
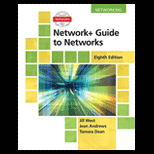
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
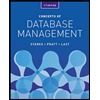
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
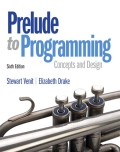
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
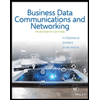
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY